How to define an enumerated type (enum) in C?
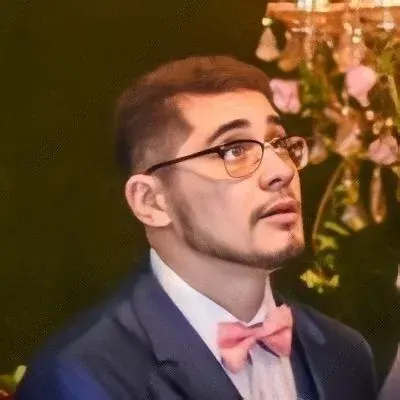
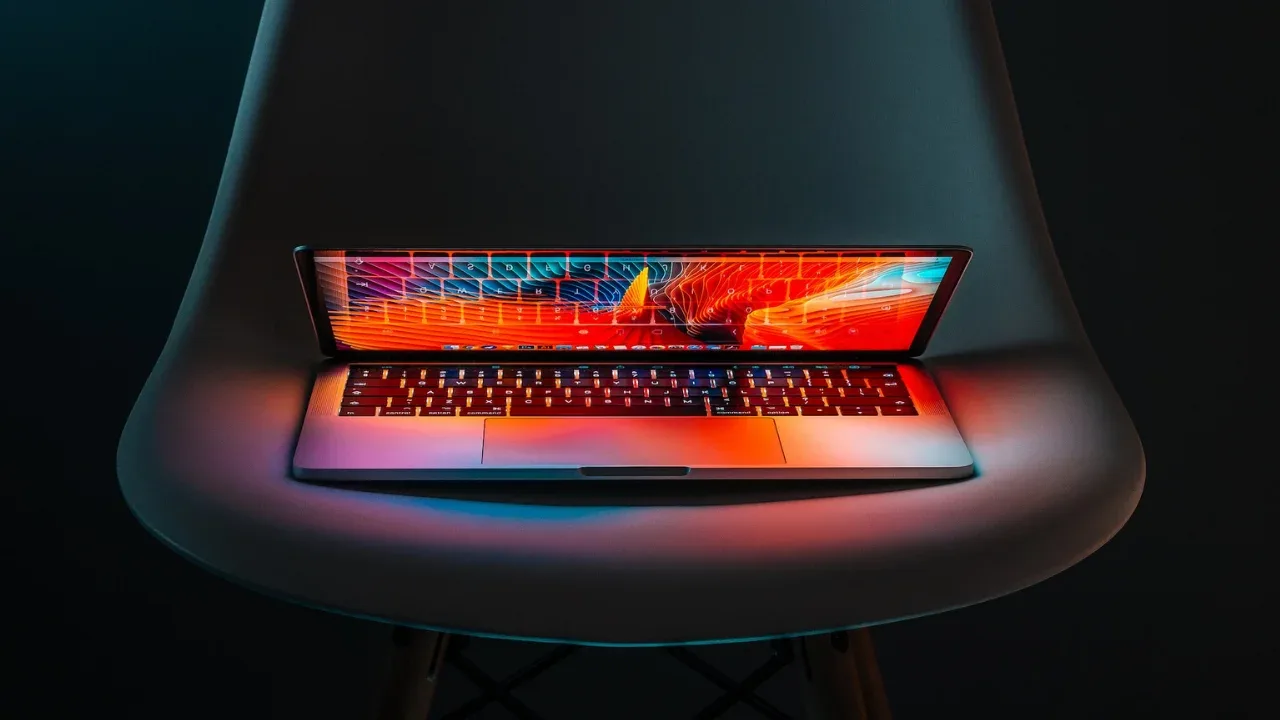
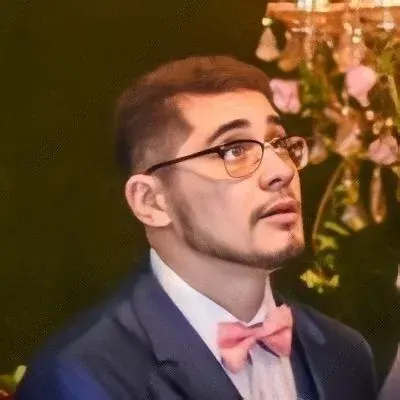
How to Define Enumerated Types (enum) in C 🤔📝
So, you want to use enums in your C code? Great choice! Enums can make your code more readable and provide a convenient way to define a set of related values. However, it's essential to use the correct syntax to avoid any errors.
Understanding the Error 🚫
The error message you're encountering is quite common when defining enums in C. Let's take a closer look at your code:
enum {RANDOM, IMMEDIATE, SEARCH} strategy;
strategy = IMMEDIATE;
The error suggests conflicting types for the strategy
variable. But wait a minute! We only declared it once, right? Well, here's the catch. The issue arises because you're redeclaring the strategy
variable. Let's see how we can fix this!
Correct Syntax for Enum Definitions 📝
To define an enum variable, you should follow this syntax:
enum enum_name {value1, value2, value3};
Here's an example using your code:
enum strategy {RANDOM, IMMEDIATE, SEARCH} strategy_var;
strategy_var = IMMEDIATE;
In this code snippet, we're giving the enum type a name (strategy
) to distinguish it from the variable of the same name (strategy_var
). Now, you won't run into any conflicting type errors!
Enum Usage Examples ✨
Let's dive deeper into how enums can make your code more expressive. Here are a couple of ways you can utilize enums in practical scenarios:
Example 1: Days of the Week
enum days {SUNDAY, MONDAY, TUESDAY, WEDNESDAY, THURSDAY, FRIDAY, SATURDAY};
enum days today;
today = MONDAY;
The above code snippet defines an enum for the days of the week and assigns the value MONDAY
to the variable today
. Now, your code can easily identify which day it is!
Example 2: Error Codes
enum error_codes {SUCCESS, FILE_NOT_FOUND, INVALID_INPUT};
enum error_codes error;
error = INVALID_INPUT;
In this example, we're defining an enum for error codes. By assigning a value to the error
variable, you can easily identify and handle different error conditions in your code.
📣 Take Action! Participate and Engage 🙌
Now that you've learned how to define enums correctly in C, put this knowledge into practice! Update your code, remove any conflicting type errors, and embrace the power of enums.
Have you encountered any other C programming issues or questions? Let us know in the comments below! Our community of passionate programmers is here to help and discuss. 🔥💬
Make sure to share this post with your fellow developers who might benefit from learning the proper enum syntax in C!
Happy coding! 🚀✨