How to render an ASP.NET MVC view as a string?
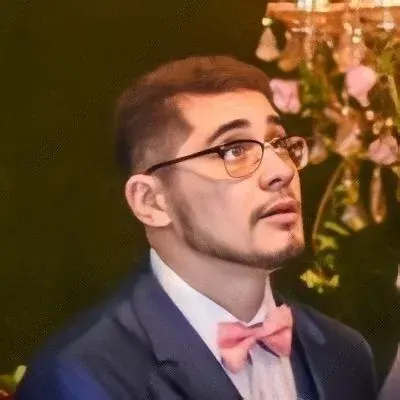
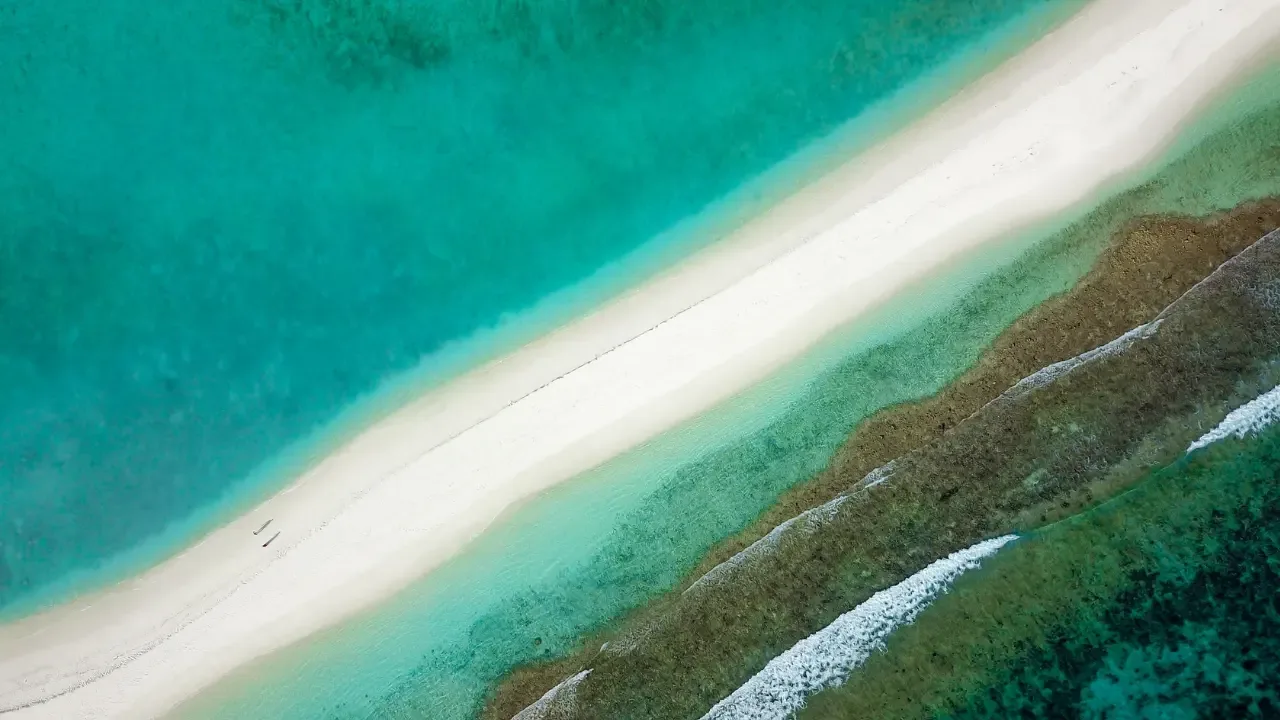
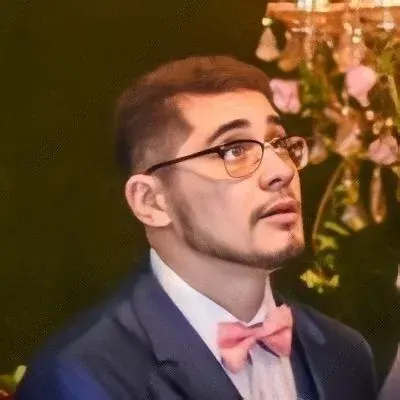
How to Render an ASP.NET MVC View as a String 💻😄
Are you looking for a way to output an ASP.NET MVC view as a string? 🤔 Perhaps you want to send the view as an email or perform some other custom action. In this blog post, we'll explore how you can accomplish this in a simple and efficient manner. 💪🚀
The Problem: Rendering a View as a String 😮
A common question in ASP.NET MVC is how to render a view as a string. The traditional approach of rendering views directly to the response stream doesn't allow us to capture the rendered output as a string. 😞
Common Solutions that Don't Quite Work 😕
Two popular solutions are often suggested, but they have their downsides:
RenderPartial to String in ASP.NET MVC Beta 🔄
This approach involves using the
RenderPartial
method to render a partial view. However, it can lead to the error "Cannot redirect after HTTP headers have been sent." Not ideal! 😓Example Source: RenderPartial to String in ASP.NET MVC Beta
MVC Framework: Capturing the output of a view ➡️
This technique captures the output of a view, but it may cause issues when trying to perform a
redirectToAction
or if the rendered view doesn't look right. 🤷♂️Example Source: MVC Framework: Capturing the output of a view
While these solutions may work in some cases, they're not reliable or convenient enough to use in our scenario. We need a better approach! 😎
The Solution: RenderViewToString Method ✨🎉
To render an ASP.NET MVC view as a string, we can define a RenderViewToString
method, which captures the output of a view and returns it as a string. Let's take a look at the implementation:
public virtual string RenderViewToString(
ControllerContext controllerContext,
string viewPath,
string masterPath,
ViewDataDictionary viewData,
TempDataDictionary tempData)
{
Stream filter = null;
ViewPage viewPage = new ViewPage();
// Create our view
viewPage.ViewContext = new ViewContext(controllerContext, new WebFormView(viewPath, masterPath), viewData, tempData);
// Get the response context, flush it, and get the response filter
var response = viewPage.ViewContext.HttpContext.Response;
response.Flush();
