C default arguments
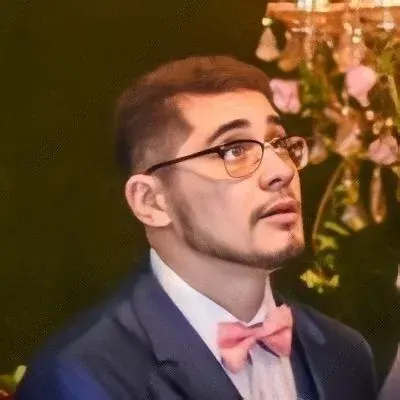
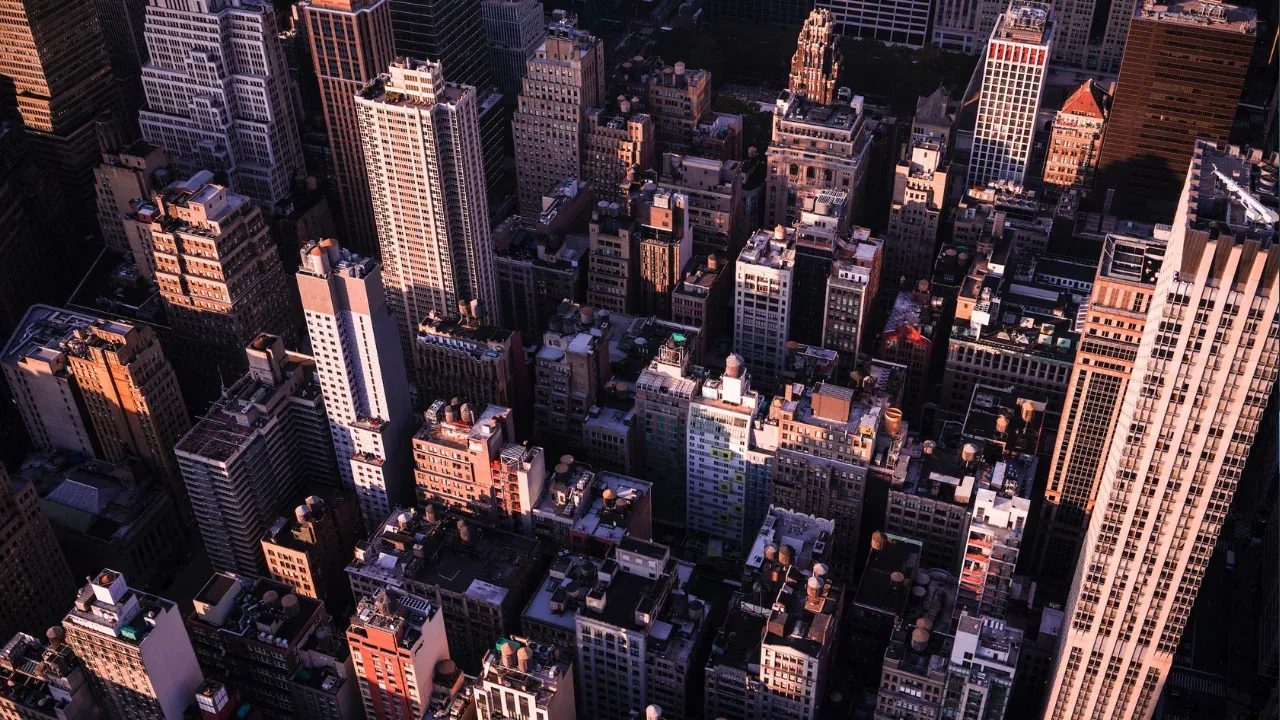
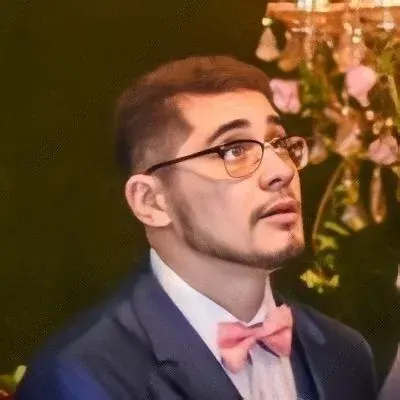
💻📝 Hey techies! Today I'm gonna talk about something that can make your life easier when coding in C – default arguments! 🌟📐
So you've got a function in C and you're wondering if there's a way to specify default arguments? Well, I'm here to tell you that unfortunately, C doesn't natively support default arguments like some other programming languages do. 😔🚫
But hey, don't fret! I've got a couple of workarounds for you to get the job done! 💪🛠️
1️⃣ The first option is to overload the function. What does that mean? Basically, you create multiple functions with the same name but different parameter lists – some with default values and some without. This way, you can call the function with or without certain arguments, and it will still work like a charm. Let me show you an example:
void myFunction(int arg1, int arg2, int arg3) {
// Do something awesome
}
void myFunctionWithDefaults(int arg1, int arg2) {
myFunction(arg1, arg2, DEFAULT_VALUE);
}
In this example, myFunctionWithDefaults
acts as a wrapper function that calls myFunction
with a default value for arg3
.
2️⃣ Another workaround is to use a struct to bundle the function arguments together. This way, you can create an instance of the struct and only set the values you want to override. The rest will be initialized to default values defined in the struct. Let's take a look at an example:
typedef struct {
int arg1;
int arg2;
int arg3;
} MyFunctionArgs;
void myFunction(MyFunctionArgs args) {
// Do something awesome
}
void myFunctionWithDefaults(MyFunctionArgs args) {
args.arg3 = DEFAULT_VALUE;
myFunction(args);
}
Here, MyFunctionArgs
is a struct that represents the function arguments. By setting a default value for arg3
in the myFunctionWithDefaults
function, we can achieve the same effect as default arguments.
🔔💡Now that you have a couple of ways to work around the lack of default arguments in C, go ahead and give them a try! Remember to choose the method that fits your coding style and project requirements best. Happy coding! 🎉💻
🤝📢 Do you have any other cool C tricks up your sleeve? Share your thoughts and experiences with default arguments or any other tips in the comments section below. Let's learn from each other and become better programmers together! 🚀🔥