How do I write unencoded Json to my View using Razor?
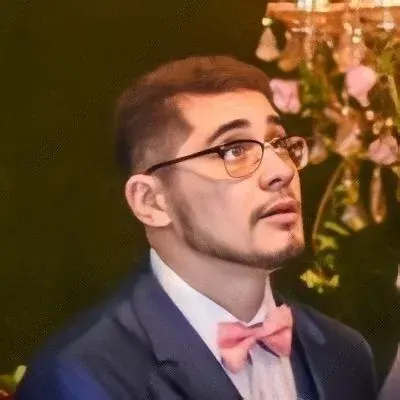
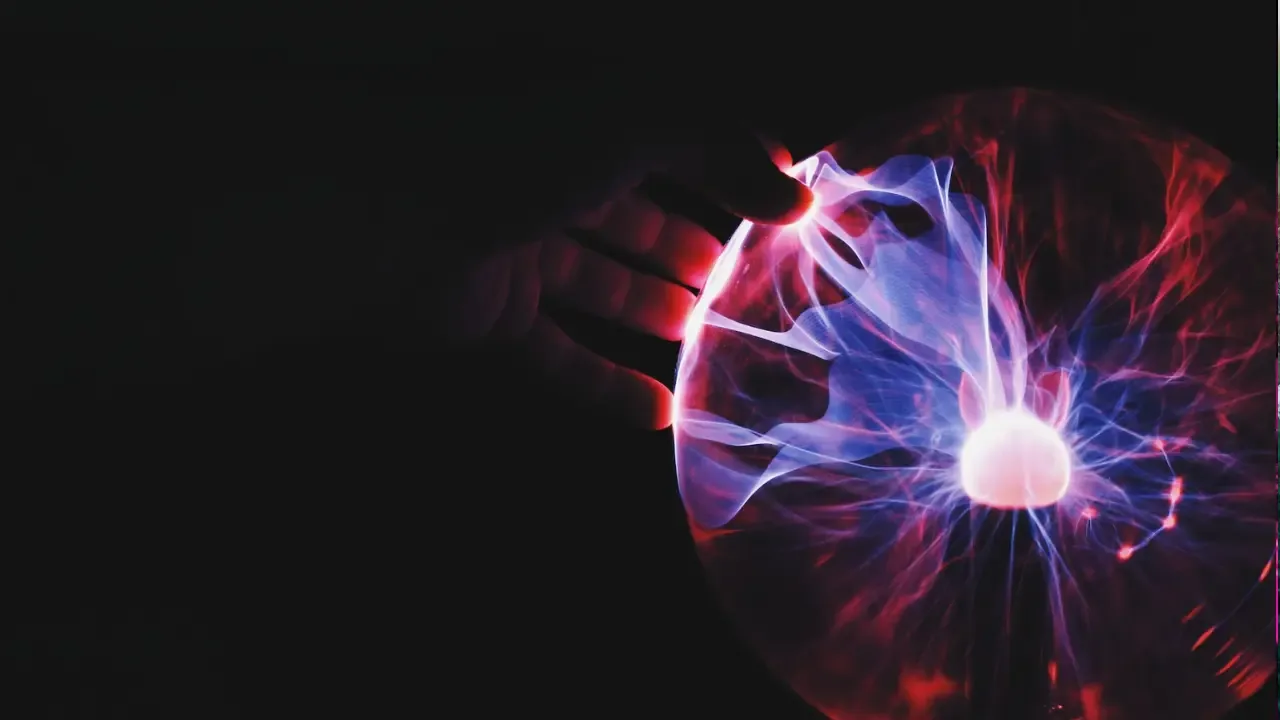
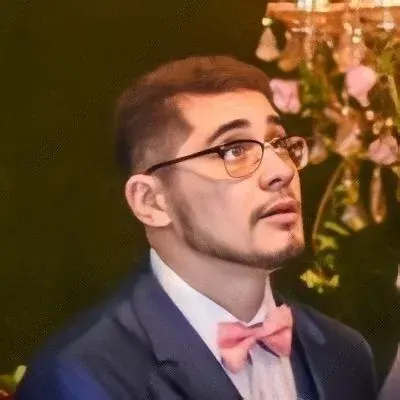
How to Write Unencoded JSON to Your View using Razor
So, you're trying to write an object as JSON to your Asp.Net MVC View using Razor, but the JSON is getting encoded in the output, causing issues for your browser. Don't worry, I've got you covered! 🙌
The Problem
Let's start by taking a look at the code you provided:
<script type="text/javascript">
var potentialAttendees = @Json.Encode(Model.PotentialAttendees);
</script>
The issue is that the JSON is being encoded, as you can see in the resulting output:
<script type="text/javascript">
var potentialAttendees = [{&quot;Name&quot;:&quot;Samuel Jack&quot;},];
</script>
This can cause problems because the browser doesn't understand the encoded characters (&quot;
in this case) and it might affect the functionality of your JavaScript code.
The Solution
To emit unencoded JSON using Razor, you can make use of the Html.Raw
helper method. It will render the JSON as-is, without any encoding.
Here's how you can update your code to achieve the desired result:
<script type="text/javascript">
var potentialAttendees = @Html.Raw(Json.Encode(Model.PotentialAttendees));
</script>
By using Html.Raw
, the JSON will be rendered without any encoding, resolving the issue and making your browser happy again! 🎉
Call-to-Action
I hope this guide helped you solve the problem of writing unencoded JSON to your View using Razor. If you found this post useful, don't forget to share it with your fellow developers so they can benefit from it too. And if you have any other tech-related questions or topics you'd like me to cover, feel free to leave a comment below. Happy coding! 💻