How can I return camelCase JSON serialized by JSON.NET from ASP.NET MVC controller methods?
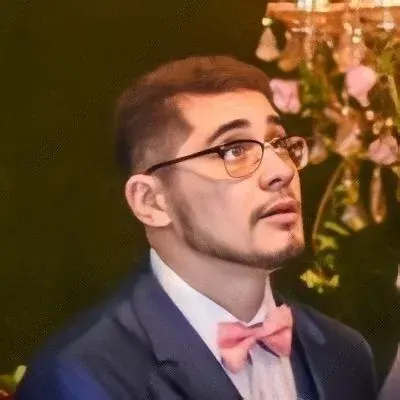
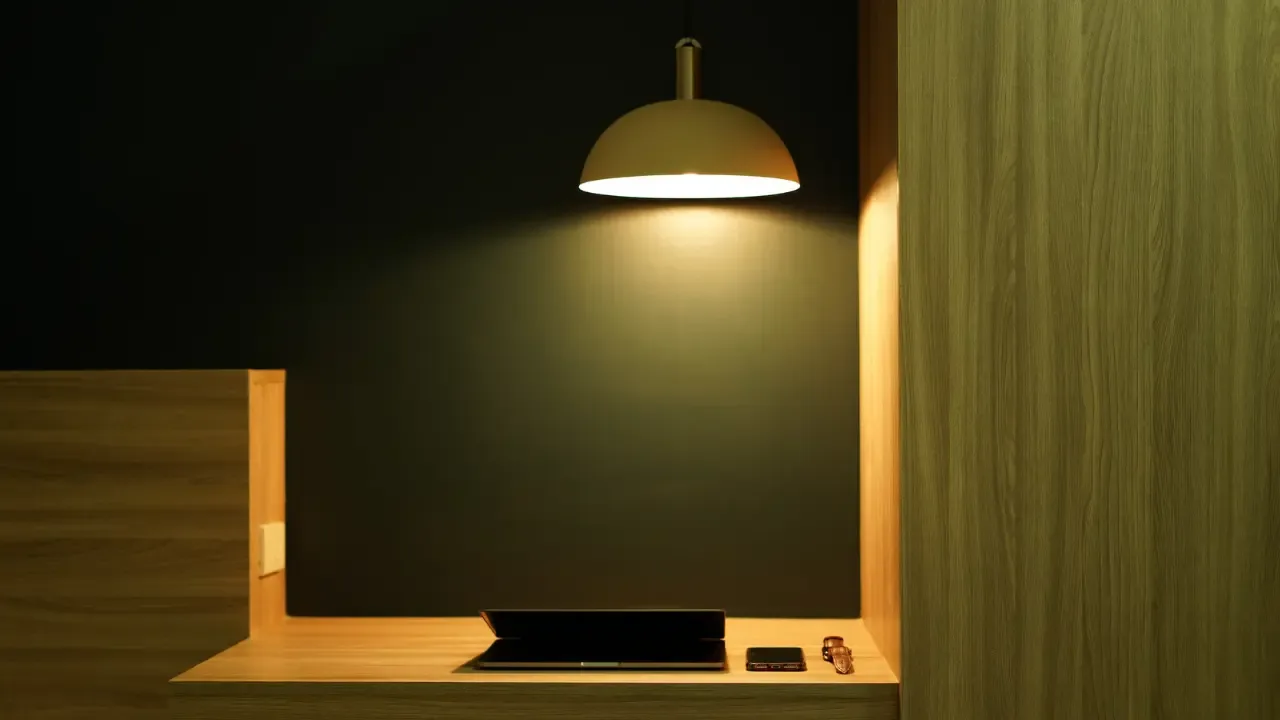
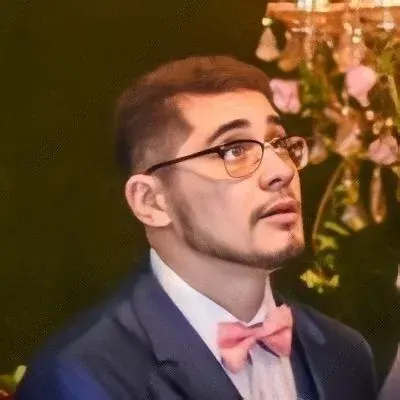
🐫 Returning camelCase JSON serialized by JSON.NET from ASP.NET MVC controller methods
If you're using ASP.NET MVC and want to return JSON data in camelCase format rather than the default PascalCase, this guide will help you solve this problem using JSON.NET.
The Issue
By default, when returning JSON data from an MVC controller using the ActionResult
class, the JSON data is serialized in PascalCase format. However, you want to serialize it in camelCase format.
The Solution
To return camelCase JSON serialized by JSON.NET, you need to customize the serialization settings of your ASP.NET MVC application.
Step 1: Install JSON.NET
If you haven't already, you need to install JSON.NET, a popular high-performance JSON framework for .NET. You can do this by downloading the package or by using NuGet Package Manager:
Install-Package Newtonsoft.Json
Step 2: Configure JSON.NET in your MVC application
In your ASP.NET MVC application, open the Global.asax.cs
file and add the following code inside the Application_Start
method:
protected void Application_Start()
{
// Other configurations
GlobalConfiguration.Configuration.Formatters.JsonFormatter.SerializerSettings.ContractResolver =
new Newtonsoft.Json.Serialization.CamelCasePropertyNamesContractResolver();
}
This code sets the ContractResolver
property of the JsonFormatter.SerializerSettings
object to an instance of the CamelCasePropertyNamesContractResolver
class. This resolver ensures that JSON object properties are serialized in camelCase format.
Step 3: Return JSON from your MVC controller
Now, you can return JSON data from your MVC controller, and it will be serialized in camelCase format.
public ActionResult GetPerson()
{
var person = new Person
{
FirstName = "Joe",
LastName = "Public"
};
return Json(person, JsonRequestBehavior.AllowGet);
}
Example
Using the above steps, the Person
object will be serialized as:
{
"firstName": "Joe",
"lastName": "Public"
}
Conclusion
By configuring JSON.NET in your ASP.NET MVC application and customizing the serialization settings, you can easily return camelCase JSON from your controller methods.
Don't forget to install JSON.NET and add the necessary code in your Global.asax.cs
file to enable camelCase serialization.
Now, go ahead and give it a try in your own application! If you have any questions or want to share your experiences, feel free to leave a comment below.
Happy coding! 🚀