How to handle checkboxes in ASP.NET MVC forms?
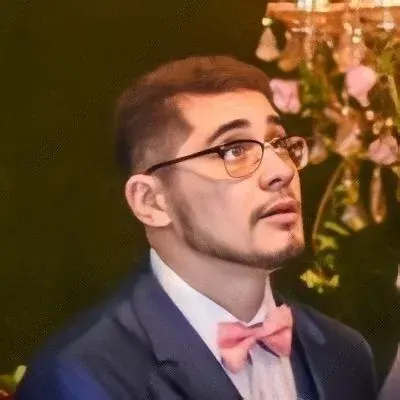
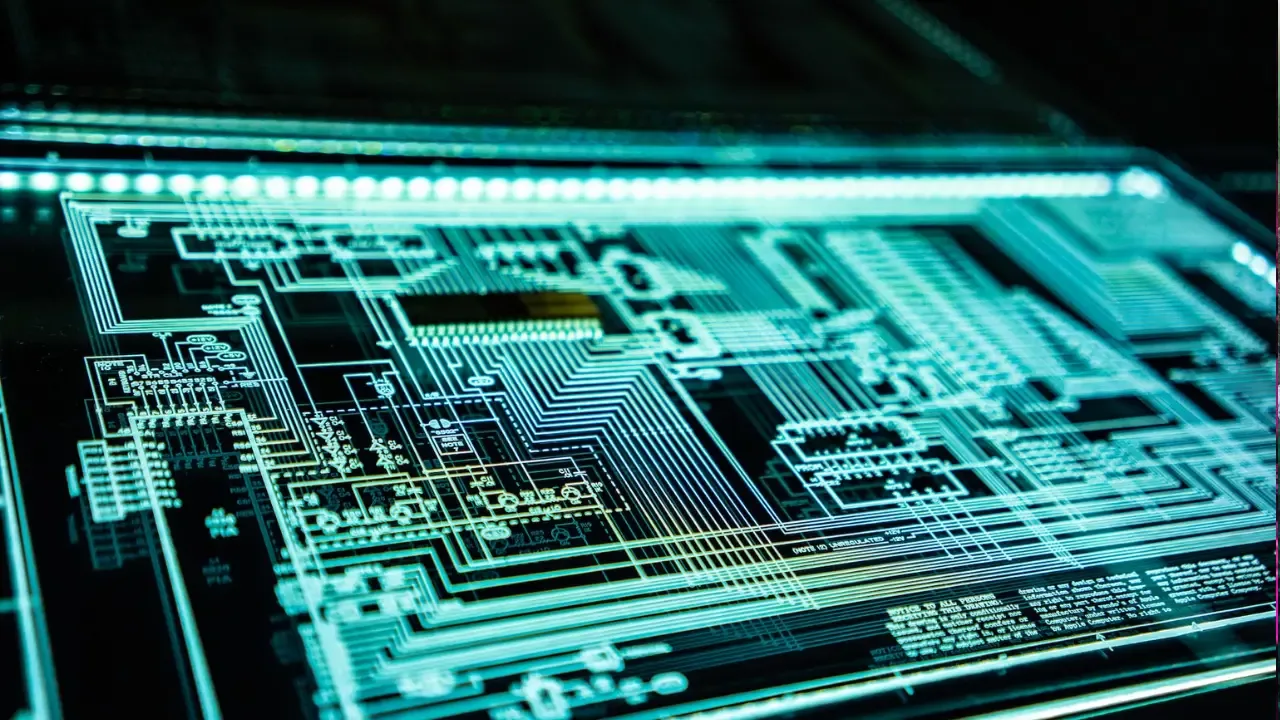
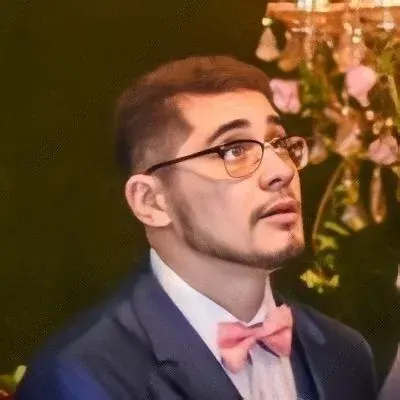
How to Handle Checkboxes in ASP.NET MVC Forms? 😕
So, you want to handle checkboxes in your ASP.NET MVC forms, huh? It can be a bit tricky, but don't worry, I've got you covered! 🙌
The Problem 😫
The user wants to select one or more objects from a collection, and you need checkboxes to accomplish that. However, your objects don't have any concept of "selected," and you're not sure how to handle the checkbox values in your controller.
The Solution 🎉
Fear not, young developer! Here's a step-by-step guide to handling checkboxes in ASP.NET MVC forms:
Step 1: Define your model 📝
In your model, create a class to represent each object in your collection. For example:
public class SampleObject{
public Guid Id { get; set; }
public string Name { get; set; }
}
Step 2: Build your view form 🖥️
In your view, use the Html.CheckBox
helper method inside a form to generate the checkboxes. Iterate over your collection and create a checkbox for each object:
@using (Html.BeginForm())
{
@foreach (var o in ViewData.Model)
{
<div>
@Html.CheckBox(o.Id.ToString())
@Html.Label(o.Id.ToString(), o.Name)
</div>
}
<input type="submit" value="Submit" />
}
Step 3: Handle the form submission in your controller 👨💻
In your controller, you can access the checkbox values through the FormCollection
parameter. However, the values are returned as "true false" instead of just "true." To get the selected objects, you can filter the FormCollection
keys based on their values:
public ActionResult HandleCheckboxSelection(FormCollection result)
{
var selectedIds = result.AllKeys.Where(key => result[key] == "true").ToList();
foreach (var id in selectedIds)
{
// Do whatever you need to do with the selected objects
}
// Handle the rest of your logic
return View();
}
Still Feeling Lost? 🤔
Handling checkboxes in ASP.NET MVC forms can be confusing, but practice makes perfect! If you're still having trouble, don't hesitate to reach out and ask for help. The tech community is always here to lend a hand! 👩💻👨💻
Remember, learning new technologies is an adventure, and solving problems like this is what makes us better developers! 🚀
So go ahead, give it a try, and let me know how it goes in the comments below! And if you have any other questions or ideas for future blog posts, I'd love to hear them! Stay curious and keep coding! 💪😎
This blog post was inspired by a Stack Overflow question: How to handle checkboxes in ASP.NET MVC forms?