ASP.NET MVC: Unit testing controllers that use UrlHelper
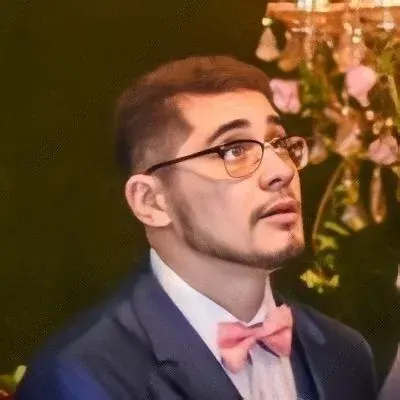
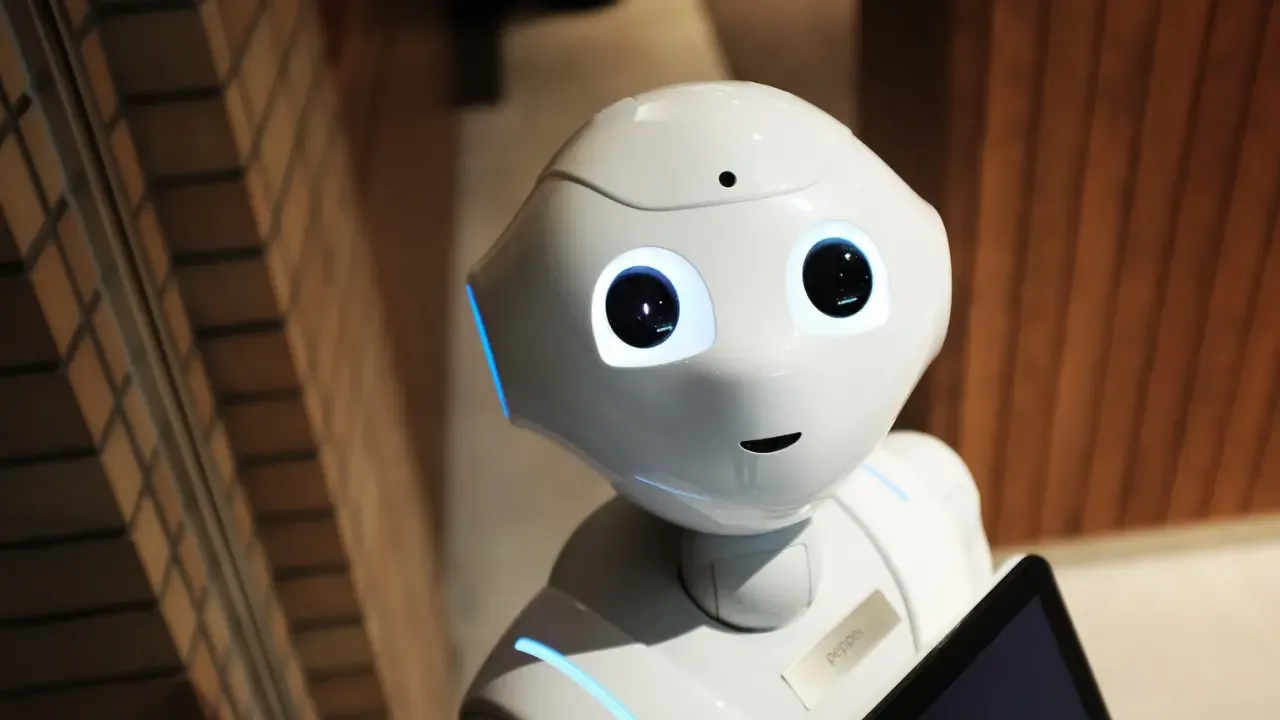
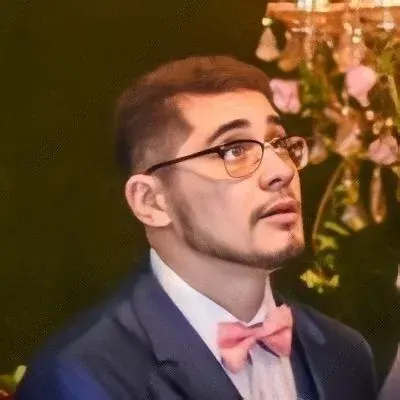
๐ฏ Unit Testing Controllers that Use UrlHelper in ASP.NET MVC
Have you ever encountered issues when trying to unit test controllers that use UrlHelper
in your ASP.NET MVC application? ๐ค If so, you're not alone!
