How can one print a size_t variable portably using the printf family?
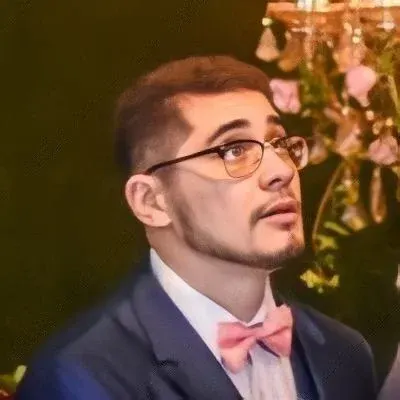
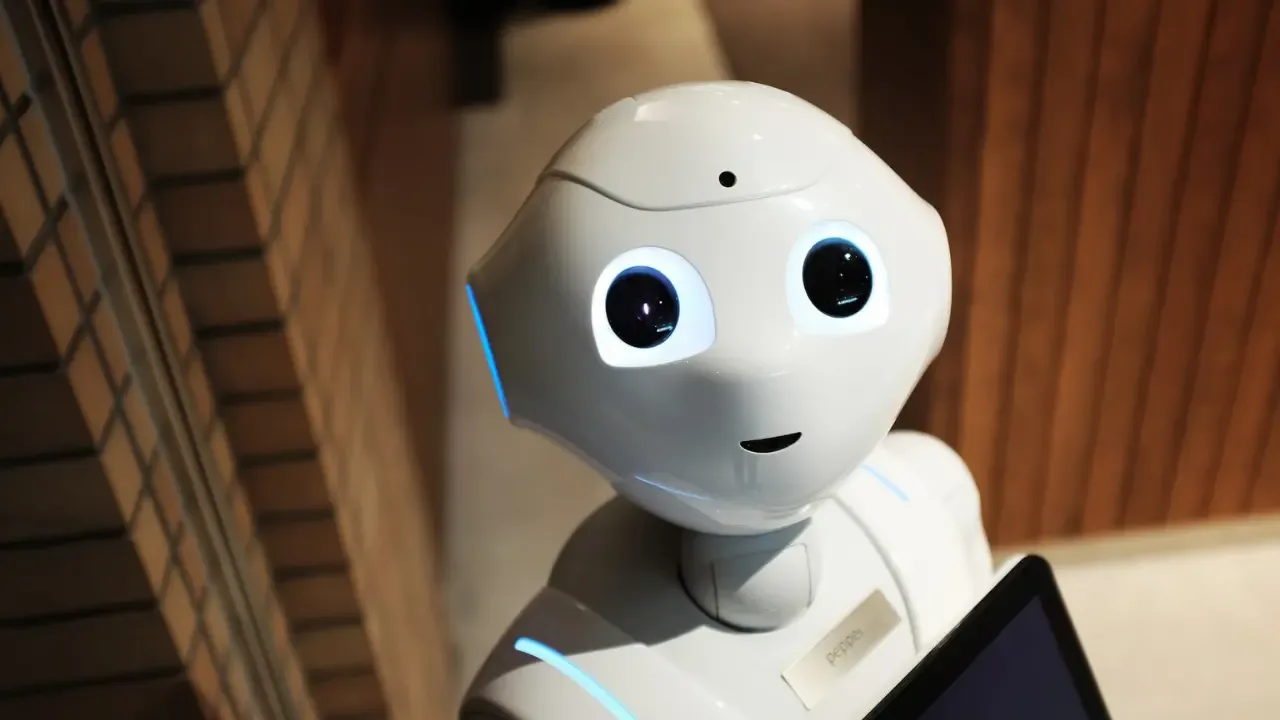
Printing a size_t
variable portably using the printf
family
So, you want to print a size_t
variable using printf()
and make sure it compiles without warnings on both 32-bit and 64-bit machines? 🤔 No worries, I've got you covered! In this blog post, I will address this common issue and provide you with easy solutions to ensure portability.
Understanding the problem 🕵️♀️
When you tried to print your size_t
variable using printf()
and used the %u
format specifier, it worked fine on your 32-bit machine without any warnings. However, when you compiled the same code on a 64-bit machine, it produced a warning about mismatched types.
