How to correctly use the extern keyword in C
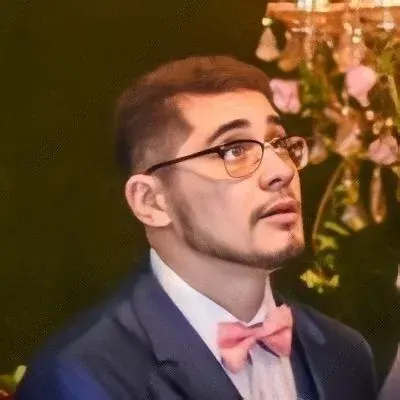
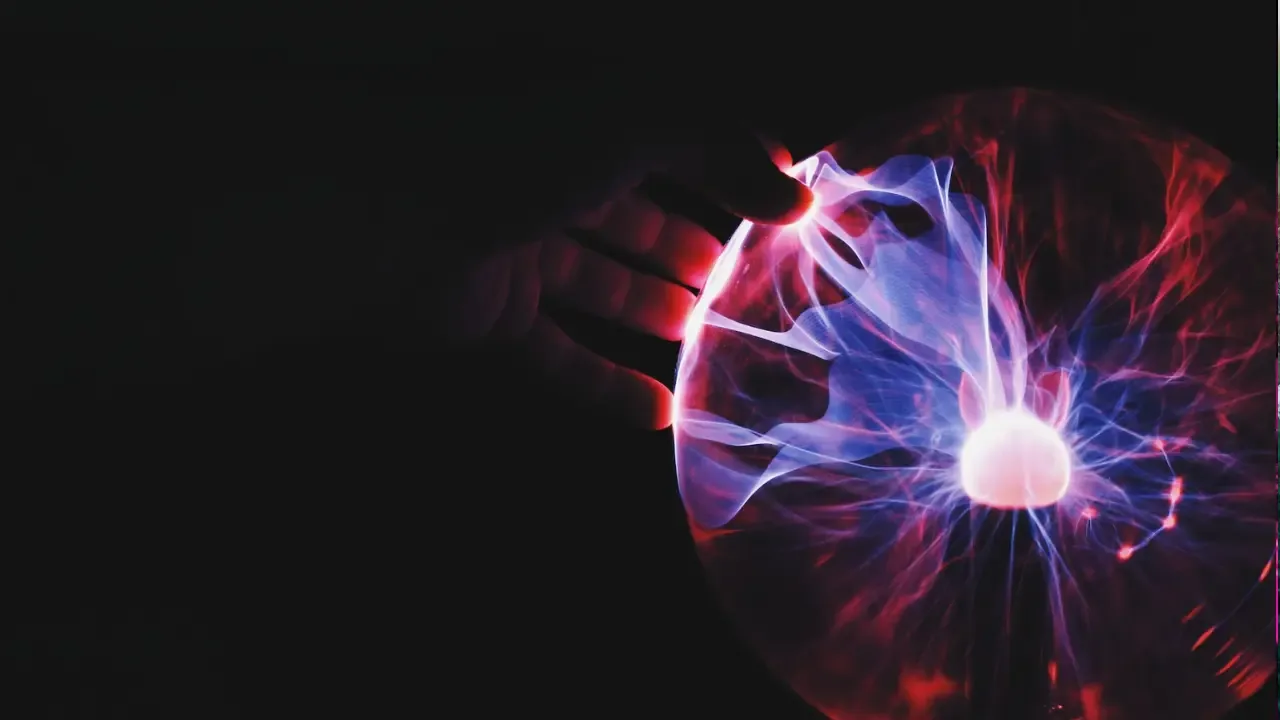
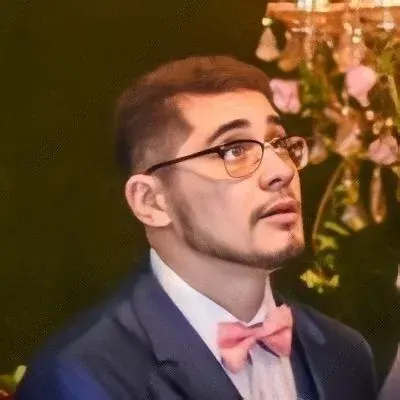
How to correctly use the extern
keyword in C 🌐
Are you confused about the extern
keyword in C? 😕 You're not alone! Many developers find it tricky to understand when and how to use it. But fear not! In this blog post, we'll demystify the extern
keyword and give you practical examples to help you understand its proper usage. Let's dive in! 💪
Understanding the purpose of extern
🔍
The extern
keyword is used to declare a variable or function that is defined in another source file or library. It is a way to tell the compiler that the declaration being made is not local but is located elsewhere. This is particularly useful when you want to access variables or functions that have global scope but are defined in a different file.
Common issues and their solutions 🛠️
Issue #1: Difficulty accessing global variables
Let's say you have a global variable defined in one source file but need to access it in another. Without the extern
keyword, the compiler will throw an "undefined reference" error. To fix this, you can use the extern
keyword in the source file where you want to access the variable:
// File1.c
int globalVariable = 10;
// File2.c
extern int globalVariable;
Now, you can use globalVariable
in File2.c without any issues.
Issue #2: Using functions across multiple source files
If you have a function defined in a different source file and want to use it in your current file, you'll face a similar issue. The extern
keyword comes to the rescue again! Use it to declare the function in your current file before using it:
// File1.c
void externalFunction(); // Function definition
// File2.c
extern void externalFunction(); // Function declaration
int main() {
externalFunction(); // Function call
}
By declaring the function as extern
, you can use it without any problems in the current file.
The extern
keyword in header files 📝
When it comes to header files, things get a bit different. In most cases, header files already provide the necessary declarations for functions and variables. Therefore, using extern
in header files is generally unnecessary.
However, if you have a global variable declared in a header file, and want to access it in multiple source files, you can declare it as extern
in the header file and define it in one of the source files:
// Header.h
extern int sharedVariable;
// File1.c
#include "Header.h"
int sharedVariable = 42;
// File2.c
#include "Header.h"
void someFunction() {
printf("Value of sharedVariable: %d", sharedVariable);
}
In this case, the extern
keyword in the header file signals that the definition of sharedVariable
is located elsewhere.
Time to master the extern
keyword! 💡
Congratulations! You now have a solid understanding of how and when to use the extern
keyword in C. Keep in mind the following key points:
Use
extern
when accessing global variables or functions defined in other source files.Avoid using
extern
in header files unless you specifically need to declare a global variable that will be defined in a separate source file.
If you still have any doubts or questions, feel free to leave a comment below. Happy coding! 💻✨