In this blog post, we'll tackle the common problem of testing controllers that utilize UrlHelper
for generating URLs, and we'll break down some easy-to-implement solutions that will have you writing effective unit tests in no time! ๐ช
๐งฉ The Issue
Let's set the stage. You have a controller action that gets called during an Ajax request, and it returns a URL to the client side for redirection purposes. Inside this action, you're using Url.RouteUrl()
to generate the desired URL. However, when running your unit tests, you encounter failures because the Controller.Url
parameter hasn't been pre-filled. ๐ฑ
You've tried various approaches, including attempting to stub UrlHelper
(which failed) and manually creating a UrlHelper
with a stubbed HttpContextBase
(which failed on a RouteCollection.GetUrlWithApplicationPath
call). You've scoured Google for solutions, but you've found almost nothing on this subject. Are you doing something incredibly stupid by using Url.RouteUrl
in your controller action? Is there an easier way? ๐ค
๐ The Solution
Fear not! We have some effective solutions that will help you overcome these challenges and write successful unit tests for your controllers that use UrlHelper
. Let's dive in:
1. Mocking UrlHelper
Using a Mocking Framework
One way to tackle this issue is by utilizing a mocking framework, such as Moq or NSubstitute. You can create a mock UrlHelper
object and set it up to return the expected URL when Url.RouteUrl()
is called. Here's an example using Moq:
// Arrange
var urlHelperMock = new Mock<UrlHelper>();
urlHelperMock.Setup(u => u.RouteUrl(It.IsAny<string>(), It.IsAny<object>()))
.Returns("http://example.com"); // Specify the expected URL
var controller = new YourController();
controller.Url = urlHelperMock.Object; // Set the UrlHelper mock
// Act
var result = controller.YourAction();
// Assert
// Add your assertions here
2. Creating a Mock UrlHelper
Manually
If you prefer to avoid using a mocking framework, you can create a mock UrlHelper
manually by inheriting from the UrlHelper
class and overriding the necessary methods. Here's an example:
public class MockUrlHelper : UrlHelper
{
public MockUrlHelper()
: base(new RequestContext(new MockHttpContext(), new RouteData()))
{
}
public override string RouteUrl(string routeName, object routeValues)
{
// Return the expected URL based on the route name and route values
return "http://example.com";
}
}
public class MockHttpContext : HttpContextBase
{
// Add necessary members to mock HttpContextBase functionality
}
Then, in your unit test:
// Arrange
var urlHelper = new MockUrlHelper();
var controller = new YourController();
controller.Url = urlHelper; // Set the mock UrlHelper
// Act
var result = controller.YourAction();
// Assert
// Add your assertions here
3. Using a Stubbed RouteCollection
To take your unit tests to the next level, you might want to control the URL resolution process by using a stubbed RouteCollection
. This allows you to test whether the correct route is being used for generating the URL. Here's a snippet demonstrating this approach:
// Arrange
var stubbedRouteCollection = new RouteCollection();
// Set up the necessary routes in the stubbed RouteCollection
var urlHelper = new UrlHelper(new RequestContext(new MockHttpContext(), new RouteData()), stubbedRouteCollection);
var controller = new YourController();
controller.Url = urlHelper; // Set the UrlHelper with the stubbed RouteCollection
// Act
var result = controller.YourAction();
// Assert
// Add your assertions here
โก๏ธ Your Next Step
Now that you have a solid understanding of how to unit test controllers that use UrlHelper
in ASP.NET MVC, it's time to put this knowledge into action! Start by implementing one of the aforementioned solutions in your tests and observe the improved test coverage and confidence in your application's behavior. ๐
Remember, writing effective unit tests is crucial for maintaining a robust and stable codebase. Don't let the challenges of testing controllers using UrlHelper
hinder your progress!
We hope you found this guide helpful! If you have any questions or additional insights, we'd love to hear from you in the comments below. Happy testing! ๐๐งช
๐ Interested in learning more? Check out these related resources:
Disclaimer: The code snippets provided in this blog post are for illustrative purposes only and may require additional customization based on your specific application's needs.