Writing/outputting HTML strings unescaped
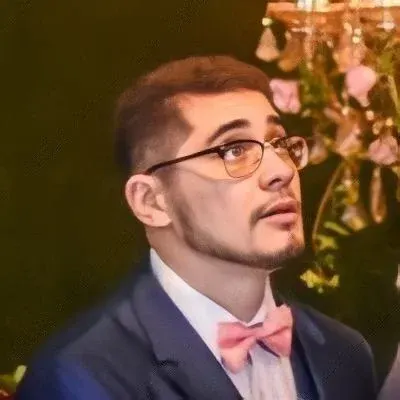
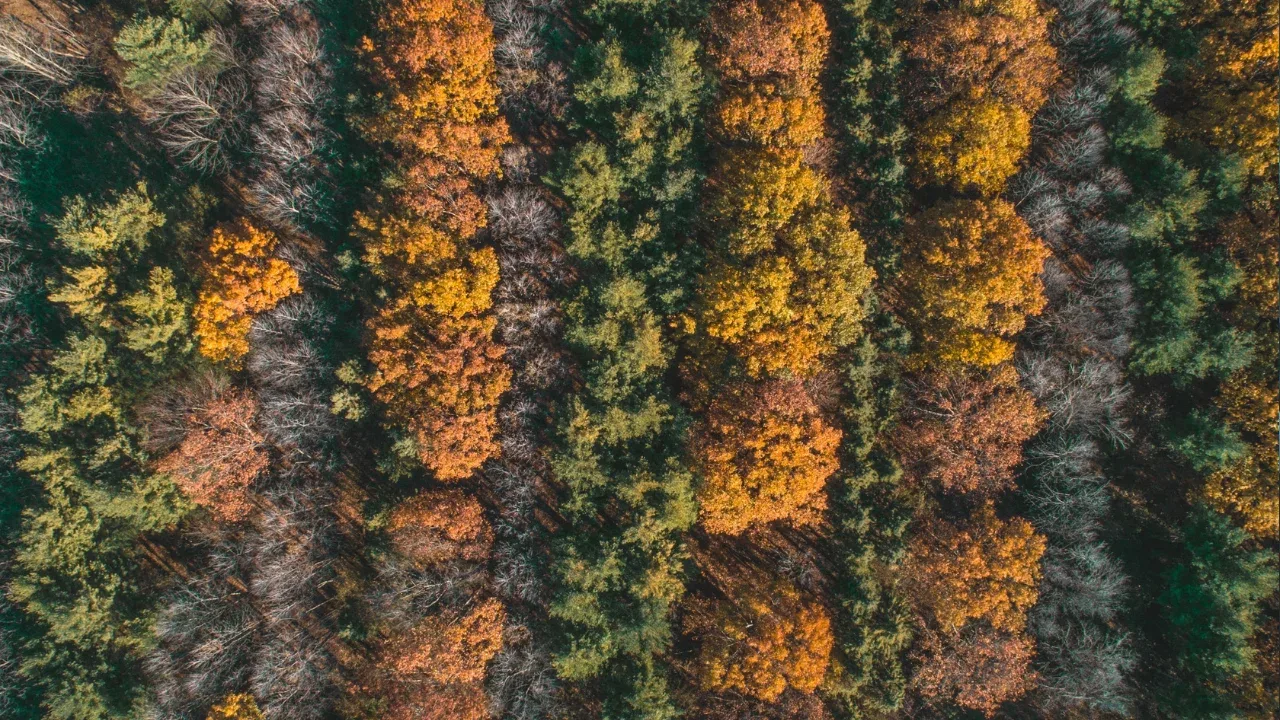
Unlocking the Magic of Unescaped HTML Strings in Your Razor Views
<div align="center"> <img src="https://i.imgur.com/1aMLU1g.png" alt="HTML code" width="400" height="300"/> </div>
So, you've got this blazing π feature where you've saved some squeaky-clean and sanitized HTML in your DB table, all ready to be unleashed upon the world. But hold up, what's this? π± Every time you try to output it in your Razor view, those pesky characters like <
and &
get transformed into their entity codes, rendering your beautiful HTML useless. Fear not! π‘οΈ We've got the solutions you need to conquer this problem and bring your HTML to life in all its unescaped glory.
The Issue at Hand π€·ββοΈ
Let's quickly recap. You have safely stored HTML content in your database table, and you want to display it in a Razor view. However, when you output the HTML using Razor's standard method, it escapes characters like <
and &
into their entity codes <
and &
. And that's a big ol' π« NO-NO because it messes up your intended layout and functionality. Yikes! But don't despair, my tech-savvy friend! We have not one, but three super cool solutions to eradicate this problem.
