Multiple models in a view
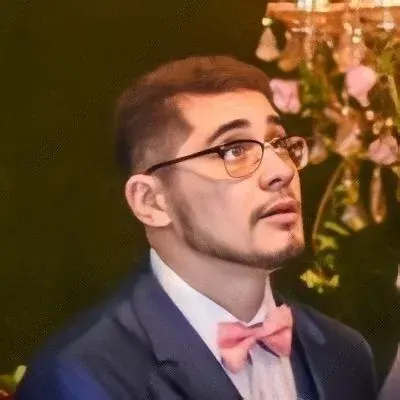
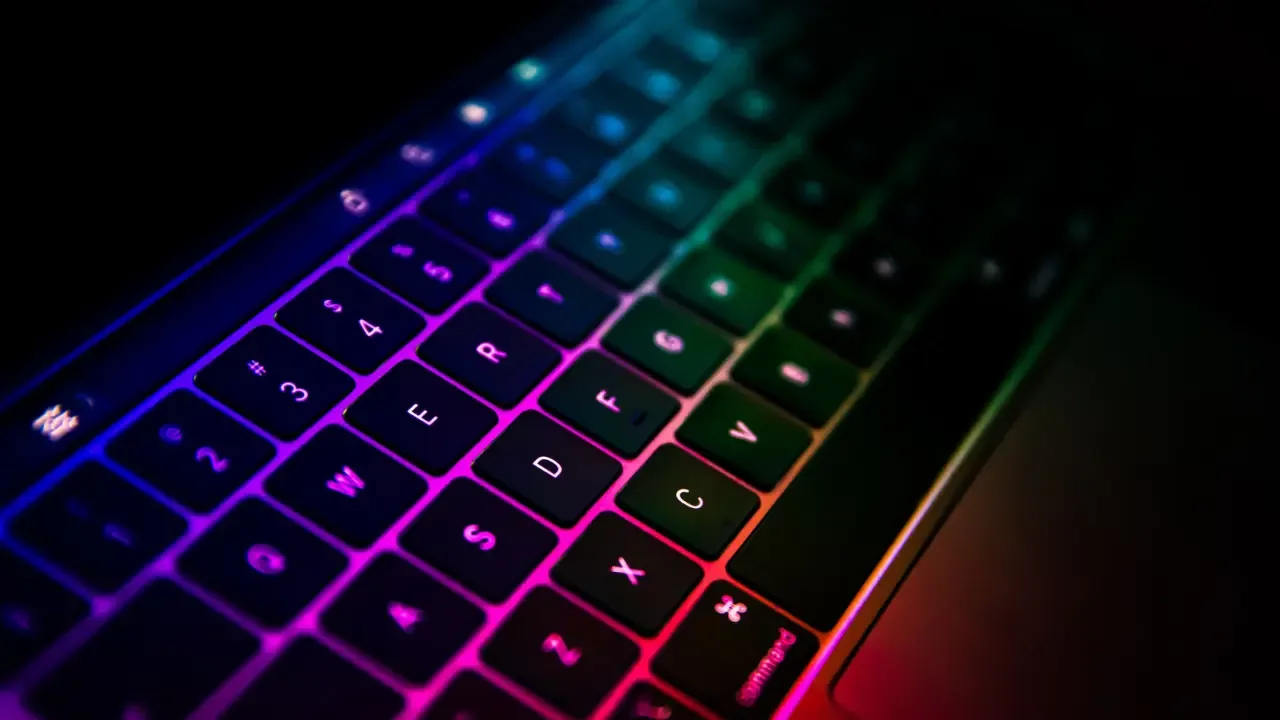
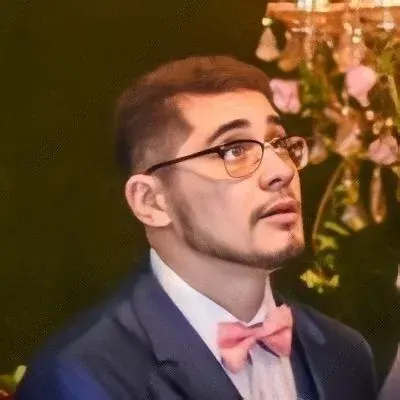
📝 Multiple Models in a View: How to Handle Common Issues and Find Easy Solutions
Are you struggling with including multiple models in a single view? Do you find yourself in a situation where you need to display different models in the same page, such as a combination of a LoginViewModel
and a RegisterViewModel
? Don't worry, we've got you covered!
The Dilemma
So, you have two separate models - LoginViewModel
and RegisterViewModel
- and you want to display them on the same page. The first thought that might come to your mind is creating a BigViewModel
that holds both LoginViewModel
and RegisterViewModel
. This would be a valid approach, but before you go down that path, let's explore an alternative solution.
The Alternative Approach
Instead of creating a BigViewModel
, you can directly use the individual view models in the view itself. This approach eliminates the need for an additional layer of abstraction and simplifies your code. Here's how you can implement it:
@model ViewModel.RegisterViewModel
@using (Html.BeginForm("Login", "Auth", FormMethod.Post))
{
@Html.TextBoxFor(model => model.Name)
@Html.TextBoxFor(model => model.Email)
@Html.PasswordFor(model => model.Password)
}
@model ViewModel.LoginViewModel
@using (Html.BeginForm("Login", "Auth", FormMethod.Post))
{
@Html.TextBoxFor(model => model.Email)
@Html.PasswordFor(model => model.Password)
}
Addressing Validation Attributes
You mentioned that you need the validation attributes to be applied to the view. The good news is that this alternative approach also retains the ability to apply validation attributes. Simply add the required validation attributes in your view models as you would normally do. The TextBoxFor
and PasswordFor
helper methods will automatically render the appropriate HTML with the validation attributes intact.
Why Choose the Alternative?
The main advantage of the alternative approach is simplicity. By directly using the individual view models, you avoid unnecessary complexity and keep your code clean. There's no need to create a separate view model (e.g., BigViewModel
) just to hold other view models.
Call-to-Action: Engage and Share Your Thoughts
We hope this article has provided you with a clear understanding of how to handle multiple models in a view. Now it's your turn to take action! Have you encountered this issue before? What approach did you take? Share your thoughts and experiences in the comments below. Don't forget to hit the share button to help fellow developers dealing with the same problem!
Happy coding! 💻🚀