MVC 4 Razor File Upload
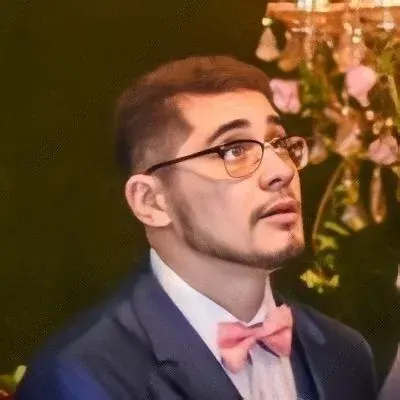
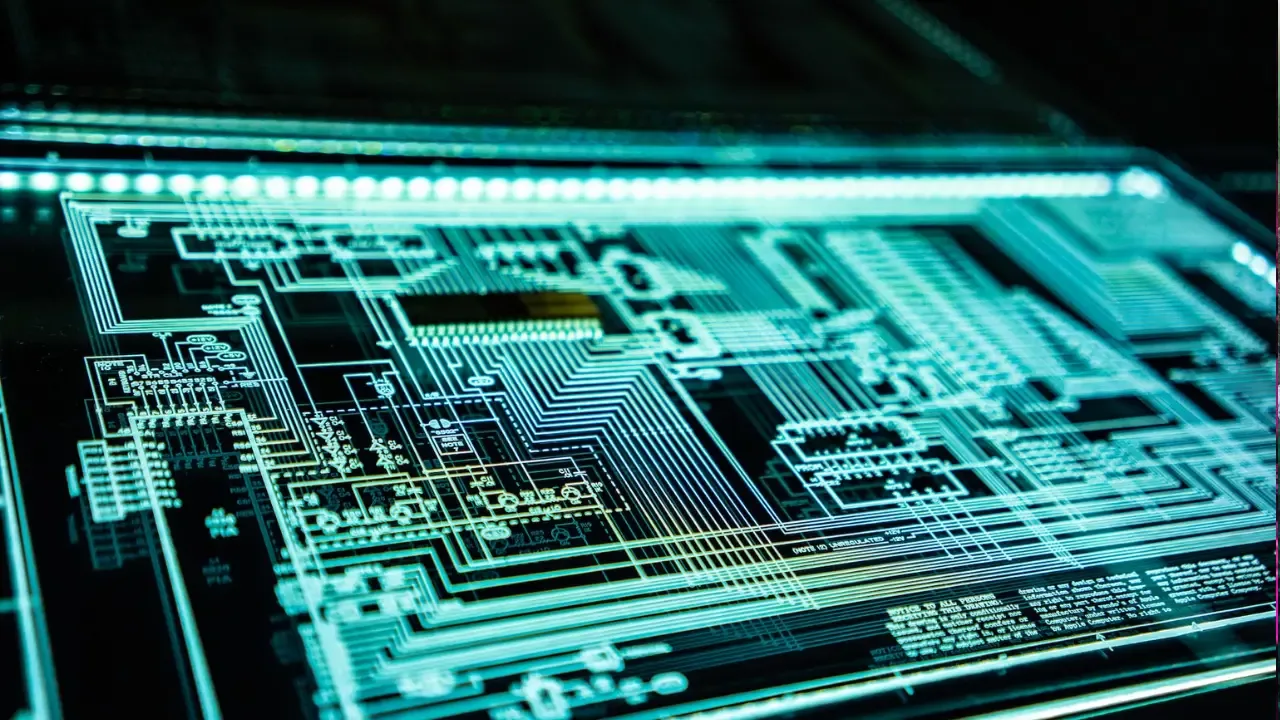
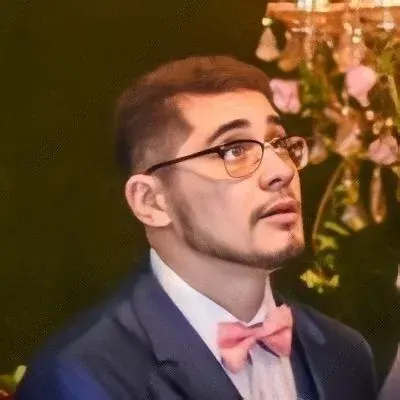
📂 Uploading Files in MVC 4 Razor: Common Issues and Easy Solutions 🚀
So, you're new to MVC 4 and you're trying to implement a file upload control in your website? 👀 Don't worry, we've got you covered! In this blog post, we'll address the common issue of getting a null value in your file during the upload process. Let's dive in and find a solution! 💪
The Controller
First, let's take a look at your controller code. It seems you have a controller named UploadController
. Within that controller, you have an action method named UploadDocument()
that returns a view. This is the action responsible for rendering the upload form.
