What is ViewModel in MVC?
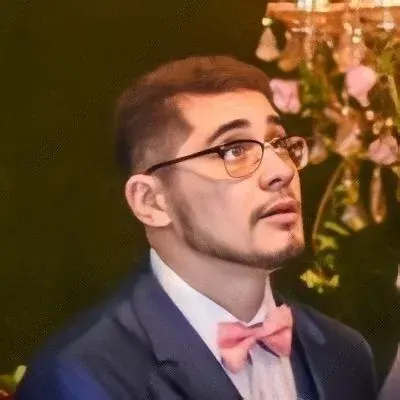
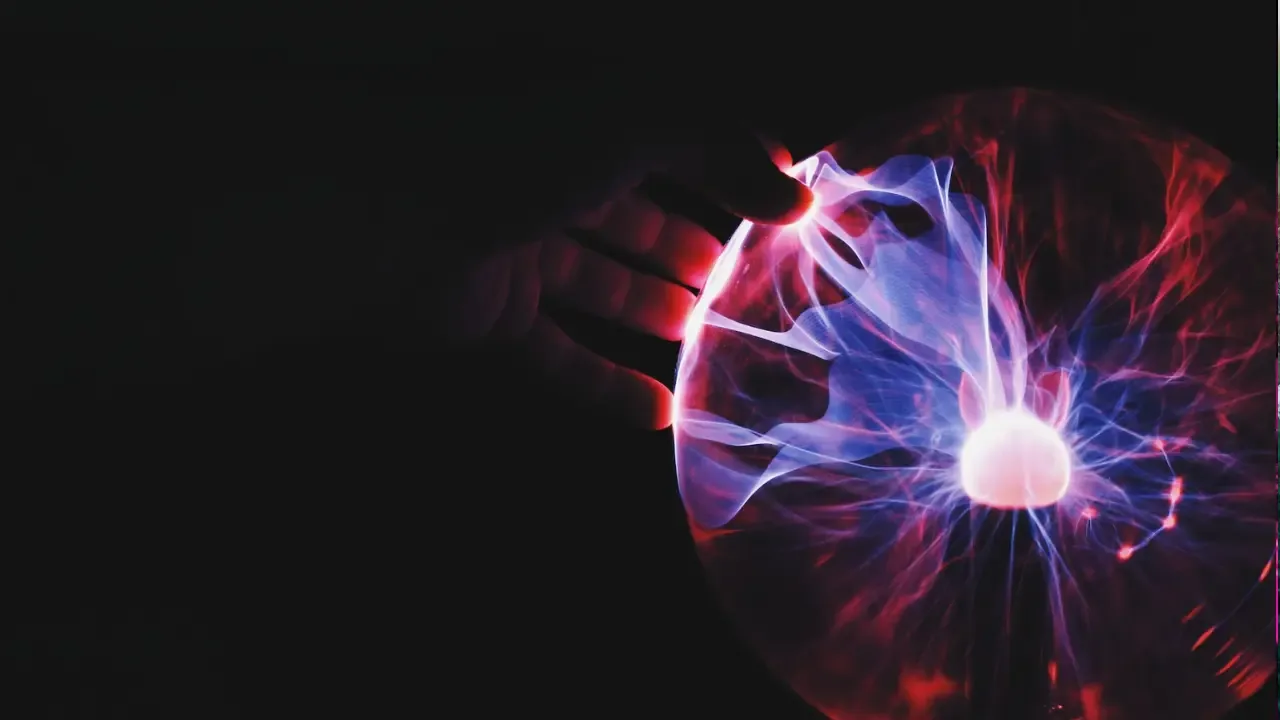
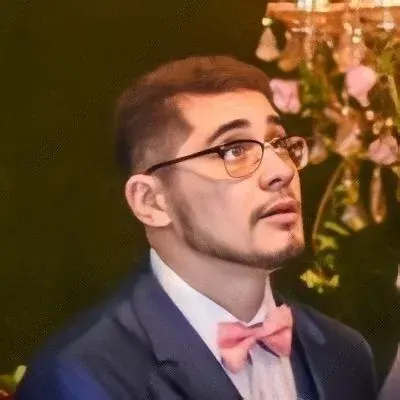
Demystifying the ViewModel in MVC: Your Guide to Clarity and Efficiency β¨π
Are you new to ASP.NET MVC? π€ Finding it hard to grasp the concept and purpose of a ViewModel? π€·ββοΈ Fear not, my tech-savvy comrades! In this blog post, we will demystify the enigma that is the ViewModel and provide you with a clear understanding of why it is vital for your ASP.NET MVC application. π―
π€ What is a ViewModel, anyway?
In MVC (Model-View-Controller) architecture, the ViewModel represents the data and behavior that the View (UI) requires to properly function. Simply put, it acts as an intermediary between the Model (data) and the View (UI). π
π§ Why do we need a ViewModel?
Imagine you have a View that needs to display data from multiple Models. Without a ViewModel, you would have to pass all the individual Model objects to the View, resulting in a mess of code πΎ. By utilizing a ViewModel, you can consolidate the necessary data into a single object, simplifying the code and improving maintainability. π
π‘ A Real-world Example
Let's take a practical example to help solidify the concept. Suppose you are building an e-commerce website, and you need to display product information along with some additional details, such as the user's shopping cart. π
Without a ViewModel, you would have to pass both the product and shopping cart objects to the View separately. This would lead to redundant code and increased complexity. π«
Enter the ViewModel! π¦ΈββοΈ By creating a ProductViewModel class that contains properties for the product and shopping cart, you can easily pass this single object to the View. This way, the View only needs to worry about working with the ViewModel, simplifying the development process. π
π Implementing the ViewModel
To implement a ViewModel in your ASP.NET MVC application, follow these simple steps:
Create a new class representing your ViewModel, e.g.,
ProductViewModel
.Add properties to the ViewModel class that correspond to the data you need to pass to the View, such as
Product
andShoppingCart
.In your Controller action method, create an instance of the ViewModel and populate its properties with the required data.
Pass the ViewModel object to the View as the model.
In the View, make sure to declare the ViewModel type in the
@model
directive at the top of the file.
Now, you can access the ViewModel properties directly in the View using Razor syntax, like @Model.Product
or @Model.ShoppingCart
. Easy peasy! π
β¨ Call-to-Action: Share Your ViewModel Victories!
Now that you're armed with the power of the ViewModel, go forth and conquer! π We'd love to hear about your experiences implementing ViewModels in your ASP.NET MVC applications. Have you encountered any challenges or discovered additional benefits? Share your stories in the comments below and let's keep the conversation going! π¬π
Remember, a well-implemented ViewModel can bring clarity, efficiency, and maintainability to your ASP.NET MVC codebase. Embrace it, simplify your life, and level up your development skills! πβ¨