How do I find the absolute url of an action in ASP.NET MVC?
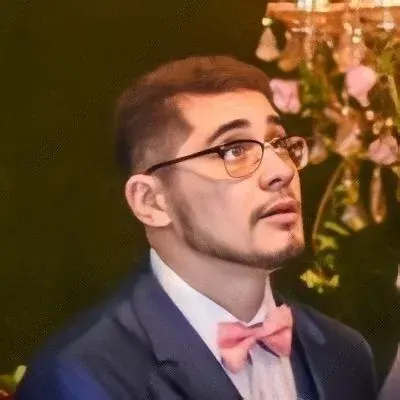
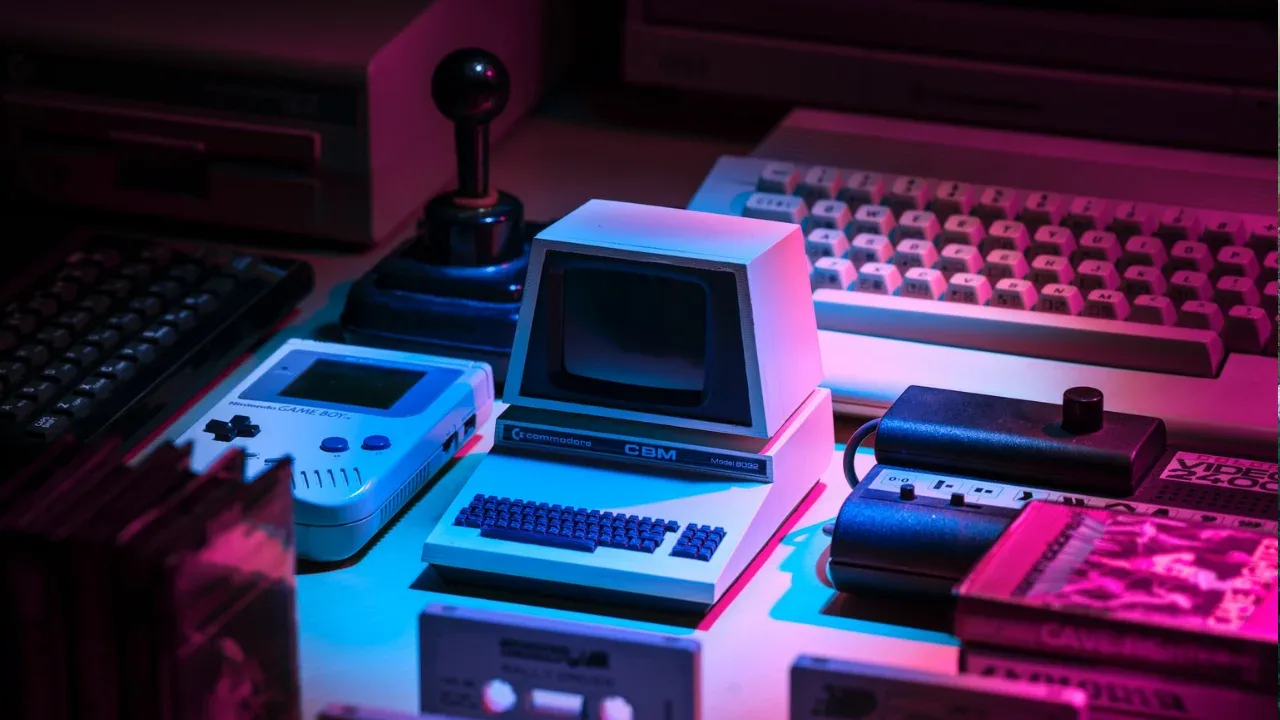
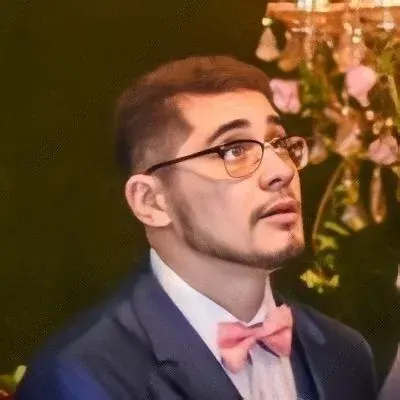
🌟📝🌐 Find the Absolute URL of an Action in ASP.NET MVC
Are you struggling to find the absolute URL of an action in ASP.NET MVC? 🤔 No worries, we've got you covered! In this blog post, we will address this common issue and provide easy solutions to help you get the absolute URL you need. 💪
Here's a common scenario: Let's say you need to specify an absolute URL for an action in your ASP.NET MVC application. You might have come across the following code snippet:
<script type="text/javascript">
token_url = "http://example.com/your_token_url";
</script>
Now, you'd like to dynamically generate the absolute URL of an action using the Url
helper, but you're not sure how to achieve it. 😕 Don't worry, we've got you covered!
Solution 1: Constructing the Absolute URL Manually
One way to get the absolute URL of an action is to construct it manually. 🏗️ You can concatenate the protocol, domain, and the relative URL of the action to form the absolute URL. Here's an example:
var baseUrl = Request.Url.Scheme + "://" + Request.Url.Authority;
var absoluteUrl = baseUrl + Url.Action("Action", "Controller");
Let's break down what's happening here:
Request.Url.Scheme
retrieves the protocol (HTTP/HTTPS) used in the current request.Request.Url.Authority
gets the domain and port number (if any) from the current request.Url.Action("Action", "Controller")
generates the relative URL for the specified action in your MVC application.
By combining these three parts, you can form the complete absolute URL. 🎉
Solution 2: Using the UrlHelper Extension Method
If you prefer a more concise approach, you can utilize an extension method on the UrlHelper
class to simplify the process. Let's take a look at how it can be done:
public static class UrlHelperExtensions
{
public static string AbsoluteAction(this UrlHelper urlHelper, string action, string controller)
{
var request = urlHelper.RequestContext.HttpContext.Request;
var baseUrl = $"{request.Url.Scheme}://{request.Url.Authority}";
return $"{baseUrl}{urlHelper.Action(action, controller)}";
}
}
To use this extension method, follow these steps:
Create a new static class (in this example,
UrlHelperExtensions
) and add the extension method.In your view or controller, import the namespace of the class you created.
Now you can use
Url.AbsoluteAction("Action", "Controller")
to get the absolute URL for your desired action.
This approach allows you to encapsulate the logic needed to construct the absolute URL into a reusable extension method. 🔄
Call-to-Action: Let's Master Absolute URLs in ASP.NET MVC! 🚀
Now that you have learned two different approaches to find the absolute URL of an action in ASP.NET MVC, you're ready to enhance your web development skills! 💪
Try implementing both solutions in your projects and see which one works best for you. Share your experience in the comments below and let us know which method you prefer! 🗣️
If you found this blog post helpful, don't forget to share it with your developer friends. Together, let's empower the developer community! 👩💻👨💻
Stay connected with us for more useful tech tips and guides. 🌐 Happy coding! 💻✨