How to achieve function overloading in C?
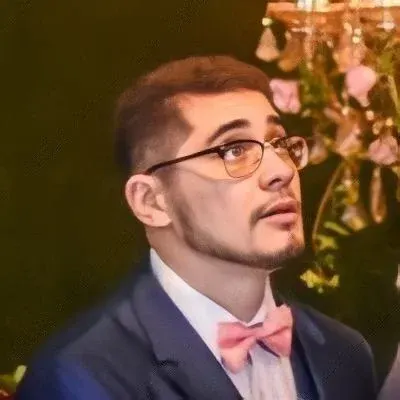
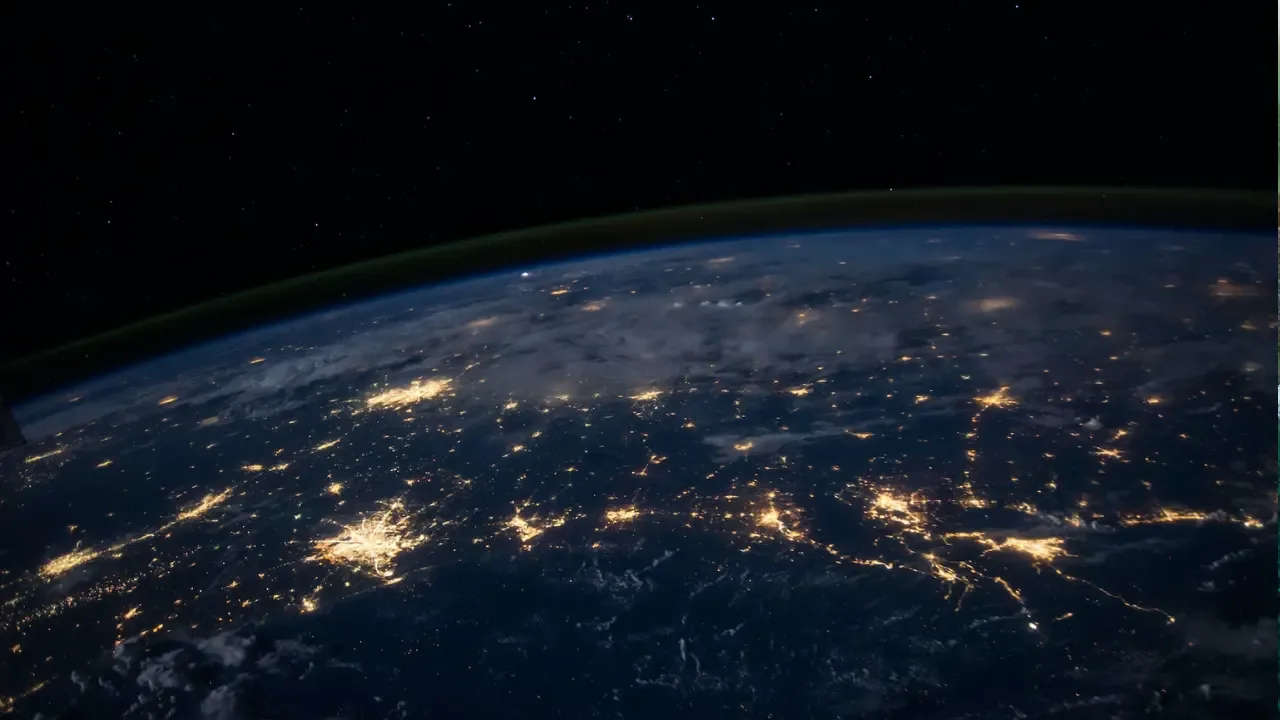
Achieving Function Overloading in C: A Guide to Bridging the Gap
š¢ Calling all C programmers! Are you tired of not being able to achieve function overloading in C? Do you find yourself longing for the simplicity and flexibility of this powerful feature? Look no further! In this blog post, we'll explore common issues surrounding function overloading in C and provide you with easy and clever solutions to tackle them head-on. So, let's dive right into it! šŖ
Understanding the Problem
š The question at hand is whether it's possible to achieve function overloading in C. Function overloading, the ability to have multiple functions with the same name but different parameter lists, is a well-loved feature in languages like C++. Unfortunately, C doesn't provide direct support for function overloading out of the box.
