Difference between Repository and Service Layer?
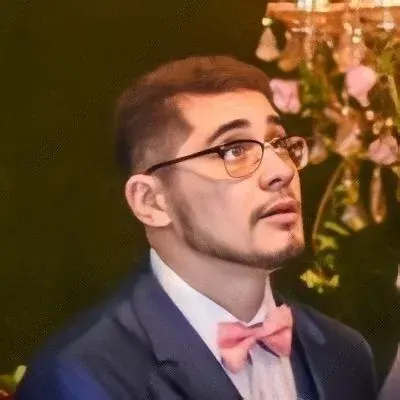
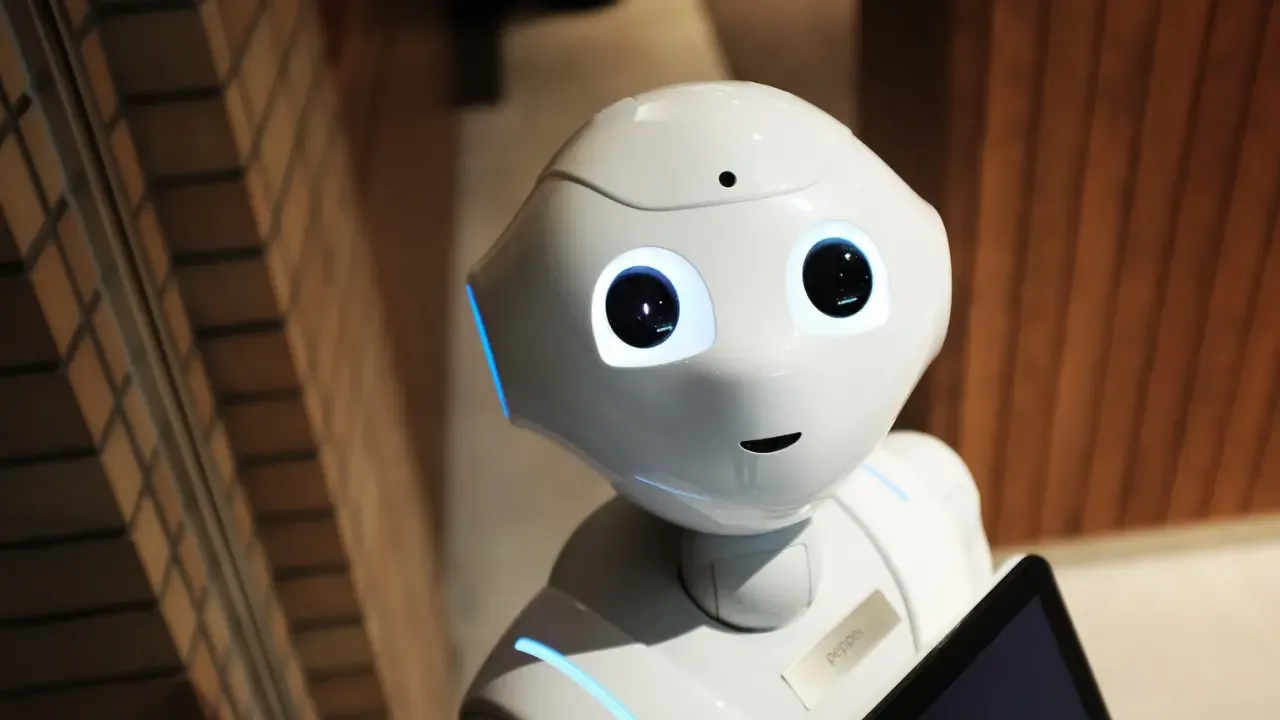
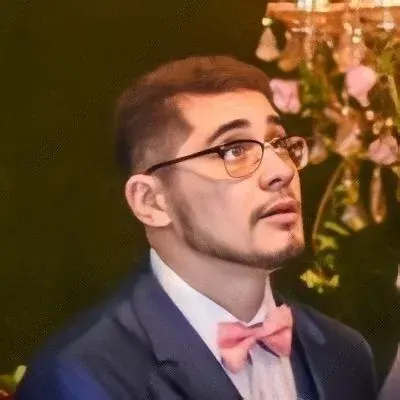
Demystifying the Repository Pattern and Service Layer 💡💪
So you're working on an ASP.NET MVC 3 app and you're scratching your head trying to understand the difference between the Repository Pattern and a Service Layer, huh? No worries, my friend! We've all been there 🤷♀️
In this blog post, we're going to break it down for you in the simplest possible way. We'll define each concept, explain their purpose, and finally, help you see the light at the end of the tunnel. Let's do this! 🚀
Understanding the Repository Pattern 📚
The Repository Pattern is a software design pattern that provides a way to encapsulate the data access logic for a specific domain entity or aggregate. In other words, it acts as a layer between your application and the data persistence layer (typically a database).
