Arrow operator (->) usage in C
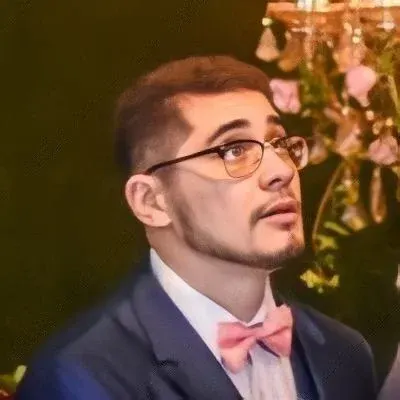
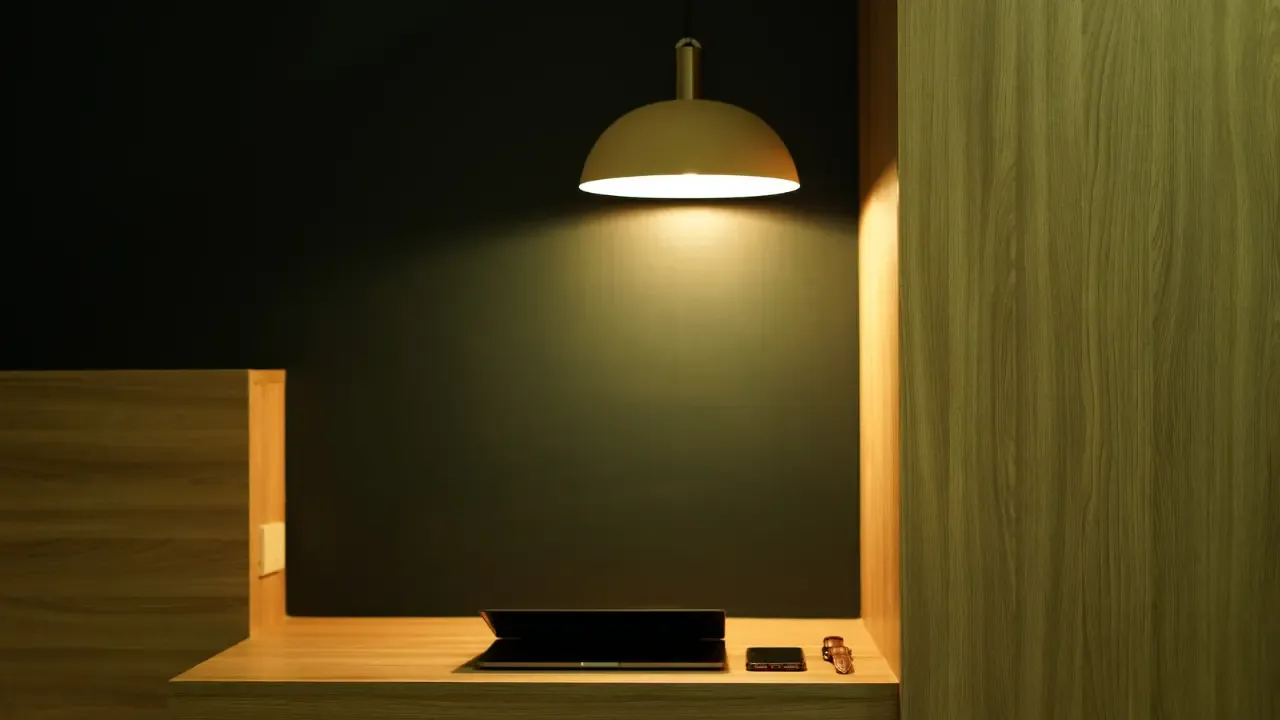
Understanding the Arrow Operator (->) in C š¹
š Hey there! Are you new to the world of pointers in C? Do you find the arrow operator (->) confusing? Don't worry, you're not alone! In this blog post, we'll dive into the usage of the arrow operator in C and provide you with easy-to-understand explanations and code samples. So let's get started, shall we?
What is the Arrow Operator?
The arrow operator (->) is used in C to access the members of a structure or union through a pointer. Consider the following code snippet:
struct Person {
char name[20];
int age;
};
struct Person person;
struct Person *personPtr = &person;
In the code above, we have a structure called "Person" with two members - "name" and "age". We also have a variable "person" of type "Person" and a pointer "personPtr" pointing to the address of "person".
