How to disable GCC warnings for a few lines of code
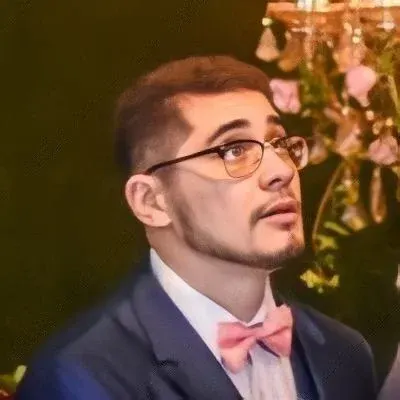

How to Disable GCC Warnings for a Few Lines of Code ๐ซโ ๏ธ
Have you ever encountered annoying warning messages when compiling your code with GCC? ๐ค These warnings can clutter your build output and make it difficult to spot legitimate errors. Fortunately, GCC provides a handy feature that allows you to selectively disable warnings for specific lines or blocks of code! ๐ In this blog post, we'll explore how to override compiler flags for individual lines or code sections in GCC. Let's dive in! ๐ช
The Problem ๐คฆโโ๏ธ
GCC is known for its strict adherence to the C and C++ standards, which means it's always on the lookout for potential issues in your code. While these warnings are valuable for catching bugs and improving code quality, there may be situations where you need to temporarily suppress certain warnings. This is especially common when working with legacy code or when dealing with third-party libraries that trigger unnecessary warnings. So, how can we silence these warnings without resorting to global suppression? ๐ค
The Solution ๐
GCC provides a feature called "diagnostic pragmas" that allows you to override specific compiler flags for a limited scope. These pragmas give you fine-grained control over warning suppression, making it possible to disable warnings for individual lines or blocks of code. Here's how you can do it:
Disable a warning for a single line:
#pragma GCC diagnostic push #pragma GCC diagnostic ignored "-W<your-warning>" // Code that triggers the warning #pragma GCC diagnostic pop
Replace
<your-warning>
with the specific warning you want to disable. For example, if you want to suppress the "unused variable" warning (-Wunused-variable), you would use-Wunused-variable
in the pragma. Enclose the code that triggers the warning between the#pragma
directives to apply the suppression only to that line.Disable warnings for a block of code:
#pragma GCC diagnostic push #pragma GCC diagnostic ignored "-W<your-warning-1>" #pragma GCC diagnostic ignored "-W<your-warning-2>" // Code that triggers the warnings #pragma GCC diagnostic pop
Similarly, you can disable multiple warnings for a code block by adding multiple
#pragma GCC diagnostic ignored
directives. Make sure to enclose the relevant code between thepush
andpop
directives to restrict the suppression to that block.
That's it! With these few lines of code, you can selectively silence GCC warnings and keep your build output clean and meaningful. ๐งน
When to Use It โ
While disabling warnings should generally be avoided unless absolutely necessary, there are valid use cases for selectively suppressing GCC warnings. Here are a few scenarios where this technique can come in handy:
Third-party libraries: If you're using a third-party library that triggers warnings in your code, you can wrap the library calls with the
diagnostic push
andpop
directives to suppress those specific warnings. This avoids polluting your build output with warnings you have little control over.Legacy code: When working with legacy code, you may encounter warnings that are too difficult or time-consuming to fix. By temporarily disabling these warnings, you can focus on more pressing issues without cluttering your development environment.
Conclusion ๐
GCC's diagnostic pragmas offer a powerful mechanism to selectively disable warnings for specific lines or blocks of code. While disabling warnings should be approached with caution, it can be a useful tool in certain situations. Now that you know how to use these pragmas, you can easily keep your build output clean and tidy, making it easier to spot real issues. Happy coding! ๐ป๐
Do you have any other tips for managing GCC warnings? Share your insights in the comments below! Let's help each other improve our code and crush those bugs together! ๐๐ช
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
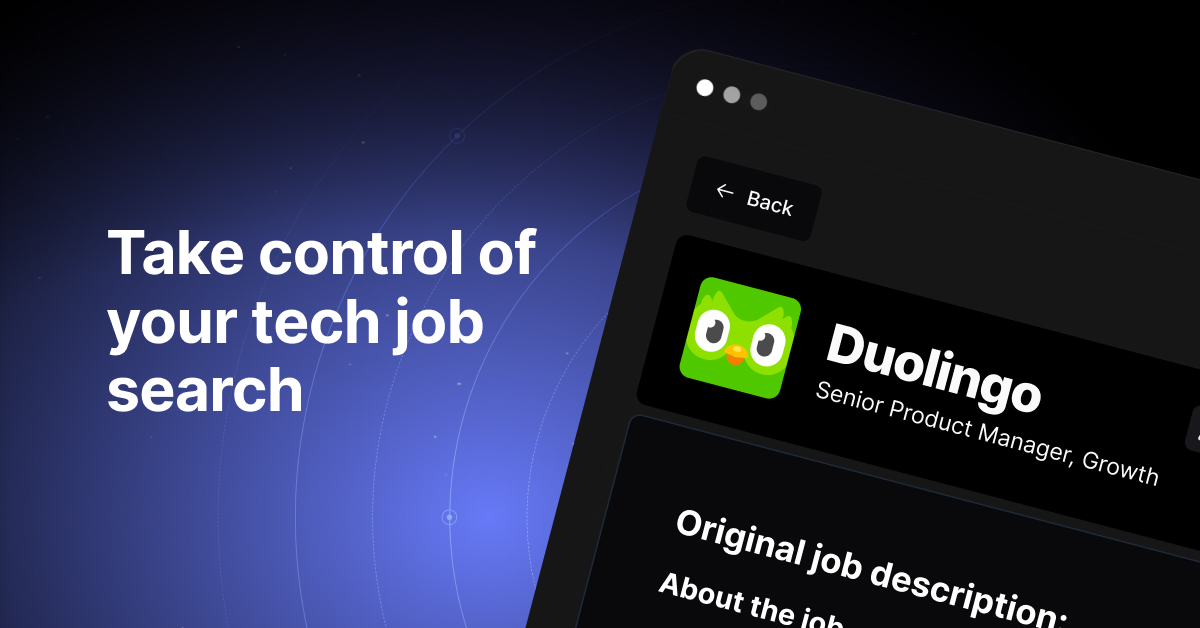