How do I create an array of strings in C?
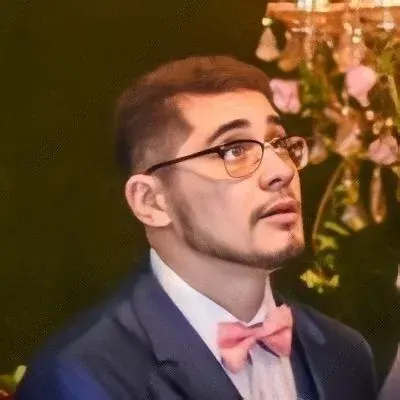
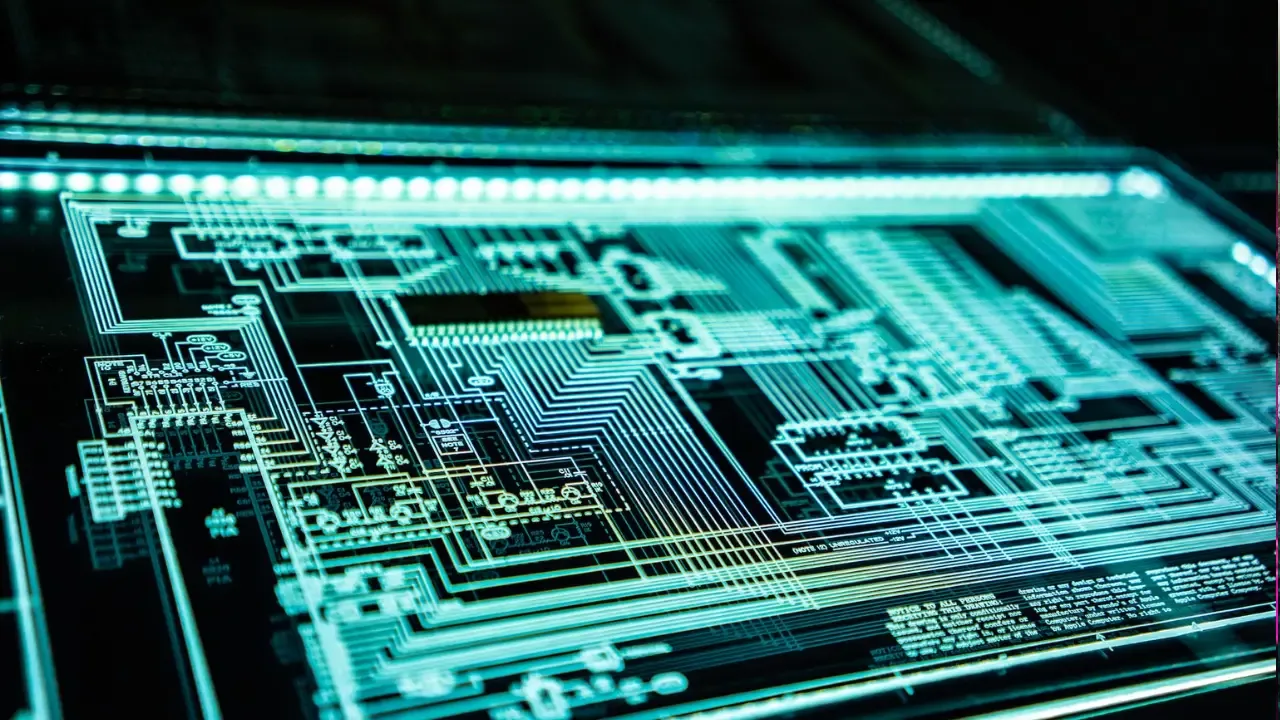
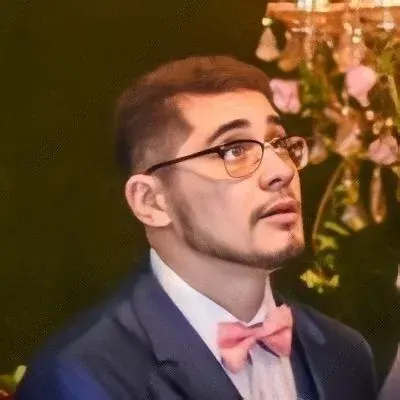
How to Create an Array of Strings in C 🌟
Creating an array of strings in C might seem daunting, but fear not! We're here to guide you through the process and provide easy solutions to overcome any obstacles. 🚀
The Warning: "Assignment from Incompatible Pointer Type" ⚠️
Let's begin by addressing the warning you encountered when running your code:
<pre><code>char (*a[2])[14]; a[0] = "blah"; a[1] = "hmm"; </code></pre>
The reason you received the warning "assignment from incompatible pointer type" is because you declared a
as an array of pointers to arrays of characters, with each array of characters having a size of 14. However, you attempted to assign string literals directly to a[0]
and a[1]
.
Solution 1: Using strcpy()
to Assign String Literals 📝
A simple solution to this problem is to use the strcpy()
function from the C standard library. strcpy()
allows you to copy the contents of one string to another.
Here's an example:
<pre><code>char a[2][14]; strcpy(a[0], "blah"); strcpy(a[1], "hmm"); </code></pre>
By declaring a
as a 2-dimensional array (char a[2][14]
), you can now use strcpy()
to copy the string literals into a[0]
and a[1]
respectively.
Solution 2: Using Array Initialization 🌈
If you prefer a more concise approach, C allows you to initialize arrays during declaration. You can take advantage of this feature to simplify your code:
<pre><code>char a[2][14] = {"blah", "hmm"}; </code></pre>
With this method, you no longer need to explicitly call strcpy()
. The array a
is declared and initialized in a single line.
🌟 Pro Tip: Working with Pointers to Strings
If you want to work with an array of pointers to strings instead of a 2-dimensional character array, you can accomplish that too!
Here's an example:
<pre><code>char *a[2] = {"blah", "hmm"}; </code></pre>
By declaring a
as an array of pointers to characters (char *a[2]
), you can directly assign string literals to each element of a
.
Wrapping Up and Encouraging Your Involvement 🏁
With these easy solutions, you can now confidently create an array of strings in C without encountering any warnings or errors. Remember to choose the method that best suits your needs and coding style.
If you found this blog post helpful, we encourage you to share it with fellow C programmers who might find it valuable. Let's spread the knowledge and make programming a little easier for everyone! 🌟
Have any questions or suggestions? Feel free to leave a comment and join the conversation. Happy coding! 💻✨