How to get all Errors from ASP.Net MVC modelState?
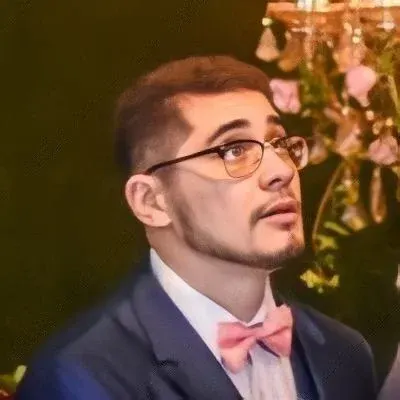
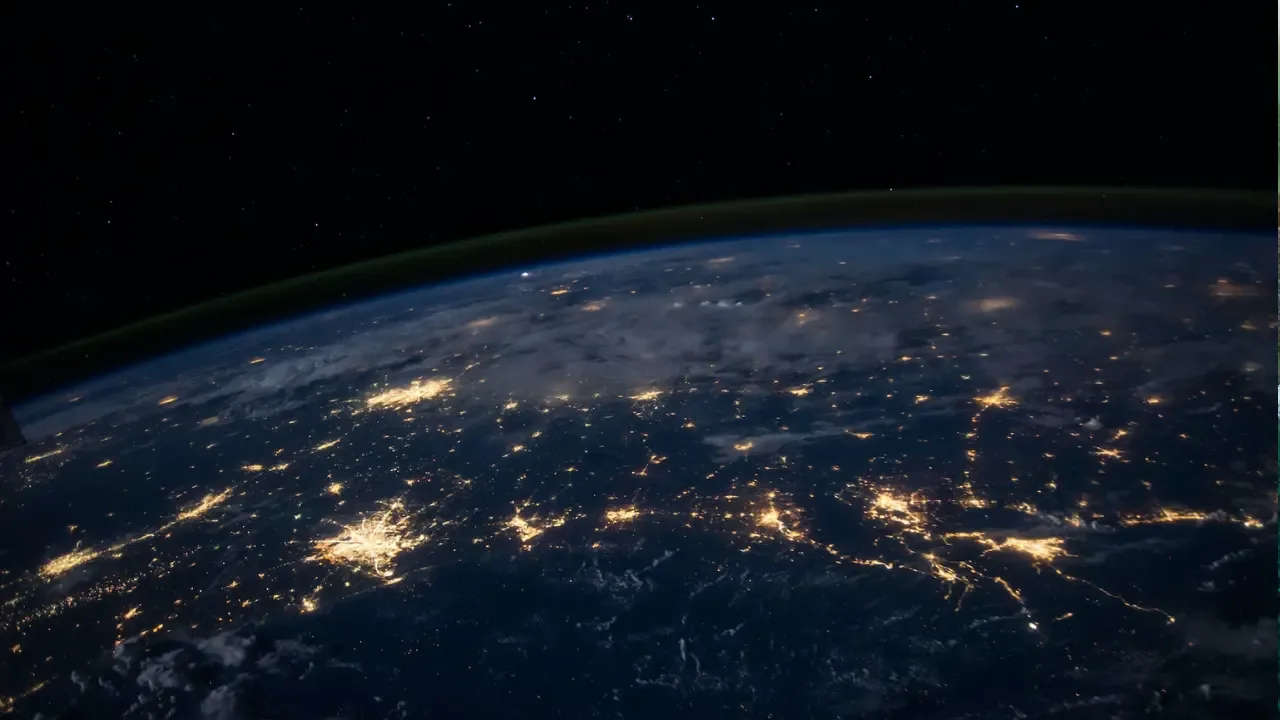
🚀 Getting All Errors from ASP.Net MVC ModelState:
Have you ever found yourself in a situation where you want to get all the error messages from the ModelState
in an ASP.Net MVC application, but you don't know the key values? Fear not, because I'm here to guide you through this challenge!
The Problem:
As mentioned in the context, the goal is to loop through the ModelState
and grab all the error messages without knowing the key values. This is a common issue developers face when they want to display or handle errors in a more generic or dynamic way.
The Solution:
Fortunately, there's a straightforward solution to overcome this problem. You can make use of the ModelState.Values
property, which represents a collection of all the ModelState
entries.