var oldFilter = response.Filter;
try
{
// Put a new filter into the response
filter = new MemoryStream();
response.Filter = filter;
// Render the view into the MemoryStream and flush the response
viewPage.ViewContext.View.Render(viewPage.ViewContext, viewPage.ViewContext.HttpContext.Response.Output);
response.Flush();
// Read the rendered view from the MemoryStream
filter.Position = 0;
var reader = new StreamReader(filter, response.ContentEncoding);
return reader.ReadToEnd();
}
finally
{
// Clean up
if (filter != null)
{
filter.Dispose();
}
// Replace the response filter
response.Filter = oldFilter;
}
}
Impressive, isn't it? This method encapsulates the logic to render a view and capture the output. With this approach, we can effortlessly obtain the rendered view as a string! 🎉
Example Usage 🌟
Let's see how we would use the RenderViewToString
method in practice. Imagine we want to generate an order confirmation email and pass the Site.Master
location.
string myString = RenderViewToString(
this.ControllerContext,
"~/Views/Order/OrderResultEmail.aspx",
"~/Views/Shared/Site.Master",
this.ViewData,
this.TempData);
By calling the RenderViewToString
method and providing the necessary parameters, we can generate the order confirmation email as a string. How cool is that? 😄
Your Turn! 💌
Now that you know how to render an ASP.NET MVC view as a string, it's time to put your newfound knowledge into action! 💪 Try using the RenderViewToString
method in your own projects and see the magic happen. ✨ And don't forget to share your experiences and any other cool techniques you discover with us in the comments below! Let's level up together! 🚀💡
Conclusion 🎊
In this blog post, we learned how to render an ASP.NET MVC view as a string. We explored common solutions that fall short and introduced the RenderViewToString
method as a reliable and efficient way to accomplish this task. Now it's up to you to take this knowledge and create amazing things in your ASP.NET MVC applications! 💪
Thanks for reading! If you found this blog post helpful, please share it with your fellow developers and spread the word. And don't forget to subscribe to our newsletter for more exciting tech tips and tutorials delivered straight to your inbox. Until next time, happy coding! 😄👩💻👨💻