Using Ajax.BeginForm with ASP.NET MVC 3 Razor
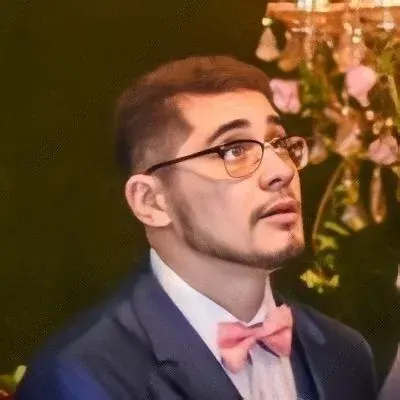
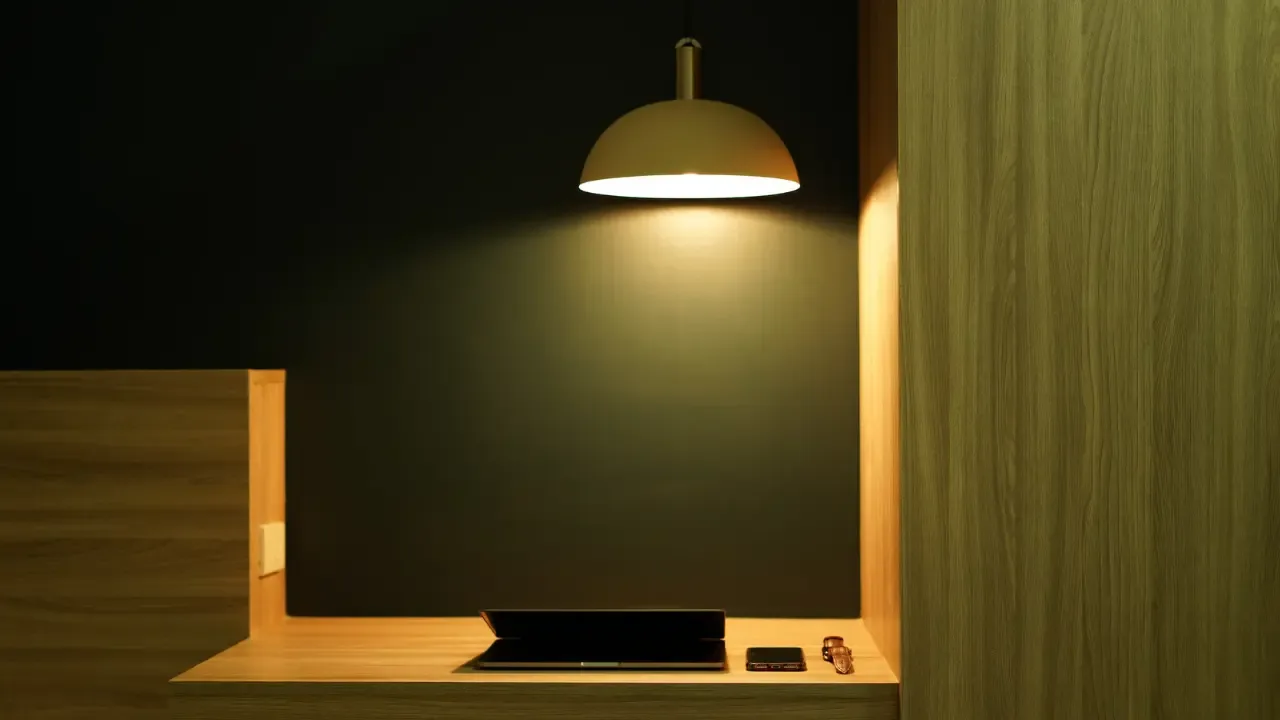
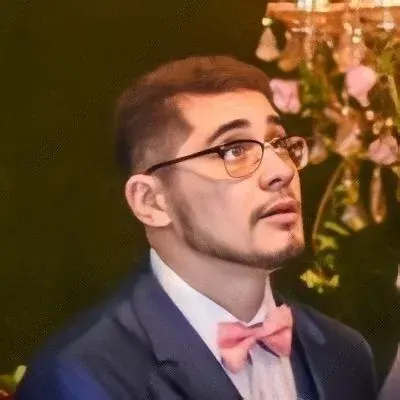
šš Hey there tech enthusiasts! In today's blog post, we're diving deep into the marvelous world of ASP.NET MVC 3 Razor and the magical powers of Ajax.BeginForm! šš»
Are you struggling with using Ajax.BeginForm within your ASP.NET MVC 3 application, where unobtrusive validation and Ajax reside? š¤ Fret no more, my friends, for we have got you covered! šŖšŖ
The Use Case: š Our fellow developer stated that they were having trouble making the form work properly. While it did submit via Ajax, it brazenly ignored those pesky validation errors. š±
Fear not, for we shall unravel this mystery together! Let's break it down step by step, shall we? š
Ensure that you have the following setup:
An up-to-date version of ASP.NET MVC 3 and Razor.
The
jquery.validate.unobtrusive.js
library included in your project. This library is responsible for the unobtrusive validation magic. š
Start by creating a simple form with validation attributes on your model properties. For example:
public class MyFormModel
{
[Required(ErrorMessage = "Please enter your name.")]
public string Name { get; set; }
}
In your Razor view, use the
Ajax.BeginForm
method to create your form. Make sure to include theAjaxOptions
parameter to enable Ajax functionality. Here's an example:
@using (Ajax.BeginForm("ActionMethod", "Controller", null, new AjaxOptions { UpdateTargetId = "myTargetDiv" }, new { id = "myFormId" }))
{
@Html.TextBoxFor(m => m.Name)
@Html.ValidationMessageFor(m => m.Name)
<input type="submit" value="Submit" />
}
<div id="myTargetDiv"></div>
Next, let's ensure that the unobtrusive validation and Ajax scripts are included in your layout or view file. You can do so by adding the following lines of code:
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
<script src="https://ajax.aspnetcdn.com/ajax/mvc/3.0/jquery.validate.unobtrusive.min.js"></script>
Finally, in your controller, create an
ActionMethod
that will handle the form submission. Make sure to decorate it with the[HttpPost]
attribute. Here's an example:
[HttpPost]
public ActionResult ActionMethod(MyFormModel model)
{
if (!ModelState.IsValid)
{
return PartialView("_MyFormPartialView", model); // Return the partial view with validation errors
}
// Process the form submission
// ...
return PartialView("_SuccessPartialView"); // Return a partial view indicating success
}
That's it! šāØ With these steps in place, your form should now submit via Ajax and display the validation errors if any arise. If the form submission is successful, it will display a partial view indicating success. Isn't that grand? š
Remember, troubleshooting is the key to success in the realm of coding. If you encounter any issues, double-check that you have followed each step carefully and that all the required libraries are included correctly. šš§
If you still find yourself scratching your head, don't hesitate to leave a comment below. Our tech community thrives on collaboration and helping each other out! Let's solve these pesky bugs together. š¤š
So go ahead, brave coders! Give it a try and let us know your thoughts in the comments. Happy coding! š»šŖš