How can I convert an int to a string in C?
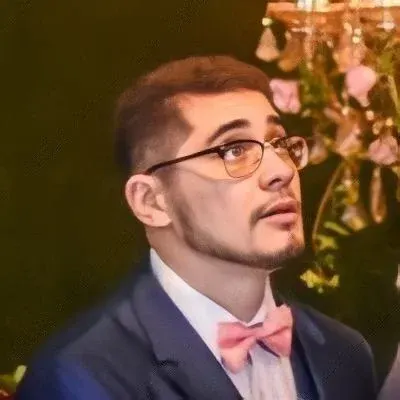

Converting an Int to a String in C: The Ultimate Guide ๐๐
So, you're trying to convert an int
to a string in C, huh? You've come to the right place! Whether you're trying to save data from a struct
to a file or performing any other operation that requires this conversion, it's quite a common task in the world of C programming.
In this comprehensive guide, we'll walk you through the entire process of converting an int
to a string in C. We'll cover common issues you may encounter along the way and provide simple, easy-to-understand solutions. ๐ ๏ธ๐ก
The Challenges ๐ง
Converting an int
to a string in C can be a bit tricky, especially if you're new to the language. Here are a few challenges you might face:
Loss of leading zeros: If the
int
value starts with leading zeros, directly converting it to a string may cause those zeros to be dropped.Memory allocation: You'll need to allocate memory for the resulting string to ensure enough space is available.
Error handling: It's important to handle errors gracefully when performing the conversion.
Now, let's dive into the solutions to these challenges and get you converting those int
values like a pro! ๐ช
Solution 1: Using the snprintf()
Function ๐ฅ๏ธ
To overcome the challenge of losing leading zeros, we can use the snprintf()
function. This function allows us to specify a minimum width for the integer value, ensuring leading zeros are preserved. Here's an example:
#include<stdio.h>
int main() {
int myInt = 42;
char myString[20];
snprintf(myString, sizeof(myString), "%02d", myInt);
printf("Converted string: %s\n", myString);
return 0;
}
In this example, we use %02d
as the format specifier within the snprintf()
function. The 0
in %02d
ensures leading zeros are added, and the 2
specifies a minimum width of 2 characters. Change the width value as needed for your specific requirements.
Solution 2: Dynamic Memory Allocation ๐ง ๐พ
If you don't know the exact size of the resulting string, you can dynamically allocate memory to accommodate it. Here's an example:
#include <stdio.h>
#include <stdlib.h>
char* intToString(int myInt) {
int length = snprintf(NULL, 0, "%d", myInt);
char* myString = malloc((length + 1) * sizeof(char));
snprintf(myString, length + 1, "%d", myInt);
return myString;
}
int main() {
int myInt = 123;
char* myString = intToString(myInt);
printf("Converted string: %s\n", myString);
free(myString);
return 0;
}
Here, we first use snprintf()
with NULL
as the destination buffer and 0
as the size, to determine the length of the string that will be formed (length
). Then, we allocate memory for the string using malloc()
and finally use snprintf()
once again to perform the actual conversion.
Don't forget to free the allocated memory once you've finished using the converted string to avoid memory leaks! ๐๐งน
Your Turn to Convert! ๐๐
Now that you have the tools in your hands, it's time to give it a try yourself! Go ahead and convert those int
values into strings, solve your programming challenge, and achieve greatness!
If you found this guide helpful, don't forget to share it with your fellow developers! And if you have any additional questions or cool ways you've discovered to convert an int
to a string in C, let us know in the comment section below!
Happy coding! ๐จโ๐ป๐
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
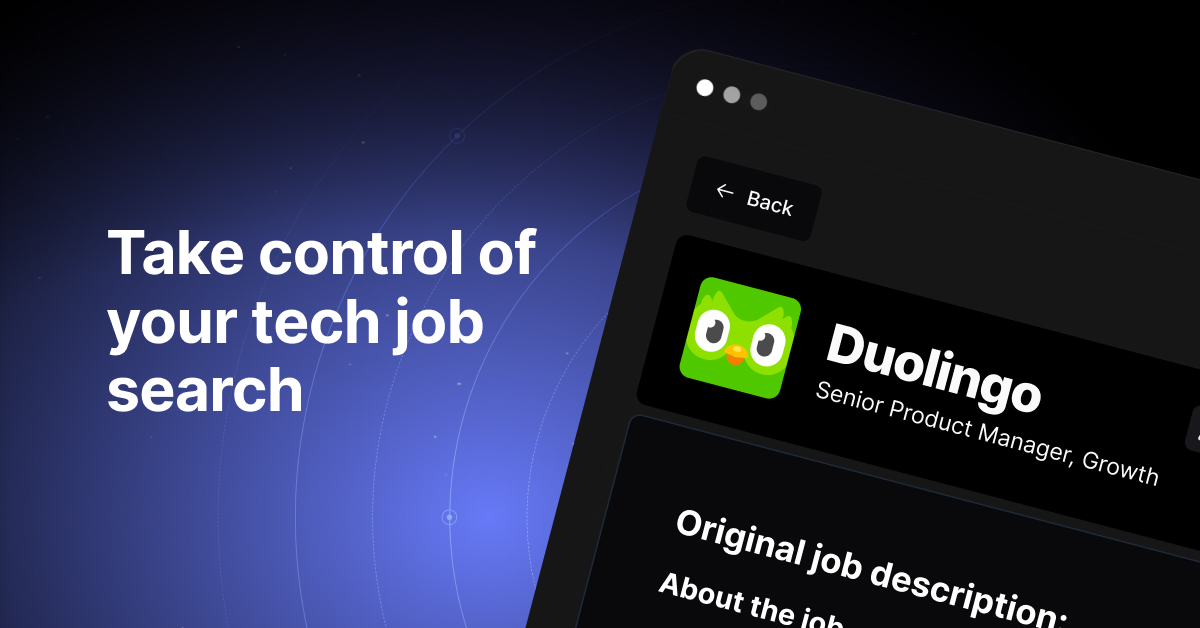