š¤ So, how do we solve this problem in the absence of native function overloading support? Fear not, my fellow developers, for we have a couple of workarounds up our coding sleeves! Let's explore them one by one. š
Method 1: Differentiating Function Names
šÆ The first workaround is to give each function a different name that reflects its purpose or parameter type. Let's take a look at an example:
void foo_int(int a) {
// Function implementation for integer parameter
}
void foo_char(char b) {
// Function implementation for character parameter
}
void foo_float_int(float c, int d) {
// Function implementation for float and integer parameters
}
In this approach, we explicitly differentiate the function names based on the parameter types. While this method achieves similar functionality to function overloading, it does require more naming creativity and may lead to longer function names. However, it is straightforward to implement and understand.
Method 2: Emulating Function Overloading with a Single Function
šÆ The second workaround involves using a single function that can handle multiple types of parameters. This technique is known as function overloading simulation. Here's an example:
void foo(void* params) {
if (params == NULL) {
// Handle error case
return;
}
// Perform type-specific operations based on the parameter type
if (typeof(params) == int) {
int a = *(int*)params;
// Function implementation for integer parameter
}
else if (typeof(params) == char) {
char b = *(char*)params;
// Function implementation for character parameter
}
else if (typeof(params) == float) {
float c = *(float*)params;
int d = *((int*)params + sizeof(float));
// Function implementation for float and integer parameters
}
else {
// Handle unsupported parameter type case
// Or perform other fallback actions
}
}
In this approach, we pass the function parameters as a generic void*
pointer and use type casting to access the actual values. By examining the parameter type, we can perform type-specific operations within the single foo
function. However, it's worth noting that this method adds runtime overhead and requires careful handling of different parameter types.
Wrap-up and Your Turn to Shine
š We've reached the end of our journey to achieve function overloading in C! We explored two workarounds: differentiating function names and emulating function overloading with a single function. While these methods may not be as elegant as native language support, they provide viable solutions to tackle the problem head-on.
š Now it's your turn to experiment and see which workaround suits your coding style and project requirements. Remember, mastering these workarounds will empower you to achieve function overloading-like behavior in your C projects, no matter the limitations. So, go forth, code, and conquer!
š¬ We'd love to hear about your experiences with function overloading in C! Have you encountered any challenges or discovered unique approaches? Share your thoughts, ideas, and code snippets in the comments below. Let's grow together as a community of passionate C programmers! š
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
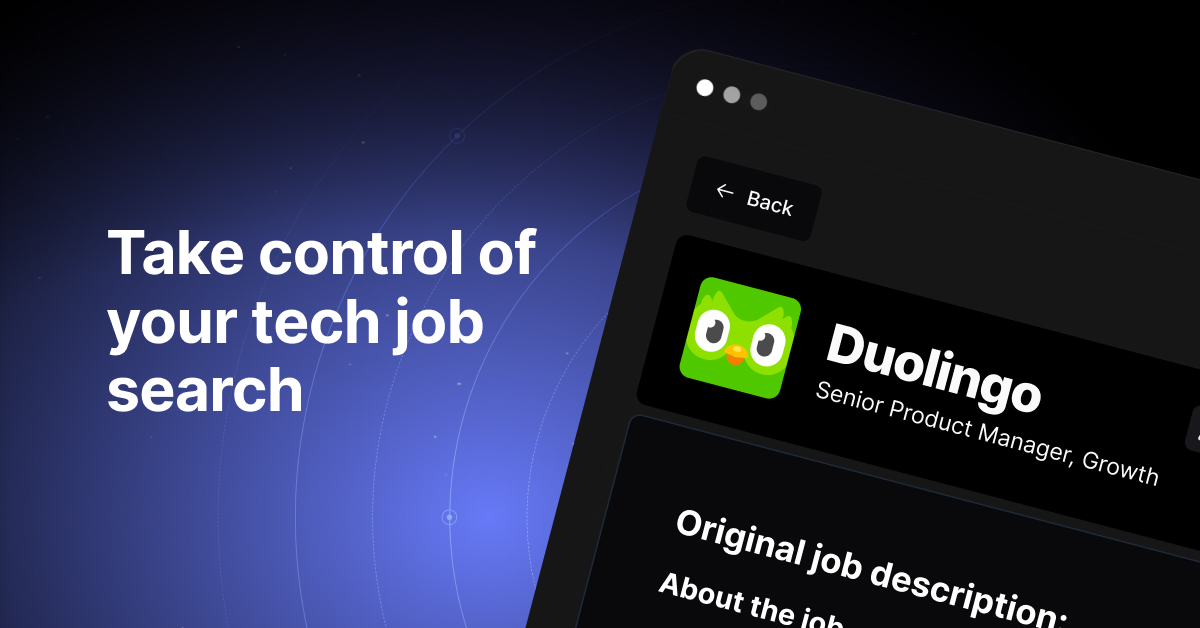