Here's what a common Repository Pattern implementation might look like:
public interface IUsersRepository
{
User GetById(int userId);
void Save(User user);
void Delete(User user);
// ...
}
public class UsersRepository : IUsersRepository
{
public User GetById(int userId)
{
// logic to fetch user from the database
}
public void Save(User user)
{
// logic to save user changes to the database
}
public void Delete(User user)
{
// logic to delete user from the database
}
}
The Repository Pattern abstracts away the details of how to interact with the database, allowing your application to work with domain entities in a more CRUD-like manner. This separation of concerns promotes maintainability and testability.
Unraveling the Service Layer 🎛
Now, let's switch gears and talk about the Service Layer. The Service Layer is another software design pattern that focuses on encapsulating the business logic of your application. It acts as a mediator between the presentation layer (such as your controllers) and the data access layer (repositories).
Here's a simplified example of how a Service Layer might look:
public interface IUsersService
{
User GetUserById(int userId);
void UpdateUser(User user);
void DeleteUser(int userId);
// ...
}
public class UsersService : IUsersService
{
private readonly IUsersRepository _usersRepository;
public UsersService(IUsersRepository usersRepository)
{
_usersRepository = usersRepository;
}
public User GetUserById(int userId)
{
// validation or additional processing
return _usersRepository.GetById(userId);
}
public void UpdateUser(User user)
{
// additional business logic or validation
_usersRepository.Save(user);
}
public void DeleteUser(int userId)
{
// additional business logic or validation
var user = _usersRepository.GetById(userId);
_usersRepository.Delete(user);
}
}
The Service Layer acts as the bridge between your application's presentation layer and the repositories. It coordinates the retrieval and persistence of data, applies additional business rules or validation, and ensures the proper behavior of your application.
So, What's the Difference? 🤔
Now that we have a basic understanding of both the Repository Pattern and the Service Layer, let's highlight the key differences:
Purpose: The Repository Pattern focuses on abstracting the data access logic and providing a simplified API for working with domain entities. It encapsulates CRUD operations and shields the rest of the application from the implementation details of the data persistence layer.
On the other hand, the Service Layer is responsible for orchestrating business logic, handling complex operations, and coordinating interactions between the presentation layer and the repositories. It acts as a controller or manager, ensuring the consistency and integrity of your application's behavior.
Relationship: The Repository Pattern is typically used by the Service Layer to interact with the database or data persistence layer. The Service Layer leverages the repositories to perform data retrieval and persistence, applying additional logic or validation when necessary.
Wrapping It Up 🎁
Congratulations! You made it through understanding the Repository Pattern and the Service Layer. Now, take a deep breath and appreciate how far you've come. 🙌
Remember, the Repository Pattern simplifies data access by providing an abstraction layer, while the Service Layer focuses on coordinating business logic and orchestrating interactions between the presentation and data layers.
If you found this blog post helpful, feel free to share it with your fellow developers who might be struggling with the same concepts. And if you have any additional questions or insights, don't hesitate to leave a comment below. Let's embark on this programming journey together! 👩💻🚀
Thanks for reading! ✨
Your friendly tech blogger 😎