Generate URL in HTML helper
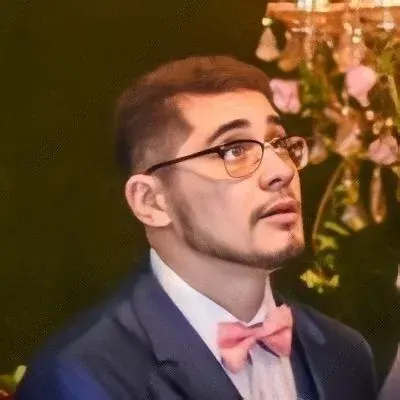
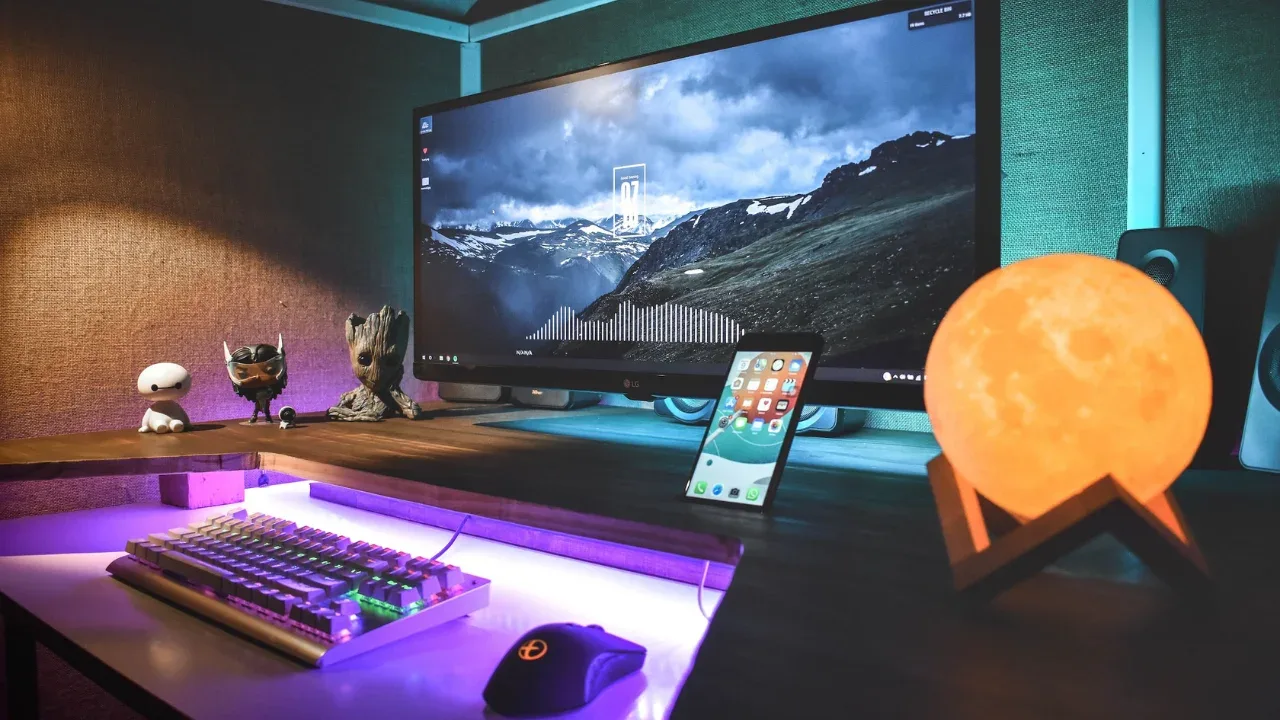
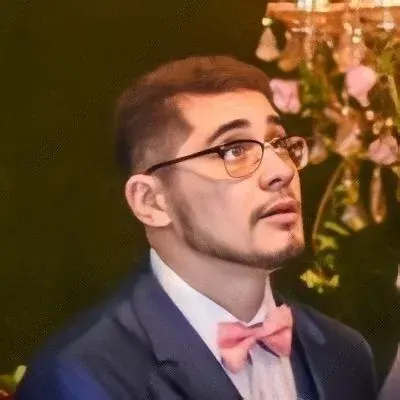
How to Generate URLs in HTML Helper in ASP.NET MVC
Are you struggling to generate URLs in a custom HTML helper in ASP.NET MVC? Don't worry, we've got you covered! In this guide, we will address this common issue and provide you with easy solutions to generate URLs in your HTML helper. Let's dive in! 💻🚀
The Problem
The problem arises when you want to generate a URL in a custom HTML helper, but the available methods only generate <a>
tags instead. You may try looking into the ASP.NET MVC source code but not find a straightforward solution. 😕
The Solution
Fortunately, there is a simple and elegant solution to generate URLs in your HTML helper without dealing with complicated internal ASP.NET stuff. 🎉
Instead of trying to create an instance of UrlHelper
yourself, you can use the HtmlHelper
instance and leverage its existing context information. Here's how you can do it:
public class MyCustomHelper
{
public static string ExtensionMethod(this HtmlHelper helper)
{
// Use the existing HtmlHelper instance to generate URLs
var url = (helper.ViewContext.Controller as Controller)?.Url;
// Generate the URL using the Action method
var generatedUrl = url?.Action("Action", "Controller");
// Return the generated URL
return generatedUrl;
}
}
By accessing the Url
property through the HtmlHelper
instance, you can generate URLs using the Action
method just like you would in a view. 🙌
An Alternative Approach
Alternatively, you can use the UrlHelper.GenerateUrl
method if you prefer a different approach. Here's an example:
public class MyCustomHelper
{
public static string ExtensionMethod(this HtmlHelper helper)
{
// Use the existing HtmlHelper instance to generate URLs
var url = (helper.ViewContext.Controller as Controller)?.Url;
// Generate the URL using the GenerateUrl method
var generatedUrl = url?.GenerateUrl(null, "Action", "Controller", null, null, null, null, null, false);
// Return the generated URL
return generatedUrl;
}
}
This method allows you to have more control over the various parameters of the URL. You can specify the route values, protocol, hostname, and more. 🛣️
Calling the HTML Helper Extension Method
Once you have implemented the HTML helper extension method, you can easily call it in your views. Here's an example of how you can use the ExtensionMethod
in a Razor view:
@{
var url = Html.ExtensionMethod();
}
You can then use the url
variable wherever you need to display or work with the generated URL. 🎯
Time to Transform Your HTML Helper!
Now that you have learned how to generate URLs in your custom HTML helper, it's time to put this knowledge into practice. 🔧 Embrace the power of ASP.NET MVC and make your HTML helper generate URLs effortlessly.
If you have any questions or suggestions, feel free to drop a comment below. Happy coding! 💪💻
Note: Remember to add appropriate comments, error handling, and any necessary modifications based on your specific requirements.