How to mock the Request on Controller in ASP.Net MVC?
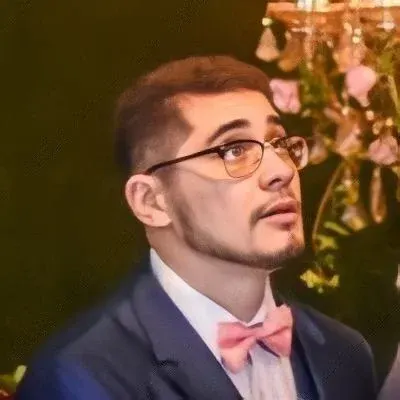

š How to mock the Request on Controller in ASP.Net MVC? šÆ
Do you have a controller in C# using the ASP.Net MVC framework and want to learn how to mock the Request object for testing purposes? We've got you covered! š
š§° The Problem: This is the code snippet of the controller:
public class HomeController : Controller
{
public ActionResult Index()
{
if (Request.IsAjaxRequest())
{
// do some ajaxy stuff
}
return View("Index");
}
}
The Request object in the controller doesn't have a setter, which makes mocking it a bit tricky. When attempting to mock the Request object using RhinoMocks or Moq, you might encounter errors.
š” The Solution: To mock the Request object successfully, we need to use Moq instead of RhinoMocks. Here's an example using Moq:
var request = new Mock<HttpRequestBase>();
request.SetupGet(x => x.Headers["X-Requested-With"]).Returns("XMLHttpRequest");
var context = new Mock<HttpContextBase>();
context.SetupGet(x => x.Request).Returns(request.Object);
var controller = new HomeController();
controller.ControllerContext = new ControllerContext(context.Object, new RouteData(), controller);
var result = controller.Index() as ViewResult;
Assert.AreEqual("About", result.ViewName);
š§° Explanation:
We create a mock of the HttpRequestBase object using Moq.
Since the IsAjaxRequest() method is a static extension method, we can't directly mock it. However, we can set the value of the "X-Requested-With" header, which is used by the IsAjaxRequest() method to determine if the request is AJAX or not.
Next, we create a mock of the HttpContextBase object using Moq and set the Request property to our mocked request object.
We then set the ControllerContext of the HomeController to our mocked HttpContextBase object.
Finally, we execute the Index() action and assert that the ViewResult's ViewName is "About" (or any expected value).
āļø Note: Make sure to include the necessary namespaces like "System.Web.Mvc" and "Moq" in your code.
š„ Take Action: Now it's time to implement this solution and successfully mock the Request object on your ASP.Net MVC Controller! Share your experience with us and let us know if it helped you. šŖš¢
#aspnetmvc #testing #moq #controller #request #mocking #csharp
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
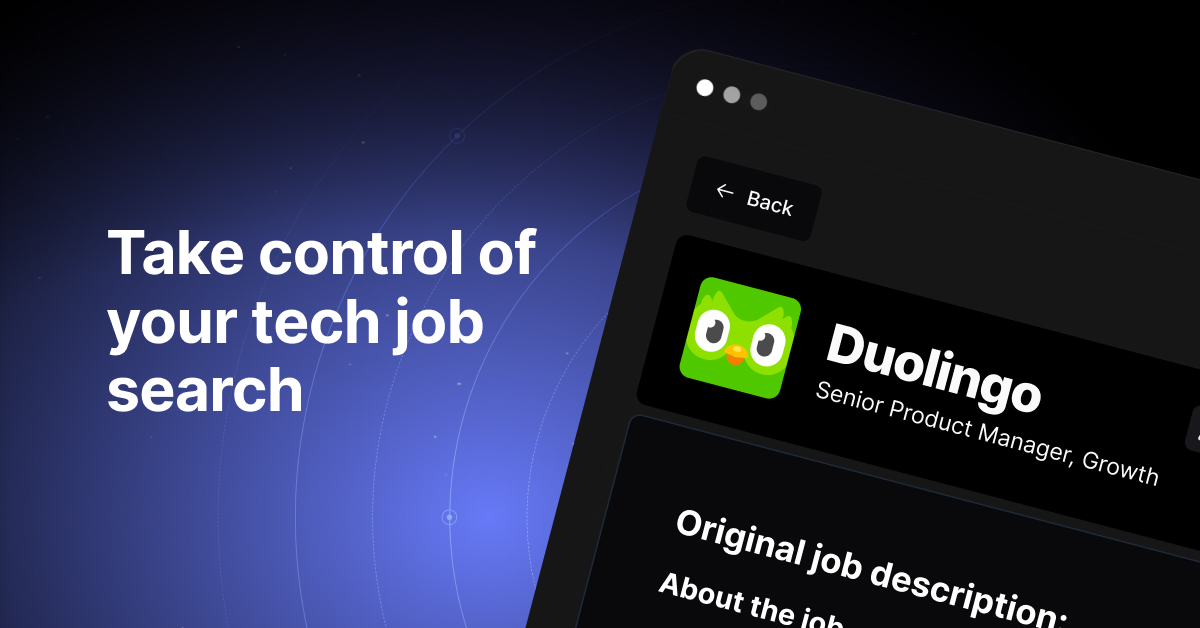