Session variables in ASP.NET MVC
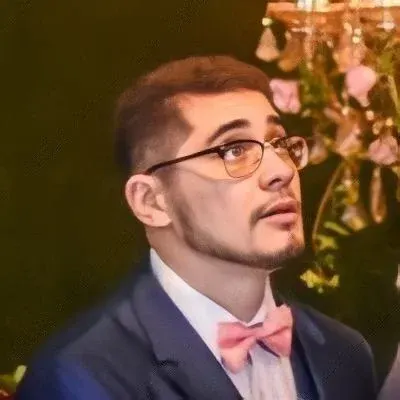
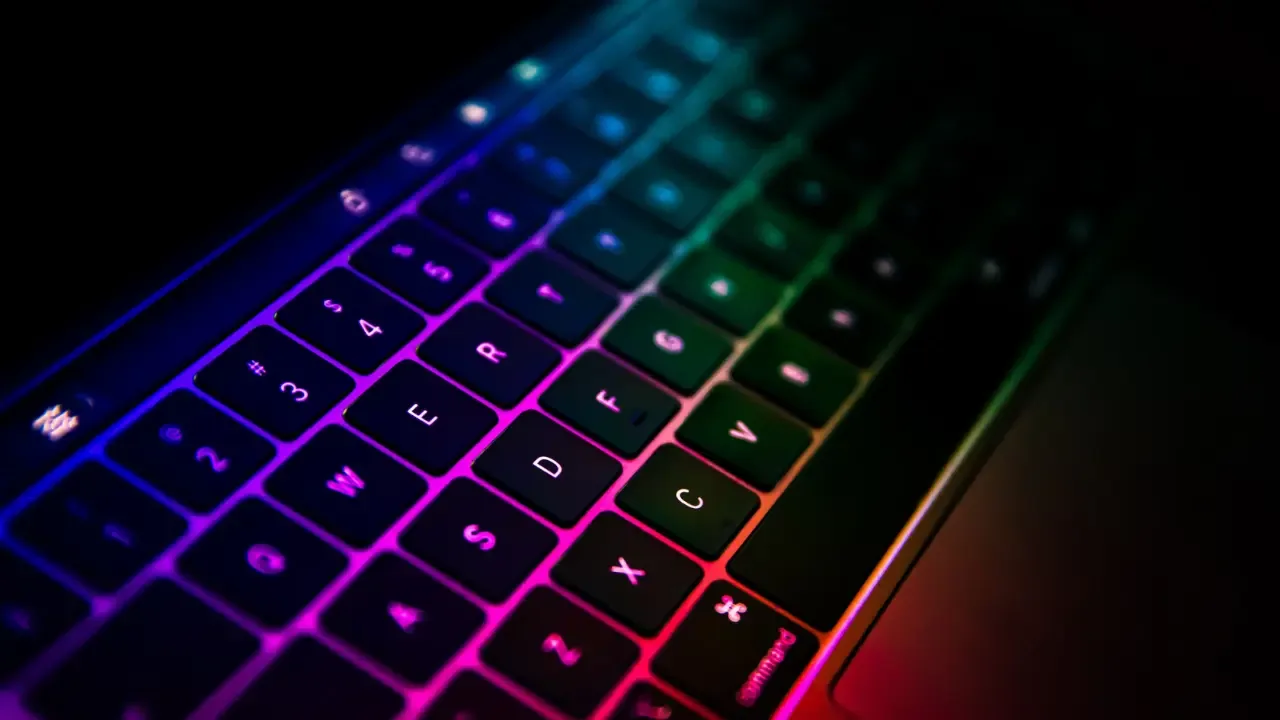
π‘ Understanding Session Variables in ASP.NET MVC
Are you building a web application in ASP.NET MVC and struggling to find a way to access user input and other data across multiple pages? ππ
Don't worry, my friend. I've got your back! In this blog post, we'll explore the power of session variables and how they can be your savior in these situations. π¦ΈββοΈπͺ
π€ What's the Problem?
