How to return a 200 HTTP Status Code from ASP.NET MVC 3 controller
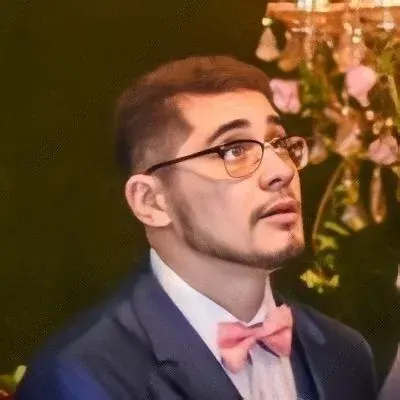
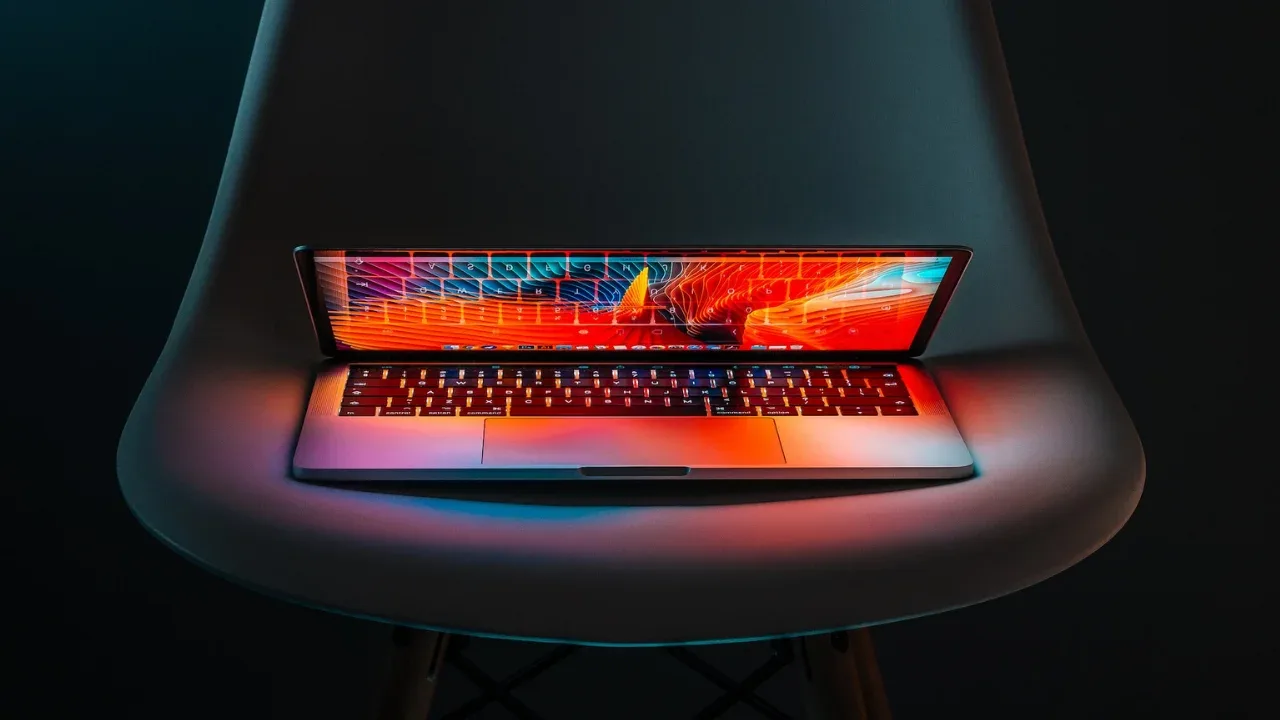
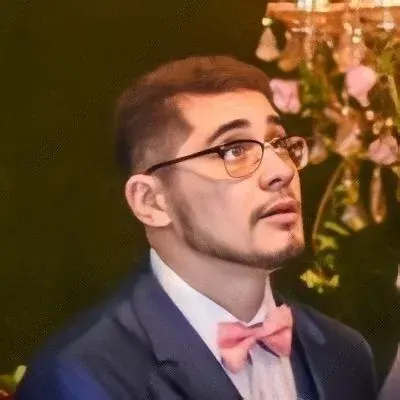
🌟 Returning a 200 HTTP Status Code from your ASP.NET MVC 3 Controller 🌟
So, you're building an amazing application that receives POST data from a third-party service, and you need to return a 200 HTTP Status Code 🤔. No worries, I'm here to guide you through!
The Power of HTTP Status Codes 🚀
HTTP Status Codes are like secret handshakes between your application and the web browser. They communicate the status of your web request, allowing you to handle errors or provide success messages. In this case, we want to communicate to the third-party service that everything went swimmingly well 👌.
Let's Get Our Hands Dirty 💪
To return a 200 HTTP Status Code from your ASP.NET MVC 3 Controller, you can follow these simple steps:
Inside your controller action method, add the following line of code:
return new HttpStatusCodeResult(200);
Voilà! You have successfully returned a 200 HTTP Status Code 🎉.
Here's an example code snippet of how it would look like:
public class MyController : Controller
{
public ActionResult MyAction()
{
// Your code logic goes here
// Return a 200 HTTP Status Code
return new HttpStatusCodeResult(200);
}
}
Common Pitfalls to Avoid 🚫
While implementing this, here are a couple of things to keep in mind:
Make sure you are using the correct namespace.
HttpStatusCodeResult
should be available under theSystem.Web.Mvc
namespace.Be cautious with the use of different HTTP Status Codes. Returning a 200 implies success, while other codes might represent different scenarios like errors or redirects. Be sure to use the appropriate code for each situation.
Engage with the Community 🤝
Have you encountered any issues with returning a 200 HTTP Status Code from your ASP.NET MVC 3 Controller? Or do you have a different approach? Share your thoughts and experiences in the comments section below! Let's help each other out and make coding even more awesome! 💻💬
Conclusion 🎉
Returning a 200 HTTP Status Code from your ASP.NET MVC 3 Controller is crucial when interacting with third-party services. By following these easy steps, you can ensure smooth communication and avoid any confusion. Remember, a well-designed application should always provide the right HTTP Status Codes.
Now, go ahead and implement this in your codebase. Happy coding! 🚀💻
P.S. Don't forget to share this article with your fellow developers who might find it helpful. Sharing is caring! ❤️