How can I properly handle 404 in ASP.NET MVC?
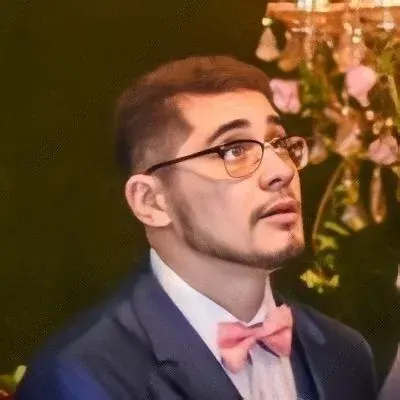
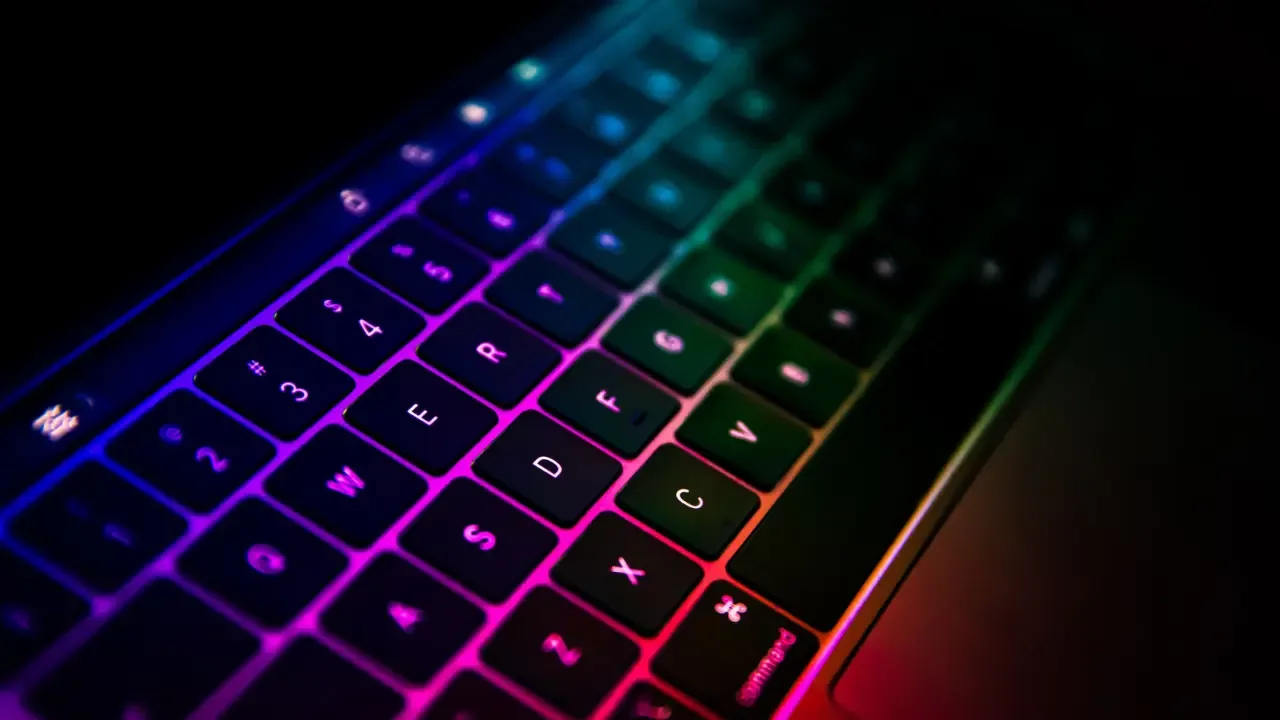
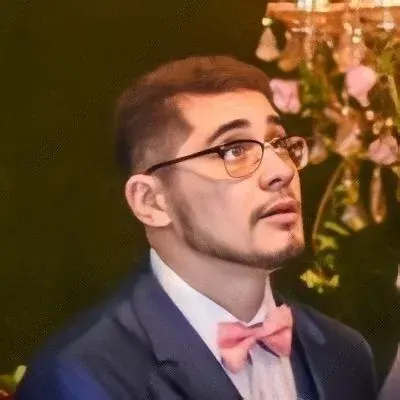
How to Properly Handle 404 in ASP.NET MVC
So, you're building an ASP.NET MVC application and want to know how to handle 404 errors like a boss. 🕶️ Don't worry, I've got you covered! In this article, I'll explain the common issues and provide easy solutions to properly handle 404 errors in ASP.NET MVC. 💪
Common Issues
Let's start by addressing the common issues faced when handling 404 errors in ASP.NET MVC. The scenarios mentioned in the question are:
Requests with unknown URLs:
The provided code snippet uses URL routing to handle these requests. 👈
Any request with an unknown URL path will be captured by the "Error" route and redirected to the "NotFound" action method in the "Errors" controller.
For example, a request for "/blah/blah/blah/blah" will be routed to the "NotFound" action method. 🛤️
Requests for unknown actions within a controller:
To handle 404 errors for unknown actions within a controller, you can override the "HandleUnknownAction" method within the controller itself. 🎮
The provided code snippet demonstrates this approach.
Any request for a non-existent action will be caught by the overridden "HandleUnknownAction" method, and a "NotFound" view will be rendered. 🚀
However, neither of these strategies handle requests for unknown or non-existent controllers.
Handling Requests for Unknown Controllers
To handle requests for unknown controllers in ASP.NET MVC, you have a few options. Here are two possible approaches:
Use a Catch-All Route:
You can add a catch-all route that captures any request for a non-existent controller and redirects it to a "NotFound" action in a dedicated "Errors" controller. 🎯
Here's an example of how you can define a catch-all route in your route configuration:
routes.MapRoute(
name: "CatchAll",
url: "{controller}/{*path}",
defaults: new { controller = "Errors", action = "NotFound" }
);
Utilize the
Application_Error
Event inGlobal.asax
:Another approach is to handle the error in the
Application_Error
event in theGlobal.asax
file. ✋In the
Application_Error
event handler, you can check if the error is a 404 (HTTP status code: Not Found).If it's a 404 error, you can redirect the user to a "NotFound" page or take any other desired action.
Choose the option that best fits your project requirements and coding style.
Should You Use Web.Config customErrors
?
The question also asks about using the customErrors
setting in the Web.config file to handle 404 errors. 🚀
While using customErrors
is a valid approach, it has some limitations. By default, the customErrors
setting redirects the user to a generic 404 page specified in the Web.config file. However, if you want to use a custom view for 404 errors, you'll need to store it outside the /Views
folder, due to the restrictions imposed by Web.config.
In contrast, using MVC-specific approaches, such as URL routing, catch-all routes, or overriding actions, allows you to have more control over the behavior and appearance of the 404 error page.
Wrapping Up
Handling 404 errors in ASP.NET MVC doesn't have to be a nightmare. By using URL routing, catch-all routes, or overriding actions, you can master the art of gracefully handling 404 errors. 🎭
Remember, it's important to choose an approach that aligns with your project's requirements and coding style. Don't forget to test your error handling logic to ensure it works flawlessly.
So, what are you waiting for? Give these techniques a try, and let me know how it goes! Share your experience and any other cool tips in the comments section below. 👇
Keep coding and keep hustling! 💻💪