Here's the step-by-step process to retrieve all the error messages:
foreach (ModelState modelState in ViewData.ModelState.Values)
{
foreach (ModelError error in modelState.Errors)
{
string errorMessage = error.ErrorMessage;
// Do something with the error message
}
}
Let's break down what's happening in the code snippet:
We use a
foreach
loop to iterate through eachModelState
entry in theModelState.Values
collection.Inside the outer loop, we have another
foreach
loop to iterate through eachModelError
within the currentModelState
.Within the inner loop, we can access the
ErrorMessage
property of theModelError
and perform any necessary actions.
By following this approach, you can easily retrieve all the error messages stored in the ModelState
.
Example Usage:
Let's consider a scenario where you want to display all the error messages in an asp.net MVC view. You can utilize the solution discussed above to implement it.
In your controller action method, you handle a form submission, and validations occur using the ModelState
. If there are any errors, you can pass the error messages to the view.
[HttpPost]
public ActionResult Create(MyViewModel model)
{
if (!ModelState.IsValid)
{
List<string> errorMessages = new List<string>();
foreach (ModelState modelState in ViewData.ModelState.Values)
{
foreach (ModelError error in modelState.Errors)
{
string errorMessage = error.ErrorMessage;
errorMessages.Add(errorMessage);
}
}
ViewBag.ErrorMessages = errorMessages;
return View(model);
}
// Handle successful submission
return RedirectToAction("Success");
}
In the example above, we collect all the error messages in errorMessages
list. Later, we pass the list to the view using ViewBag
to display those error messages.
In the view, you can loop through ViewBag.ErrorMessages
and display the error messages as per your design preferences.
Share Your Thoughts:
I hope this guide helped you to understand how to retrieve all the error messages from the ModelState
in an ASP.Net MVC application. If you have any questions or alternative solutions to this problem, feel free to share them in the comments section below! 🤔👇
📣 Call-to-Action:
Don't forget to check out our other invaluable guides on ASP.Net MVC and stay up to date with the latest tech trends. Remember, knowledge is power! 💪✨
Happy coding! 😄💻
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
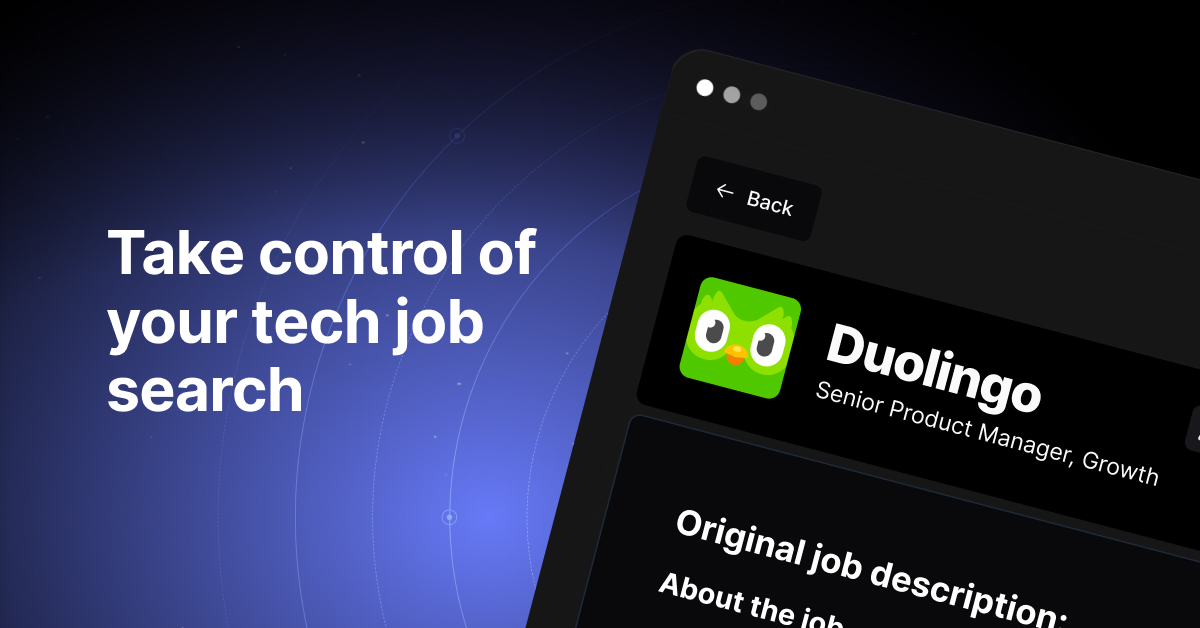