How to create a function in a cshtml template?
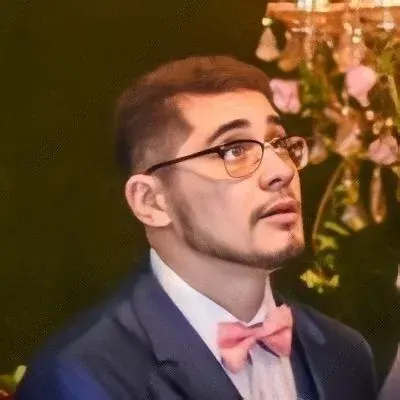
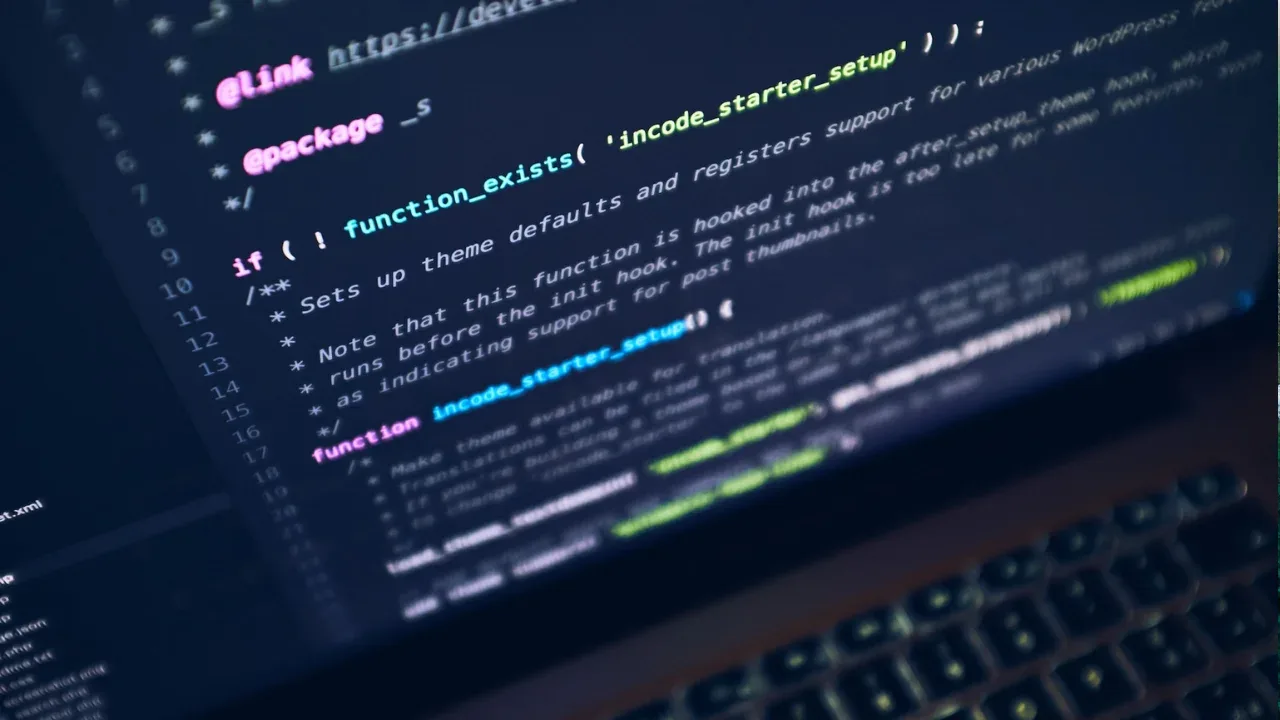
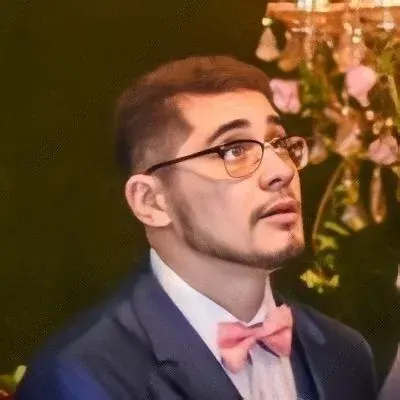
How to Create a Function in a cshtml Template? 💻🔧
So, you're working on a cshtml file and you need to create a function that is specific to that file only. Don't worry, I got you covered! In this article, I'll guide you on how to create and use a function within a cshtml template. 🎉
Understanding the Context
Before we dive into the solution, let's understand the context a bit more. In ASP.NET, cshtml files are used with the Razor template engine. These files are responsible for generating dynamic HTML and can contain server-side code mixed with HTML markup.
The Challenge
You may find yourself in a situation where you need a function that is only required within one specific cshtml file. Unlike HTML helpers or extension methods, your function needs to be scoped to one cshtml file only. But how can you achieve that? 🤔
The Solution
Don't worry, Razor has a neat feature called partial views, which can solve this problem for you. A partial view is a reusable piece of a cshtml file that can be included within other cshtml files or views.
Step 1: Create a Partial View
First, you need to create a partial view that will contain your function. To create a partial view, follow these steps:
Right-click on the directory where you want to add the partial view.
Select "Add" from the context menu and then choose "View" (or "Razor View" depending on your IDE).
Give your partial view a name and make sure to check the "Create as a partial view" option.
Click "Add" to create the partial view.
Step 2: Define Your Function
Next, open the newly created partial view and define your function inside it. You can use any valid C# code inside the partial view. For example, let's say you want to create a function named GetFormattedDate
that returns the current date in a specific format. Here's how you can do it:
@functions {
public string GetFormattedDate()
{
return DateTime.Now.ToString("MMMM dd, yyyy");
}
}
Step 3: Use the Partial View
Once you have created and defined your function in the partial view, you can now use it within your main cshtml file. To include the partial view, follow these steps:
At the location where you want to use the function, add the following line of code:
@{ await Html.RenderPartialAsync("_YourPartialViewName"); }
Replace _YourPartialViewName
with the actual name of your partial view file (excluding the file extension).
Now, you can easily use your function inside the main cshtml file by calling it like any other function:
<div>
<p>The formatted current date is: @GetFormattedDate()</p>
</div>
Step 4: Enjoy Your Function!
Congratulations! You have successfully created a function that is scoped to a single cshtml file using partial views. Now you can reuse this partial view in other cshtml files if needed.
The Call-to-Action
I hope this guide has helped you overcome the challenge of creating a function within a cshtml template. Now, it's your turn to implement and rock your cshtml files with custom functions! 🚀
If you found this guide helpful, be sure to share it with your fellow developers and leave a comment below with any questions or feedback you may have. Happy coding! 💪😊