Is it possible to make an ASP.NET MVC route based on a subdomain?
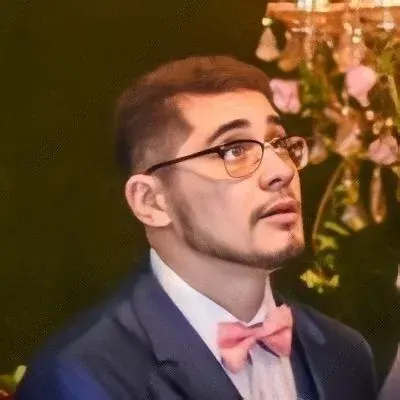
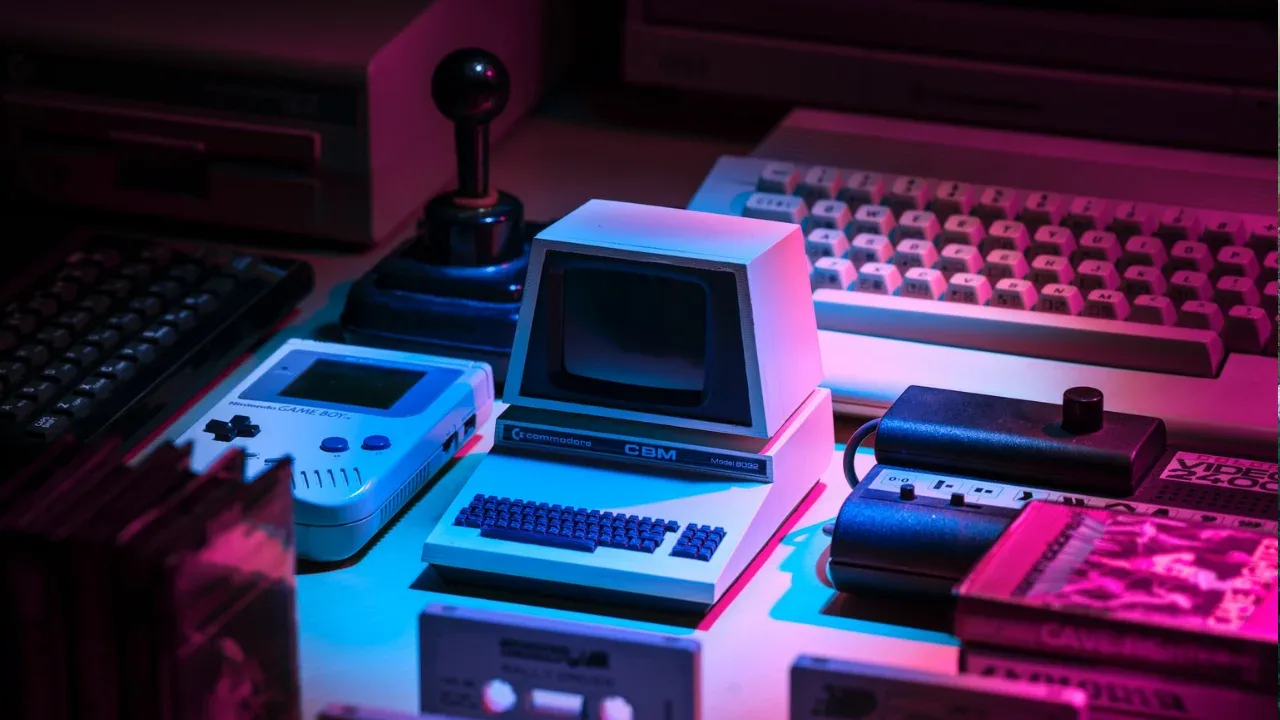
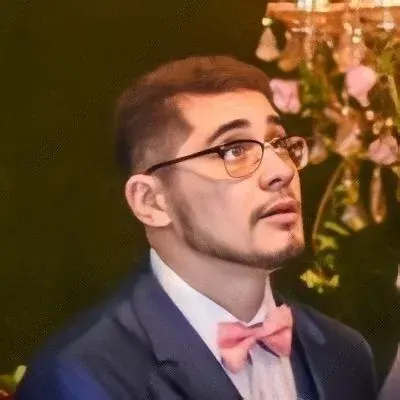
🚀 Making ASP.NET MVC Routes Based on Subdomains
Are you tired of dealing with complex routing configurations in your ASP.NET MVC project? Do you want to create routes based on subdomains, allowing different subdomains to point to different actions or even use a shared action with a username parameter? Look no further! We've got you covered with this comprehensive guide!