Accessing Members with the Arrow Operator
To access the members of a structure or union through a pointer, we use the arrow operator (->) followed by the member name. Here's how we can access the "name" and "age" members using the arrow operator:
strcpy(personPtr->name, "John Doe");
personPtr->age = 25;
In the code above, we use the arrow operator to assign the value "John Doe" to the "name" member of the structure pointed to by "personPtr". We also assign the value 25 to the "age" member using the arrow operator.
The Equivalent Dot Operator
You might be wondering if the arrow operator is similar to the dot operator (.) used without pointers. And you're absolutely right! The arrow operator is essentially the pointer equivalent of the dot operator. Let's compare the two:
// Dot Operator
person.name = "John Doe";
person.age = 25;
// Arrow Operator
personPtr->name = "John Doe";
personPtr->age = 25;
In the code above, we can see that the dot operator is used when we have direct access to the structure itself, whereas the arrow operator is used when we have a pointer to the structure.
Code Sample
To illustrate the usage of the arrow operator, let's consider a simple example. We'll define a structure called "Point" representing a point in a 2D coordinate system:
struct Point {
int x;
int y;
};
// Function to create a point
struct Point* createPoint(int x, int y) {
struct Point* pointPtr = malloc(sizeof(struct Point));
pointPtr->x = x;
pointPtr->y = y;
return pointPtr;
}
int main() {
struct Point* point = createPoint(5, 10);
printf("Point coordinates: (%d, %d)", point->x, point->y);
free(point);
return 0;
}
In the code above, we create a point using the "createPoint" function, which returns a pointer to the dynamically allocated "Point" structure. We then use the arrow operator to access the "x" and "y" members of the structure pointed to by "point" in the main function.
Conclusion and Call-to-Action
Congratulations! You've made it through our exploration of the arrow operator in C. We hope that this blog post has provided you with a clear understanding of how to use the arrow operator to access the members of a structure or union through a pointer.
Don't stop here! Put your newfound knowledge to practice by experimenting with the arrow operator in your own C projects. š And if you have any questions or want to share your experiences, let us know in the comments below. Happy coding!
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
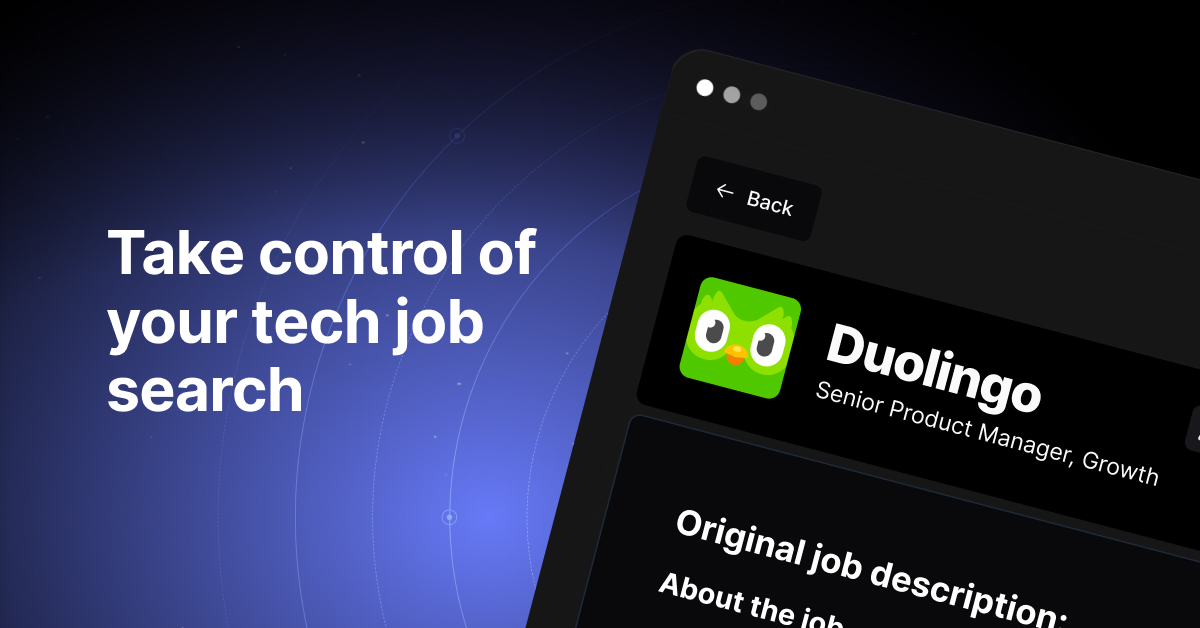