How do I concatenate const/literal strings in C?
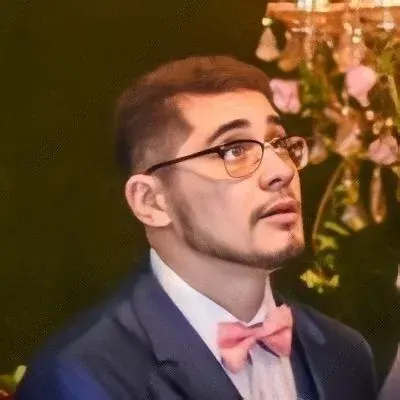
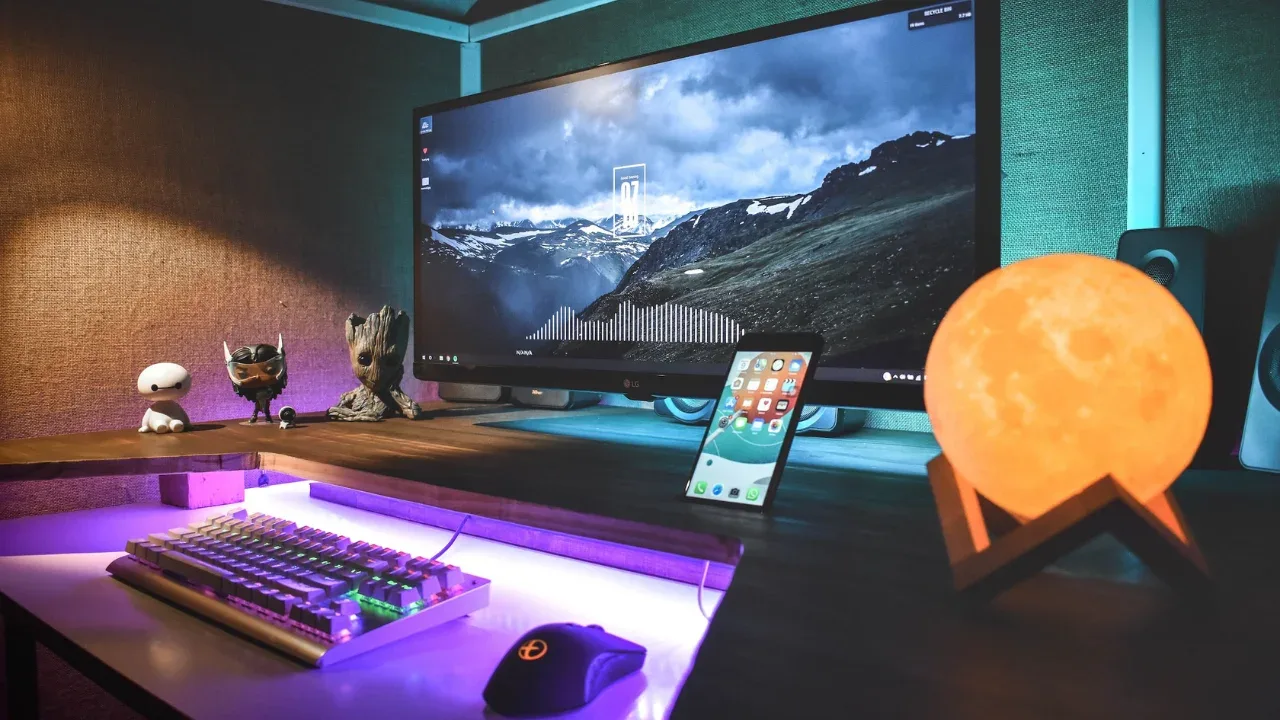
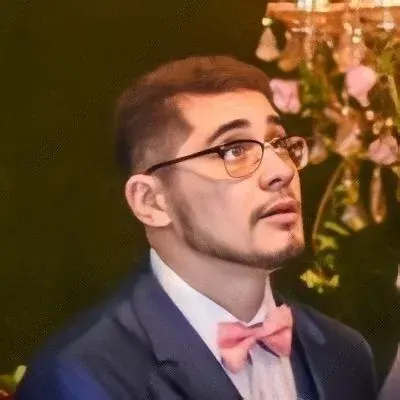
🧩 Concatenating Const/Literal Strings in C: A Guide 🧩
Are you struggling with concatenating const/literal strings in C? 😫 Don't worry, you're not alone! This common issue often leads to frustrating segmentation faults. But fear not, as I'm here to guide you through the process step by step! 🚀
The Problem 😵
Let's start with the problem at hand. The code you provided is attempting to concatenate strings using the strcat
function. However, this approach can lead to segmentation faults when dealing with const/literal strings. In C, const/literal strings are stored in read-only memory, making them unmodifiable. 🚫📝
Here's the code you mentioned:
message = strcat("TEXT ", var);
message2 = strcat(strcat("TEXT ", foo), strcat(" TEXT ", bar));
The Solution 💡
To concatenate const/literal strings in C without encountering segmentation faults, we need to use a different approach. Here are two simple solutions for you:
Solution 1: Combine strcpy
and strcat
🤝
To concatenate a const/literal string with a variable, follow these steps:
Declare a character array to store the concatenated string.
Use
strcpy
to copy the const/literal string into the new array.Use
strcat
to concatenate the variable string onto the end of the new array.
Here's an example:
char message[MAX_LENGTH]; // Declare a new character array
strcpy(message, "TEXT "); // Copy the const/literal string
strcat(message, var); // Concatenate the variable string
Solution 2: Use a Temporary Buffer 🔄
If you prefer a more concise approach, you can use a temporary buffer to hold the concatenated string before assigning it to your desired variable. Here's how:
char temp[MAX_LENGTH]; // Declare a temporary buffer
strcpy(temp, "TEXT "); // Copy the const/literal string
strcat(temp, var); // Concatenate the variable string
strcpy(message, temp); // Assign the concatenated string to your desired variable
Choose the solution that suits your coding style and project requirements. With either approach, you can safely concatenate const/literal strings in C without worrying about segmentation faults! 😄
Your Turn! 📝
Now it's your time to shine! Implement the solution that works best for you and let me know how it goes. If you encounter any issues or have any questions, feel free to leave a comment below. I'm here to help you out! 💪
Happy coding! ✨👨💻