How to convert a string to integer in C?
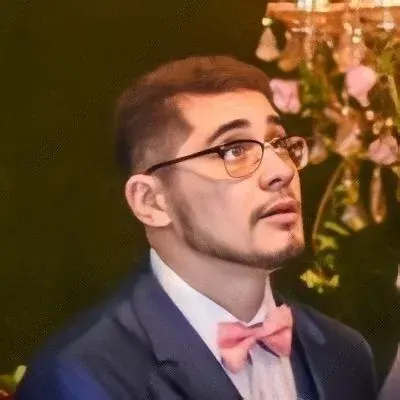
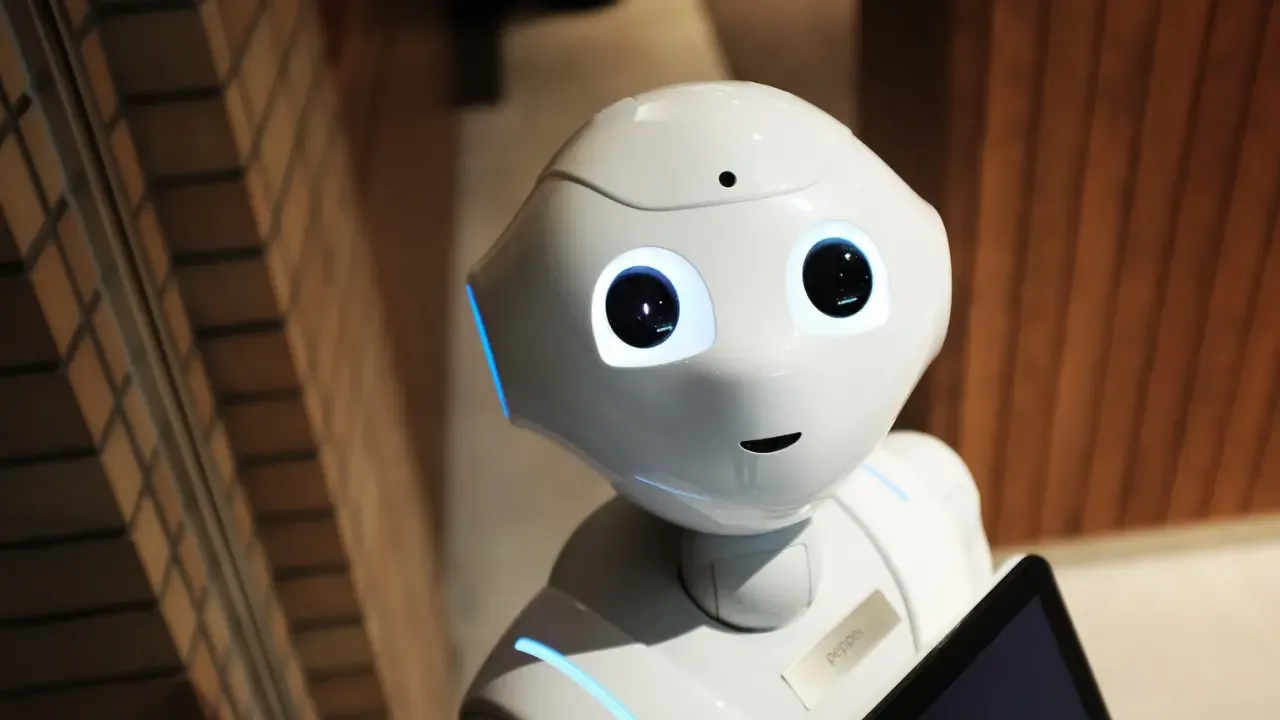
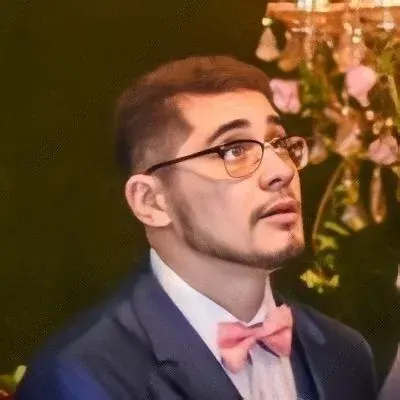
How to Convert a String to Integer in C? 🤔💡
Are you tired of using the same old method to convert a string to an integer in C? Looking for a better or alternative way? You've come to the right place! In this blog post, we'll explore different methods and provide easy solutions to convert a string to an integer in C. Let's dive right in and solve this problem together.