Now, let's focus on the Upload()
action method. This method is decorated with the [HttpPost]
attribute, indicating that it will handle the form submission. It accepts a parameter of type HttpPostedFileBase
named file
, which represents the uploaded file.
public class UploadController : BaseController
{
public ActionResult UploadDocument()
{
return View();
}
[HttpPost]
public ActionResult Upload(HttpPostedFileBase file)
{
if (file != null && file.ContentLength > 0)
{
var fileName = Path.GetFileName(file.FileName);
var path = Path.Combine(Server.MapPath("~/Images/"), fileName);
file.SaveAs(path);
}
return RedirectToAction("UploadDocument");
}
}
The View
Moving on to the view. The view contains a form that uploads the file. The form is created using the Html.BeginForm()
method and specifies the action and controller where the form data will be submitted (Upload
action in Upload
controller). Additionally, we need to set the enctype
attribute of the form to multipart/form-data
so that it can handle file uploads.
@using (Html.BeginForm("Upload", "Upload", FormMethod.Post, new { enctype = "multipart/form-data" }))
{
<input type="file" name="FileUpload" />
<input type="submit" name="Submit" id="Submit" value="Upload" />
}
The Issue: Getting a Null Value
Now, let's address the issue you mentioned - getting a null value in the file
parameter of the Upload()
action. One possible reason for this issue is the mismatch in the name attribute of the file input element in the view and the parameter name in the action method.
Currently, the file input element in the view has the name attribute set as FileUpload
. However, in the controller action, the parameter name is file
. These names should match for the framework to bind the uploaded file to the parameter.
The Solution: Matching the Names
To fix the issue, let's ensure that the names match in both the view and the controller. Update the name attribute of the file input element in the view to match the parameter name in the action method (file
).
Here's the updated code snippet for the view:
@using (Html.BeginForm("Upload", "Upload", FormMethod.Post, new { enctype = "multipart/form-data" }))
{
<input type="file" name="file" /> <!-- Updated name from "FileUpload" to "file" -->
<input type="submit" name="Submit" id="Submit" value="Upload" />
}
With this change, the framework will bind the uploaded file to the file
parameter in the Upload()
action method, and you'll no longer encounter the issue of getting a null value.
Your Turn! 📢
Now that we've resolved the file upload issue, it's time for you to try implementing it in your website. Follow the steps we discussed, and you'll be able to upload files successfully using the MVC 4 Razor. Feel free to share your experiences or any further questions in the comments section below!
Happy file uploading! 🎉📁
Don't want to miss out on future tutorials and troubleshooting guides? Join our tech-savvy community by subscribing to our newsletter and stay up-to-date with the latest tips and tricks. Never stop learning! 🙌💡
Subscribe to our Newsletter 💌
To receive more helpful resources like this straight to your inbox, subscribe to our newsletter. Don't miss out on the latest tech trends, tutorials, and pro tips! Simply enter your email below and hit "Subscribe."
Enter your email address... 📧
[Subscribe Button]
Follow us on social media for more exciting content:
Twitter: [Twitter Handle]
Facebook: [Facebook Page]
Instagram: [Instagram Handle]
Together, let's master the world of tech! 🌍💻