Why Subdomain Routing?
Subdomain routing can be a powerful tool to create a user-friendly and intuitive website structure. By using subdomains, you can categorize content or provide personalized experiences for different users. For instance, you might want to direct users to different pages or actions based on their username or role.
The Challenge
Traditionally, ASP.NET MVC routing is based on the path part of the URL. By default, the hostname or subdomain is not considered when determining the route. However, with a little bit of customization, you can easily achieve the desired behavior.
The Solution - Custom Route Constraint
To make ASP.NET MVC routes based on subdomains, we need to create a custom route constraint. The custom constraint will extract the subdomain from the URL and map it to the appropriate action or parameter. Here's how you can do it:
Create a new class, let's call it
SubdomainRouteConstraint
, and implement theIRouteConstraint
interface.
public class SubdomainRouteConstraint : IRouteConstraint
{
public bool Match(HttpContextBase httpContext, Route route, string parameterName, RouteValueDictionary values, RouteDirection routeDirection)
{
string subdomain = httpContext.Request.Url.Host.Split('.')[0];
// Implement your matching logic here
// Return true if the subdomain matches the expected value(s)
// Return false if it doesn't match
}
}
In the
Match
method of theSubdomainRouteConstraint
class, extract the subdomain from theHttpContext
by splitting theUrl.Host
property. You can choose how you want to extract the subdomain based on your specific requirements.Implement your own logic inside the
Match
method to determine whether the subdomain matches the desired value(s). You can use regular expressions, dictionary lookups, or any other approach that suits your needs.Return
true
from theMatch
method if the subdomain matches the expected value(s), andfalse
otherwise.In your route configuration, add a constraint for the desired route parameter, using the
SubdomainRouteConstraint
class.
routes.MapRoute(
"SubdomainRoute",
"{controller}/{action}/{username}",
new { controller = "Home", action = "Index", username = UrlParameter.Optional },
new { username = new SubdomainRouteConstraint() }
);
In this example, we define a route with the pattern /{controller}/{action}/{username}
. The SubdomainRouteConstraint
is used specifically for the username
parameter. Any requests that match this route with a valid subdomain will be handled accordingly.
Examples
Let's take a look at some examples to see the subdomain routing in action.
Example 1: Routing to Different Actions
Suppose we have two subdomains, user1.domain.example
and user2.domain.example
. We want each of these subdomains to go to different actions on our UserController
, such as Profile
and Settings
, respectively.
To achieve this, we can modify our route configuration as follows:
routes.MapRoute(
"SubdomainRoute",
"{controller}/{action}/{username}",
new { controller = "User", action = "Profile", username = UrlParameter.Optional },
new { username = new SubdomainRouteConstraint() }
);
Now, navigating to user1.domain.example
will invoke the User
controller's Profile
action, while user2.domain.example
will invoke the Settings
action.
Example 2: Routing to the Same Action with a
username
Parameter
If we want to use a shared action that takes a username
parameter for all subdomains, we can modify our route configuration as follows:
routes.MapRoute(
"SubdomainRoute",
"{controller}/{action}/{username}",
new { controller = "Common", action = "Index", username = UrlParameter.Optional },
new { username = new SubdomainRouteConstraint() }
);
Now, both user1.domain.example
and user2.domain.example
will invoke the Index
action of the Common
controller, with the username
parameter set accordingly.
Conclusion
By creating a custom route constraint in ASP.NET MVC, you can easily implement subdomain routing. Whether you want to direct users to different actions or use a shared action with a parameter, this guide has provided you with the knowledge to achieve it.
Ready to take your routing game to the next level? Start exploring the possibilities of subdomain routing in your ASP.NET MVC projects today! 💪🎉
If you have any questions or need further assistance, feel free to leave a comment below. Happy coding! 😊👩💻👨💻