How to find the size of an array (from a pointer pointing to the first element array)?
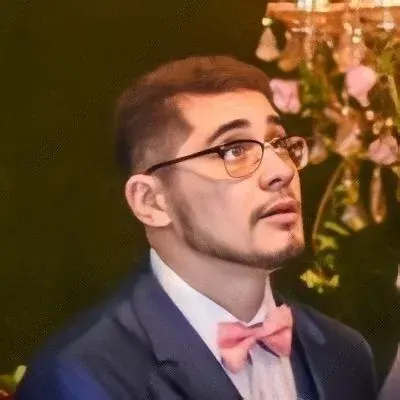
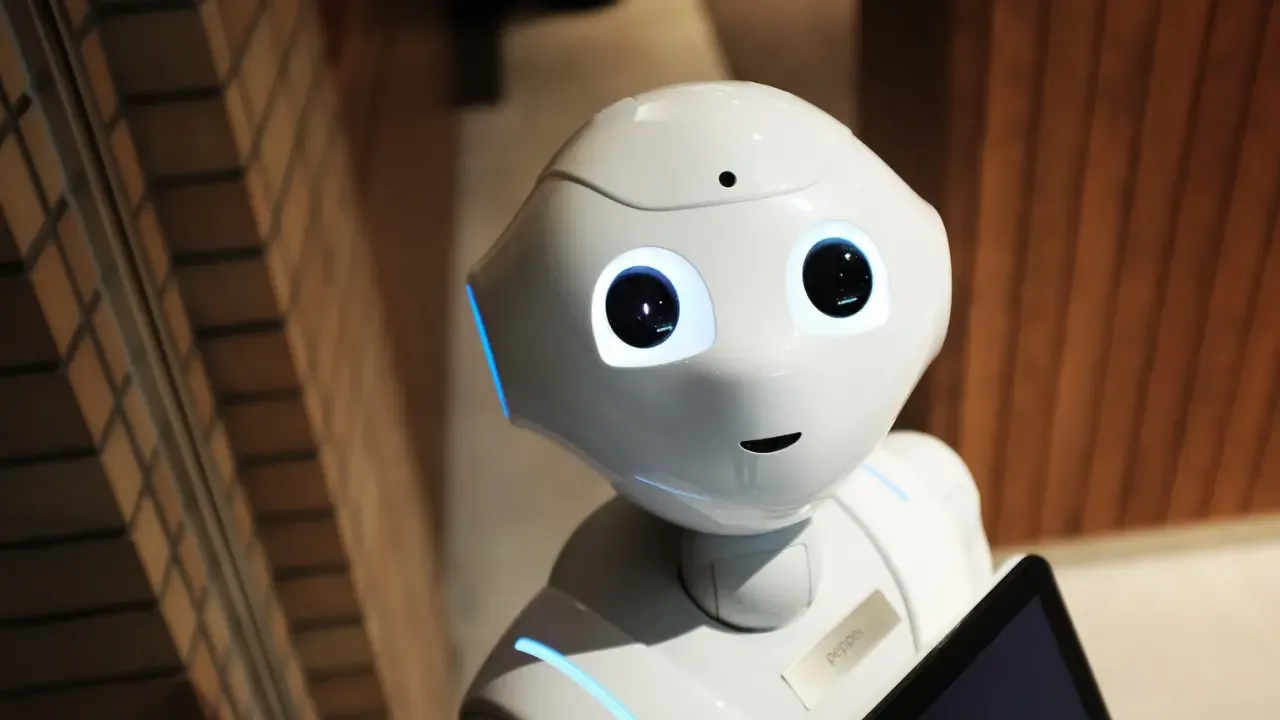
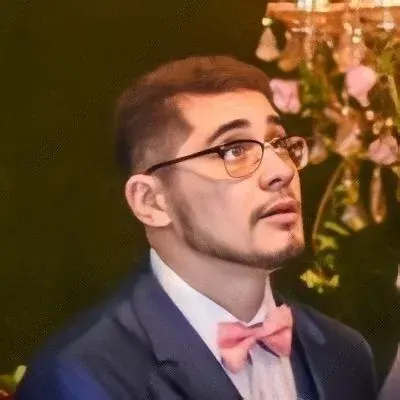
How to Find the Size of an Array 📏 (from a Pointer Pointing to the First Element of the Array)
So, you've come across this interesting question: How can you find the size of an array from a pointer pointing to the first element? 🤔
The Context: 📝
Let's take a look at this code snippet to understand the context:
int main()
{
int days[] = {1,2,3,4,5};
int *ptr = days;
printf("%u\n", sizeof(days));
printf("%u\n", sizeof(ptr));
return 0;
}
In this code, we have an array called days
which holds 5 integers. We also have a pointer called ptr
that points to the first element of the days
array.
The two printf
statements are used to display the size of the days
array and the size of the ptr
pointer respectively.
The Problem: ❗
The question arises: Is there a way to find out the size of the array that ptr
is pointing to, instead of just giving its size (which is four bytes on a 32-bit system)? 🤷♂️
The Solution: ✅
Unfortunately, there is no way to directly find the size of an array from a pointer pointing to its first element. 😕
However, we can use a workaround by calculating the size indirectly. One way to achieve this is by dividing the size of the pointer by the size of the data type the pointer points to.
For instance, if ptr
is a pointer to an int
, we can calculate the size of the array days
as follows:
size_t arraySize = sizeof(days) / sizeof(int);
Putting It All Together: 💡
Let's modify our original code snippet to include the calculation for the size of the array:
#include <stdio.h>
int main()
{
int days[] = {1,2,3,4,5};
int *ptr = days;
size_t arraySize = sizeof(days) / sizeof(int); // Calculate the size of the array
printf("%u\n", arraySize); // Display the size of the array
printf("%u\n", sizeof(ptr)); // Display the size of the pointer
return 0;
}
When we run this modified code, it calculates and displays the size of the days
array using the arraySize
variable.
Conclusion: 🎉
Although we cannot directly find the size of an array from a pointer pointing to its first element, we can use the size of the pointer and the size of the data type to calculate it indirectly.
Now you have the knowledge to overcome this common challenge when working with arrays and pointers in C. Happy coding! 💻
Your Turn: ✏️
Have you ever encountered a different way to find the size of an array from a pointer? Share your thoughts and experiences in the comments below. Let's learn together! 🌟