Why does AuthorizeAttribute redirect to the login page for authentication and authorization failures?
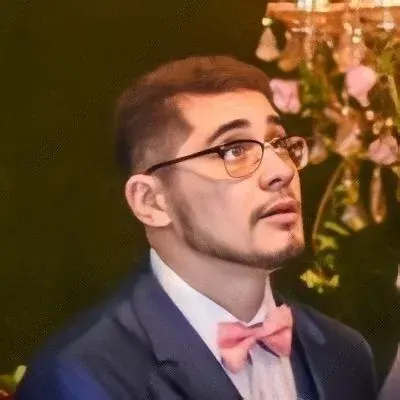
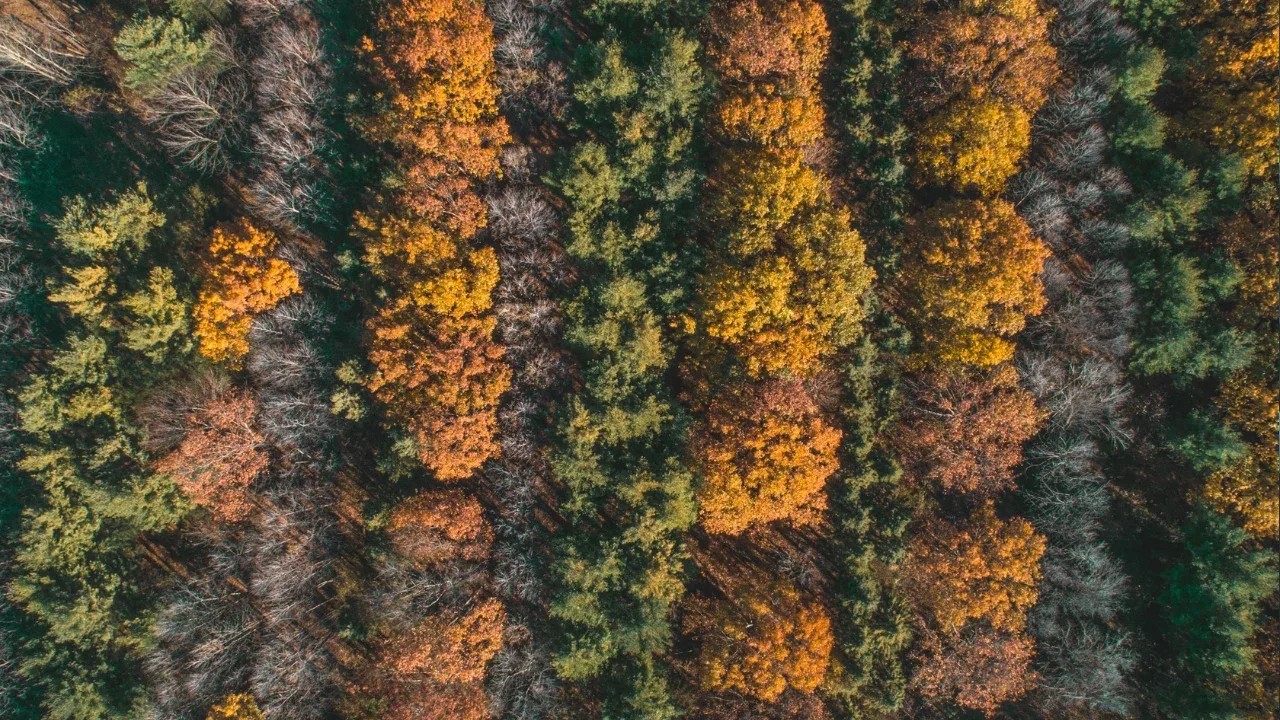
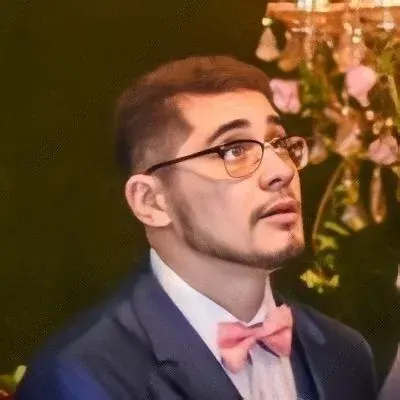
Why does AuthorizeAttribute redirect to the login page for authentication and authorization failures?
If you're using ASP.NET MVC, you might have encountered the AuthorizeAttribute
. This attribute allows you to mark up a controller method, indicating that only certain roles are authorized to access that method. For example, you could have a method for deleting tags that should only be accessible to users in the "CanDeleteTags" role.
[Authorize(Roles = "CanDeleteTags")]
public void Delete(string tagName)
{
// ...
}
But here's the catch - if the current user is not in the required role, the AuthorizeAttribute
will return an HttpUnauthorizedResult
, which always results in a redirect to the login page. 🚦
Now, if the user is not logged in, this behavior makes sense. They should be redirected to the login page to authenticate themselves. However, if the user is already logged in but doesn't have the necessary role, it can be quite confusing and frustrating to be sent back to the login page. 🚫➡️🔑
You might be wondering why the AuthorizeAttribute
behaves this way. Well, the truth is, it's a bit of an oversight in ASP.NET MVC. The AuthorizeAttribute
conflates authentication and authorization, treating them as one and the same. This means that it doesn't differentiate between an unauthenticated user and an authenticated user without the required role. 😕
But fear not! There is a solution to this issue.
The Solution: Separating authentication and authorization with DemandRoleAttribute
To address this problem, you can create your own attribute, let's call it DemandRoleAttribute
, which separates authentication and authorization. 🌟
public class DemandRoleAttribute : AuthorizeAttribute
{
protected override void HandleUnauthorizedRequest(AuthorizationContext filterContext)
{
if (!filterContext.HttpContext.User.Identity.IsAuthenticated)
{
base.HandleUnauthorizedRequest(filterContext);
}
else
{
filterContext.Result = new NotAuthorizedResult();
}
}
}
In this custom attribute, we override the HandleUnauthorizedRequest
method. If the user is not authenticated, we simply let the base HandleUnauthorizedRequest
method handle the request (which would redirect to the login page). However, if the user is already authenticated, we set the result to be a NotAuthorizedResult
instead. This gives you more control over how to handle unauthorized users. 🛠️
You can customize the NotAuthorizedResult
to fit your needs. It could redirect to an error page, display a custom message, or take any other action that makes sense for your application.
Conclusion: You shouldn't have had to do this, but you're not alone
It's unfortunate that the AuthorizeAttribute
in ASP.NET MVC doesn't handle authentication and authorization failures differently. It's a small oversight that can lead to confusion for users who are already logged in.
However, by creating a custom attribute like DemandRoleAttribute
, separating authentication and authorization becomes possible. It gives you more control and allows you to handle different scenarios appropriately. 🙌
So, if you've been scratching your head wondering why you're being redirected to the login page even when you're already logged in, fear not! You're not alone, and there's a solution available.
Have you encountered this issue before or found another way to deal with it? Let us know in the comments below! Let's share our experiences and help each other. 😊