jQuery Ajax calls and the Html.AntiForgeryToken()
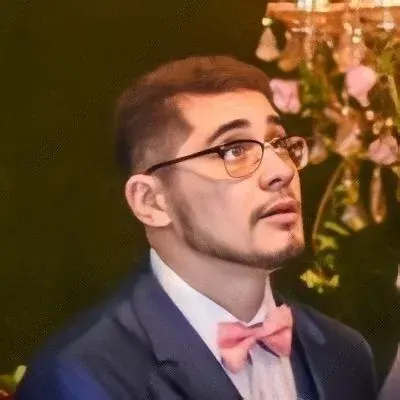
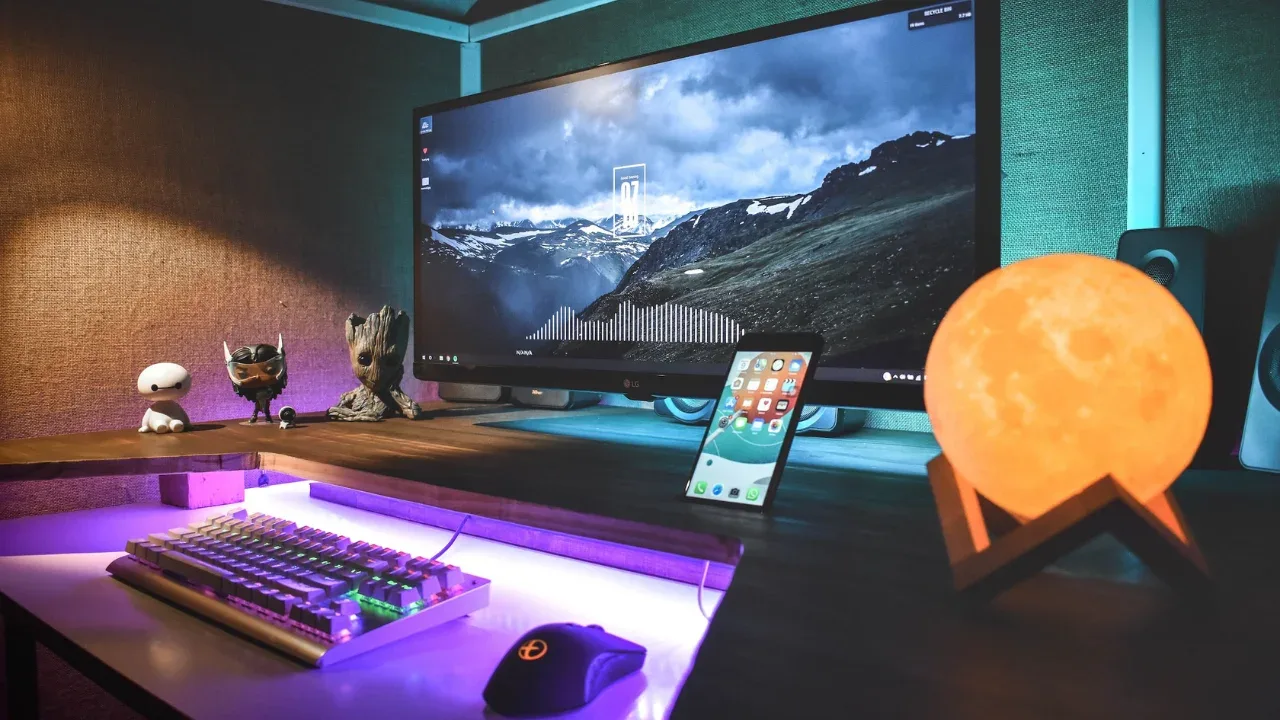
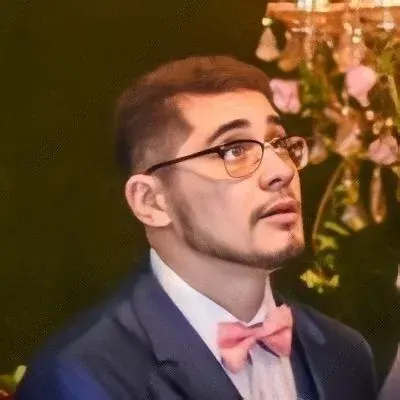
🤔 How to use Html.AntiForgeryToken() in jQuery Ajax calls?
If you've implemented CSRF (Cross-Site Request Forgery) attack mitigation in your app, you might be wondering how to use the Html.AntiForgeryToken()
helper in jQuery Ajax calls where there's no form involved. Let's dive in!
🛡️ Understanding CSRF Attacks
CSRF attacks occur when an unauthorized website tricks a user's browser into performing actions on a different website without their knowledge or consent. Implementing measures like ValidateAntiForgeryToken
can prevent these attacks by confirming that the request originated from the expected source.
💡 The Solution: Adding AntiForgeryToken to AJAX Calls
To use Html.AntiForgeryToken()
in jQuery Ajax calls, you need to include the anti-forgery token in the data parameter of your Ajax request.
Here's an example of how to modify your code to achieve this:
$("a.markAsDone").click(function (event) {
event.preventDefault();
var token = $('input[name="__RequestVerificationToken"]').val(); // Get the anti-forgery token from the form
$.ajax({
type: "post",
dataType: "html",
url: $(this).attr("rel"),
data: {
__RequestVerificationToken: token, // Include the anti-forgery token in the data
id: parseInt($(this).attr("title"))
},
success: function (response) {
// ....
}
});
});
In the example above, we fetch the anti-forgery token from the form before making the Ajax request. Then, we include the token in the data
parameter as __RequestVerificationToken
. This ensures that the request includes the necessary validation token, preventing CSRF attacks.
🚀 Take Action: Protect Your App!
With the modified code, your jQuery Ajax calls will now include the anti-forgery token, ensuring that each request is validated and protected against CSRF attacks.
Remember to add the ValidateAntiForgeryToken
attribute to your server-side actions that accept the POST Http verb to complete the implementation and maximize security.
Stay ahead of potential threats and protect your app — implement CSRF mitigation today!
Have you encountered any issues with jQuery Ajax calls and Html.AntiForgeryToken()
? Share your experiences and let's discuss in the comments below! 😄