The Common Approach and Its Limitations 🔄🔒
The most common approach to convert a string to an integer in C is by using the atoi()
function. It takes a string as input and returns the corresponding integer value. It works great in most cases, just like in the code snippet provided:
char s[] = "45";
int num = atoi(s);
However, despite its simplicity, the atoi()
function has some limitations you need to be aware of. It assumes that the input string is a valid representation of an integer and doesn't handle errors gracefully. For example, if the string contains non-numeric characters or exceeds the maximum or minimum values for an integer, it may produce unexpected results or even crash your program. 😱
A Safer and More Versatile Solution ✨🛡️
To overcome the limitations of atoi()
, we recommend using the strtol()
function instead. It offers better error handling and flexibility. Here's how you can use it:
#include <stdlib.h>
char s[] = "45";
char* endptr;
long num = strtol(s, &endptr, 10);
In this example, strtol()
converts the string s
to a long integer (long
), which can accommodate larger values than an int
. The third argument, 10
, specifies the base of the number system (decimal in this case). The function also takes a second argument, endptr
, which points to the first character that couldn't be parsed as part of the integer. This feature provides built-in error checking, allowing you to handle invalid input more gracefully. 🧐
To determine if the conversion was successful, you can check the contents of endptr
. If it points to the string's null terminator (\0
), the entire string was converted successfully. Otherwise, there was an error in the conversion process.
But what if you want to stick with using an int
instead of a long
? No worries! You can simply cast the long
variable to an int
once the conversion is complete. 😉
#include <stdlib.h>
char s[] = "45";
char* endptr;
long num = strtol(s, &endptr, 10);
int convertedNum = (int)num;
Going Beyond: Handling Special Cases 🚀🌟
What about scenarios where you need to convert a string with leading or trailing non-numeric characters? The strtol()
function can handle that too! Take a look at this example: ✨
#include <stdlib.h>
#include <ctype.h>
char s[] = "ABC123XYZ";
char* endptr;
long num = strtol(s, &endptr, 10);
if (endptr == s) {
// No digits were found
// Handle the error gracefully
}
else if (*endptr != '\0' && !isspace((unsigned char)*endptr)) {
// Conversion stopped at an invalid character
// Handle the error gracefully
}
else {
// Conversion was successful
// Use the converted number
int convertedNum = (int)num;
// Proceed with your code
}
In this example, we added additional checks to ensure we handle cases where the string contains leading non-numeric characters or invalid characters after the numeric portion. This provides even more robust error handling for your code. 😎
Conclusion and Your Next Steps 🎉💪
Converting a string to an integer in C is a common task, and it's important to choose the right method that suits your needs. While the atoi()
function is commonly used, it has its limitations. By using the strtol()
function, you can overcome these limitations and enjoy better error handling. Don't forget to handle special cases like leading or trailing non-numeric characters, enhancing the reliability of your code! 🚀
Now that you've learned about the alternative method, it's time to level up your C programming skills! Test out the strtol()
function in your code and see the difference it makes. Share your experience with us in the comments below. Let's help each other become better programmers! 💻🤝
Stay tuned for more exciting tech tips, coding hacks, and in-depth tutorials on our blog. Don't forget to subscribe to our newsletter for regular updates, and follow us on social media for even more engaging content. Together, let's continue to explore the fascinating world of programming! 📚🌐
Happy coding! 😄✨