Return XML from a controller"s action in as an ActionResult?
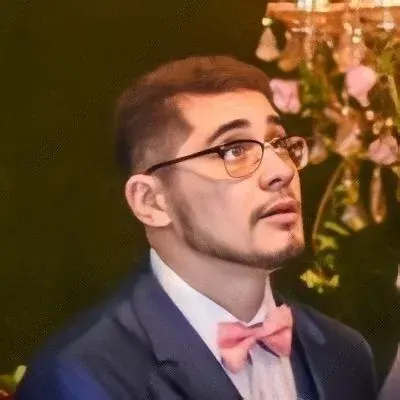
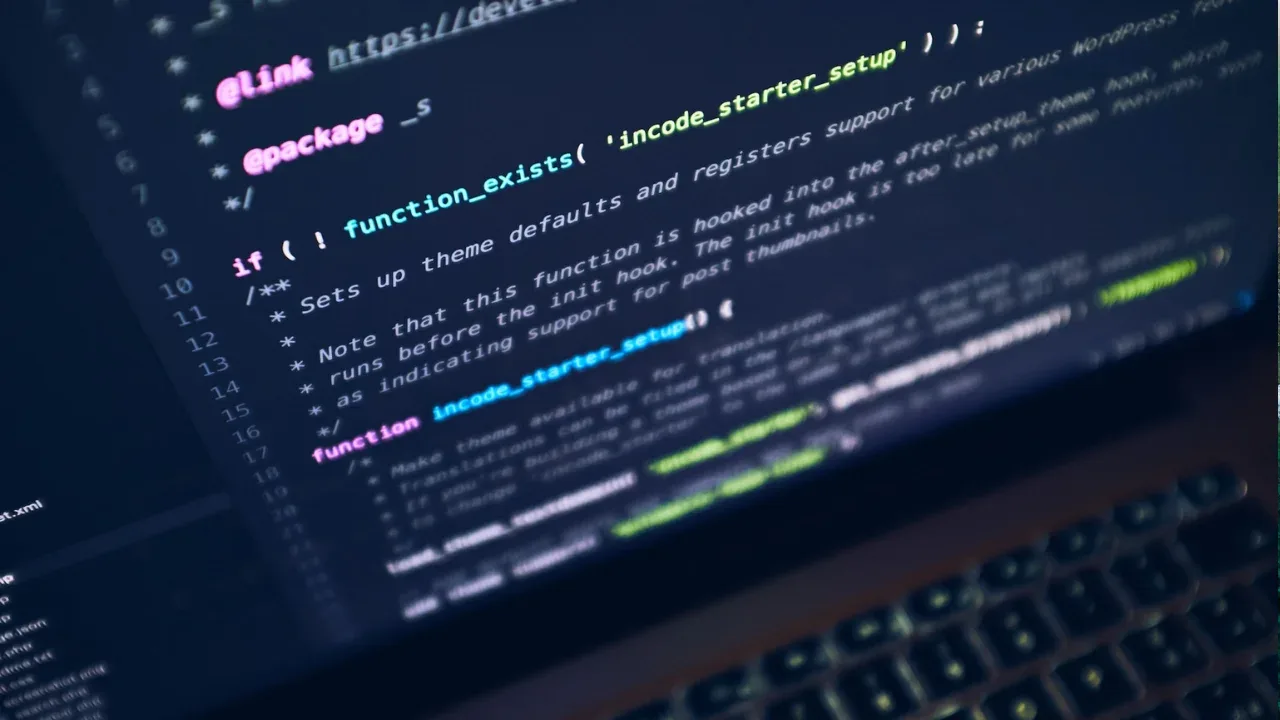
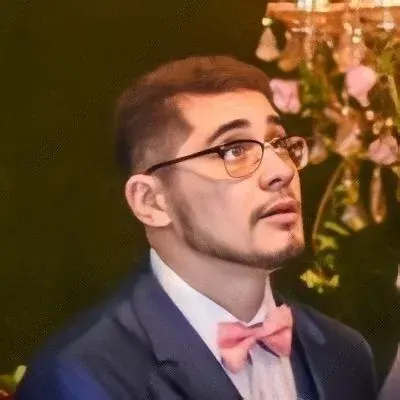
š Blog Post: "Return XML from a Controller's Action in ASP.NET MVC: Easy Solutions"
š Hey there, tech enthusiasts! Welcome back to our blog. Today, we'll tackle a common issue in ASP.NET MVC: returning XML from a controller's action. šš®
š¤ Are you tired of only finding smooth solutions to returning JSON, but struggling with XML? Fear not, because we've got you covered! Let's dive right into the details. šŖ
š The Problem:
Fellow developers have often wondered: "What is the best way to return XML from a controller's action in ASP.NET MVC? Do I really need to route the XML through a view, or can I get away with using the not-so-best-practice method of Response.Write
?"
Well, worry not! We're here to share the best practices and easy solutions to get your XML data flowing seamlessly. š
š” The Solution - Option 1: Leveraging ActionResult:
Rather than utilizing Response.Write
, ASP.NET MVC provides a cleaner approach using the ActionResult
class. This class represents the result of an action method and can be used to encapsulate different types of responses, including XML. š„šļø
To return XML using ActionResult
, you can make use of the ContentResult
class. This class allows you to pass the XML content and specify the content type as "text/xml." Here's an example:
public ActionResult GetXml()
{
// Create your XML content
string xmlContent = "<root><value>Content returned as XML!</value></root>";
// Return the XML as an ActionResult
return Content(xmlContent, "text/xml");
}
By returning a ContentResult
with the appropriate content type, you can seamlessly deliver XML data. šš»
š” The Solution - Option 2: Utilizing JsonResult:
Believe it or not, another way to return XML is by making use of the JsonResult
class. Although it's primarily designed for JSON, we can take advantage of its flexibility. Just make sure to set the content type to "text/xml" and serialize your XML content appropriately. Here's an example:
public ActionResult GetXml()
{
// Create your XML content
XElement xmlContent = new XElement(
"root",
new XElement("value", "Content returned as XML!")
);
// Return the XML as an ActionResult
return new JsonResult
{
Data = xmlContent.ToString(),
ContentType = "text/xml",
JsonRequestBehavior = JsonRequestBehavior.AllowGet
};
}
By creating an XML structure using XElement
and setting the appropriate content type, you can also return XML using JsonResult
. š±š
š In both solutions, remember to map your action method in the controller's routes to ensure proper routing! š¦
⨠Call-to-Action:
And there you have it! Two easy solutions to return XML from a controller's action in ASP.NET MVC. Now it's your turn: give these methods a try and let us know in the comments below which one worked best for you. š¤©š
If you found this blog post helpful, don't forget to share it with your fellow developers. Sharing is caring! Let's spread the knowledge. šš”
Until next time, happy coding! š»āļø