Imagine you're working on a web application where users can navigate through various pages, making requests and inputting information. You want to store all this user input in an object and have the flexibility to access and use it from any part of the website. But how? π€·ββοΈ
π‘ The Session Variables Solution
Thankfully, session variables come to the rescue! π
Session variables in ASP.NET MVC allow you to store and retrieve data throughout a user's session on your website. They enable you to persist data across multiple requests and pages, providing a seamless user experience. π
π Implementing Session Variables
To start using session variables in ASP.NET MVC, you first need to enable session state in your web application. You can do this by following these simple steps:
Open the
Web.config
file in your project.Locate the
<configuration>
element.Inside
<configuration>
, add the following code:
<system.web>
<sessionState mode="InProc" timeout="20" />
</system.web>
In the above code, we've set the mode
attribute as "InProc"
, which means session state will be stored in the web server's memory. You can also choose other modes like "StateServer"
or "SQLServer"
depending on your application's requirements.
Optionally, adjust the
timeout
attribute value to set the session timeout duration (in minutes).
Once you've enabled session state, you can start using session variables in your controller actions. Here's an example:
public class ExampleController : Controller
{
public ActionResult StoreUserInput(string userInput)
{
Session["MyUserInput"] = userInput;
return RedirectToAction("NextPage");
}
public ActionResult NextPage()
{
string storedInput = Session["MyUserInput"] as string;
// Use the storedInput in your logic
return View();
}
}
In the above code, we store the user input in a session variable called "MyUserInput"
and retrieve it on the next page using the Session
object.
π₯ Other Alternatives?
While session variables are an excellent choice for persisting data across pages, there are alternatives you can consider based on your specific needs. Here are a few options:
Query Strings: You can pass data between pages using query strings. However, this may not be suitable for sensitive or large amounts of data.
Cookies: Cookies allow you to store data on the client-side. Though they are useful in some scenarios, keep in mind that they have size limitations.
Database: If you require permanent storage across sessions, consider storing the user data in a database. This gives you reliability and scalability.
Assess your requirements and choose the option that best suits your needs.
π£ Time to Level Up Your ASP.NET MVC Game!
Now that you're armed with the knowledge of session variables, go ahead and unlock the unlimited power of data persistence in your ASP.NET MVC applications! π₯π
Have any questions or faced any session variable-related challenges? Share them in the comments section below! Let's solve those brain-teasers together! ππ
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
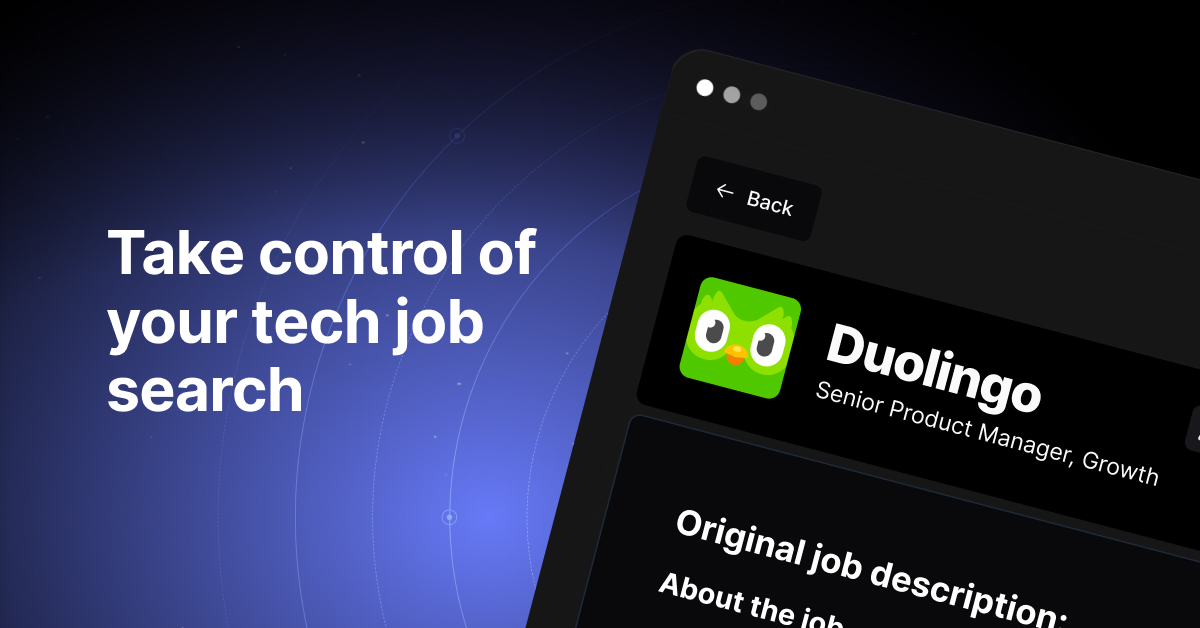