The warning message indicates that the %u
format specifier expects an unsigned int
, but your size_t
variable is actually a long unsigned int
. This inconsistency between the expected and actual types is what causes the warning.
Easy solutions to ensure portability ✅
Solution 1: Use %zu
format specifier 📝
To print a size_t
variable portably, you can use the %zu
format specifier. This specifier is specifically designed for size_t
variables and ensures that the correct type is used regardless of the machine architecture.
Here's an example of how you can modify your code:
size_t x = <something>;
printf("size = %zu\n", x);
By using %zu
, you're telling printf()
to expect a size_t
variable and avoid any type mismatches or warnings.
Solution 2: Cast the variable to unsigned long
and use %lu
🔄
Another workaround is to cast your size_t
variable to an unsigned long
type and use the %lu
format specifier. This approach can be used if you encounter any issues with %zu
or if you need to support older compilers that don't fully adhere to the C99 standard.
Here's an example:
size_t x = <something>;
printf("size = %lu\n", (unsigned long)x);
This way, you are explicitly casting the size_t
variable to unsigned long
before printing it, ensuring compatibility with both 32-bit and 64-bit machines.
Take action and stay portable! 💪
Now that you have easy solutions at your disposal, go ahead and apply them to your code. By using either the %zu
format specifier or casting to unsigned long
with %lu
, you can print size_t
variables portably without encountering any warnings.
So, the next time you find yourself needing to print a size_t
variable using printf()
, remember these tips and stay worry-free about portability.
Got more questions or other ideas? Share them in the comments below, and let's keep the discussion going! 😄👇
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
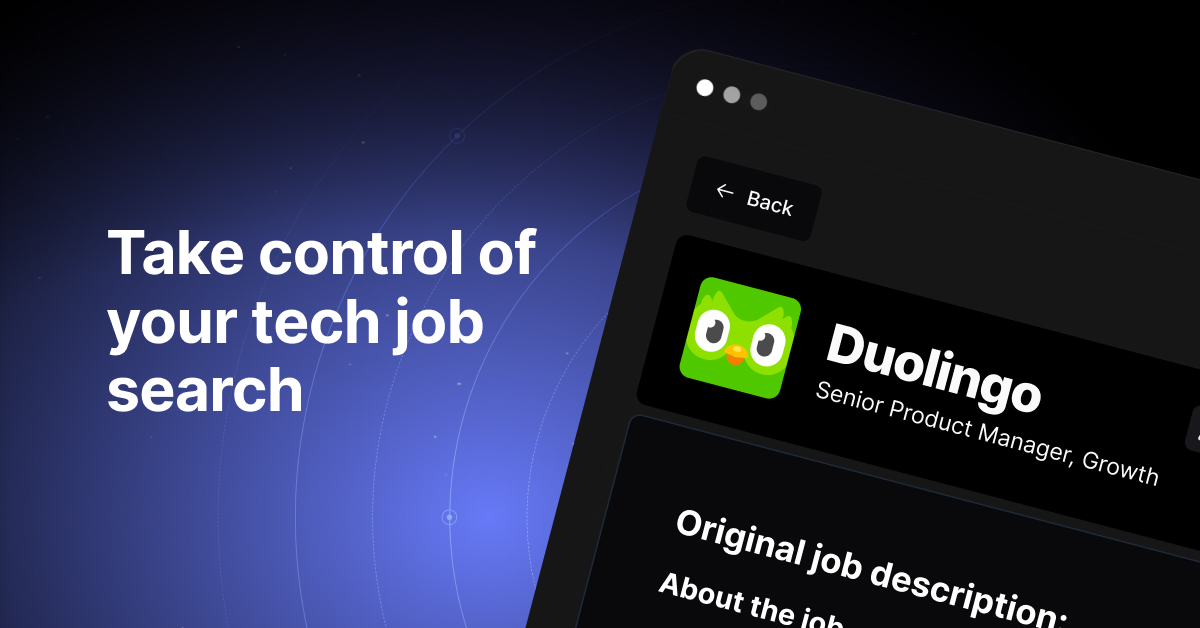