Solution 1: Using Html.Raw()
to Maintain Sanity π§
Have you heard of the magical Html.Raw()
function? πͺ If not, hold onto your hats! This little powerhouse will make all your unescaped HTML dreams come true. Simply wrap your HTML string with Html.Raw()
, sit back, and watch the magic happen β¨. This will prevent Razor from escaping the characters and preserve your HTML in its true form. Just take a look at this example:
<p>Safety first! Here's your sanitized HTML:</p>
@Html.Raw(Model.YourSafeHtmlString)
And voila! Your content will be displayed exactly as you saved it β no more pesky entity codes to ruin the day π.
Solution 2: Embrace the @
Symbol π€
Don't worry, we're not talking about a secret handshake here! We're talking about leveraging the power of the humble @
symbol in Razor views to achieve unescaped HTML goodness. Simply prepend your HTML string with @
to create a code block, like so:
<p>Catch that unescaped HTML in action:</p>
@{ var safeHtml = Model.YourSafeHtmlString; }
@safeHtml
And there you have it! You're making Razor dance to your tune, displaying the HTML just the way you want it β scandalously unescaped π.
Solution 3: Custom Helpers to the Rescue! π¦ΈββοΈ
Sometimes, it's not just about being a hero but creating one. How about creating your own custom helper method to handle the unescaped HTML conundrum? π€ With this approach, you can encapsulate the logic in a reusable and cleaner manner. Here's how you can define your custom helper:
using Microsoft.AspNetCore.Html;
using Microsoft.AspNetCore.Mvc.Rendering;
public static class HtmlHelperExtensions
{
public static IHtmlContent DisplaySafeHtml(this IHtmlHelper htmlHelper, string content)
{
return new HtmlString(content);
}
}
With your mighty helper defined, you can now use it in your Razor views without a hitch:
<p>Behold! Your unescaped HTML, wrapped in a custom helper:</p>
@Html.DisplaySafeHtml(Model.YourSafeHtmlString)
BOOM! π₯ Watch as your custom helper swoops in to save the day, keeping your HTML intact and free from all those nefarious entity codes.
Empowerment Awaits! π
Now that you have conquered the enigmatic problem of escaped HTML in your Razor views, it's time to take your newfound knowledge and save the world! πͺ So go forth, spread the word of unescaped HTML, and banish those entity codes for good.
What are your favorite techniques for handling unescaped HTML in Razor views? Share your wisdom in the comments below and let's unleash the power together! ππ
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
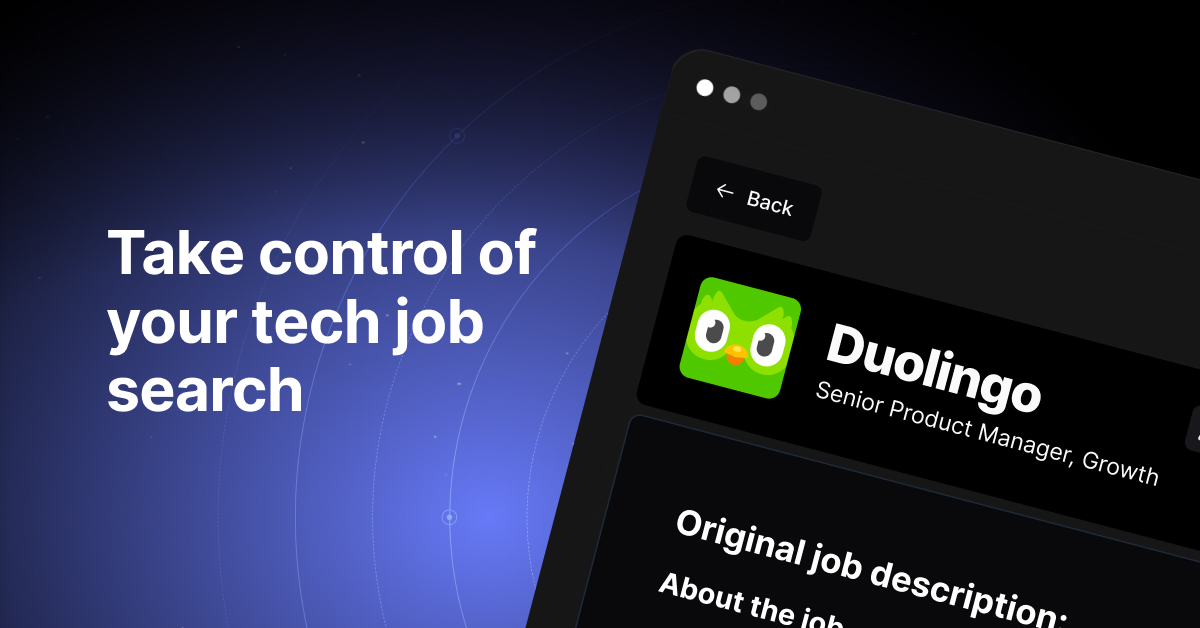