Using Html.ActionLink to call action on different controller
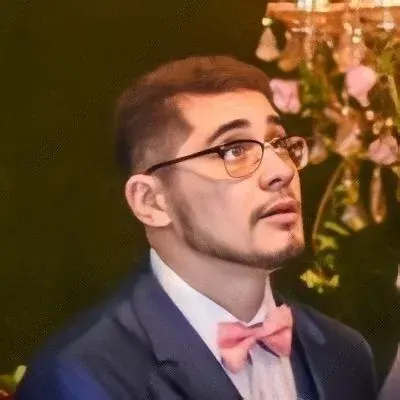
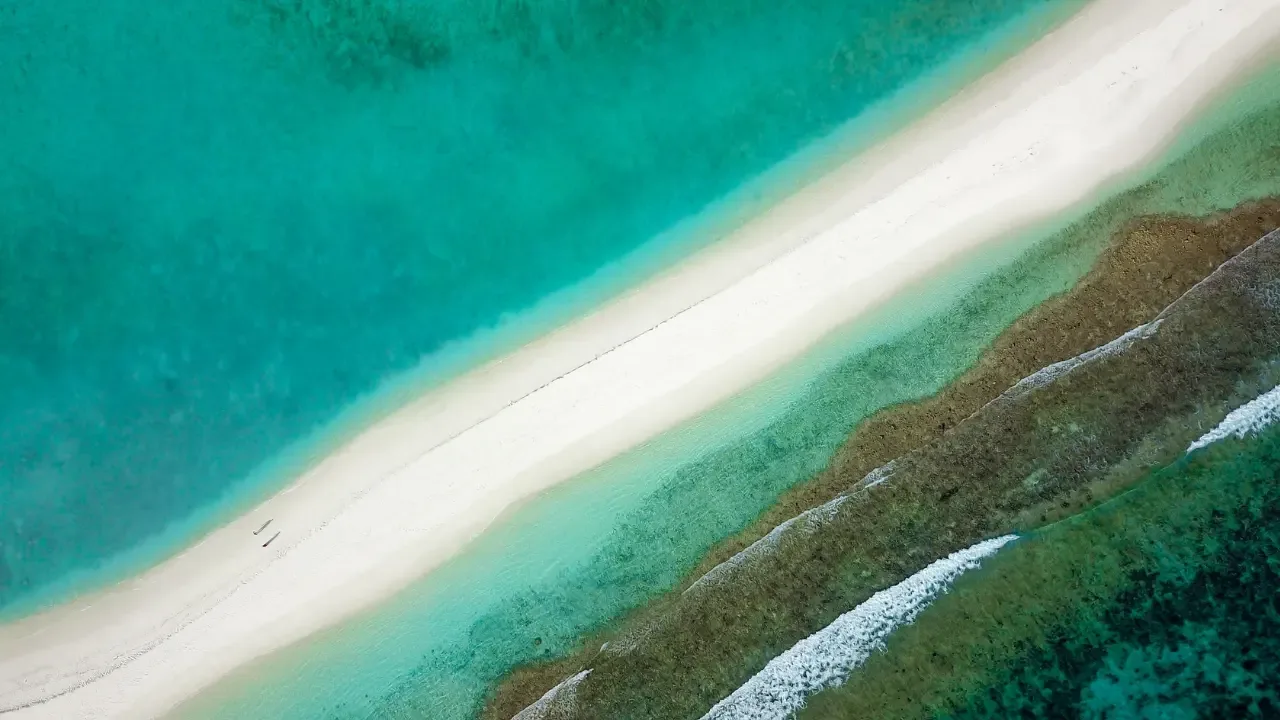
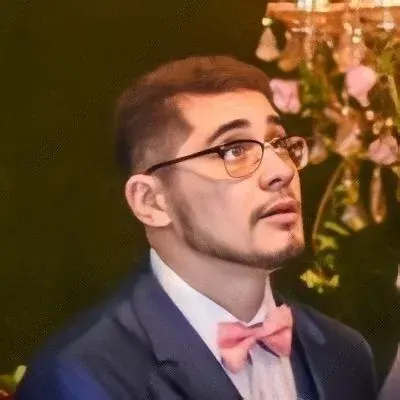
🖥️ ActionLink and the Trouble of Navigating Between Controllers
So, you're trying to use Html.ActionLink
to create a link from one controller to another, but for some reason, it's not working as expected. 😕 Don't worry, I've got your back! In this blog post, we'll dive into the common issue you're facing and provide an easy solution to help you navigate between controllers seamlessly. 🔄
The Problem: Generating the Wrong Link
Based on the example you shared, you're currently on the Index
view of the Hat
controller and want to create a link to the Details
action of the Product
controller. You've used the following code:
<%= Html.ActionLink("Details", "Details", "Product", new { id = item.ID }) %>
However, instead of generating a link to the Details
action on the Product
controller, it creates a link to the Details
action under the Hat
controller and appends a Length
parameter at the end. Oops!
The generated link looks like this: Hat/Details/9?Length=7
.
The Solution: Specify the RouteValues
Parameter
To fix this issue, you need to specify the RouteValues
parameter correctly in the Html.ActionLink
method. This parameter allows you to provide additional information required for routing, such as the target controller and action.
In your case, you can modify your code as follows:
<%= Html.ActionLink("Details", "Details", "Product", new { controller = "Product", action = "Details", id = item.ID }, null) %>
By explicitly specifying the controller
and action
values in the RouteValues
parameter, you ensure that the correct controller and action are used. Additionally, setting null
for the htmlAttributes
parameter ensures no additional HTML attributes are added to the link.
Additional Considerations: Default Routing
You mentioned that you're using the default route setting that comes with MVC. Here's a quick reminder of the default route configuration in the RouteConfig.cs
file:
routes.MapRoute(
"Default",
"{controller}/{action}/{id}",
new { controller = "Home", action = "Index", id = "" }
);
This configuration maps a URL pattern of /controller/action/id
to the corresponding controller, action, and optional ID parameter. Make sure this default route is properly set up in your application's routing configuration.
Wrapping Up: Time to Navigate Like a Pro!
With the corrected Html.ActionLink
code snippet and the default routing properly configured, you should now be able to navigate between controllers without any trouble. 👏
Feel free to try it out and let me know if you have any further questions or issues. Remember, technology is all about problem-solving and continuous learning! 🌟
Now it's your turn! Have you experienced any similar challenges while working with ActionLink
or navigating between controllers? Share your thoughts, experiences, or tips in the comments below. Let's help each other become better developers! 💪