ASP.NET MVC Html.ValidationSummary(true) does not display model errors
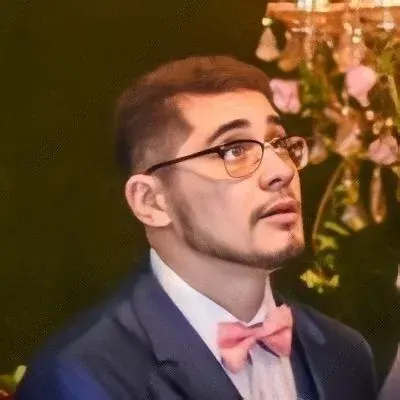
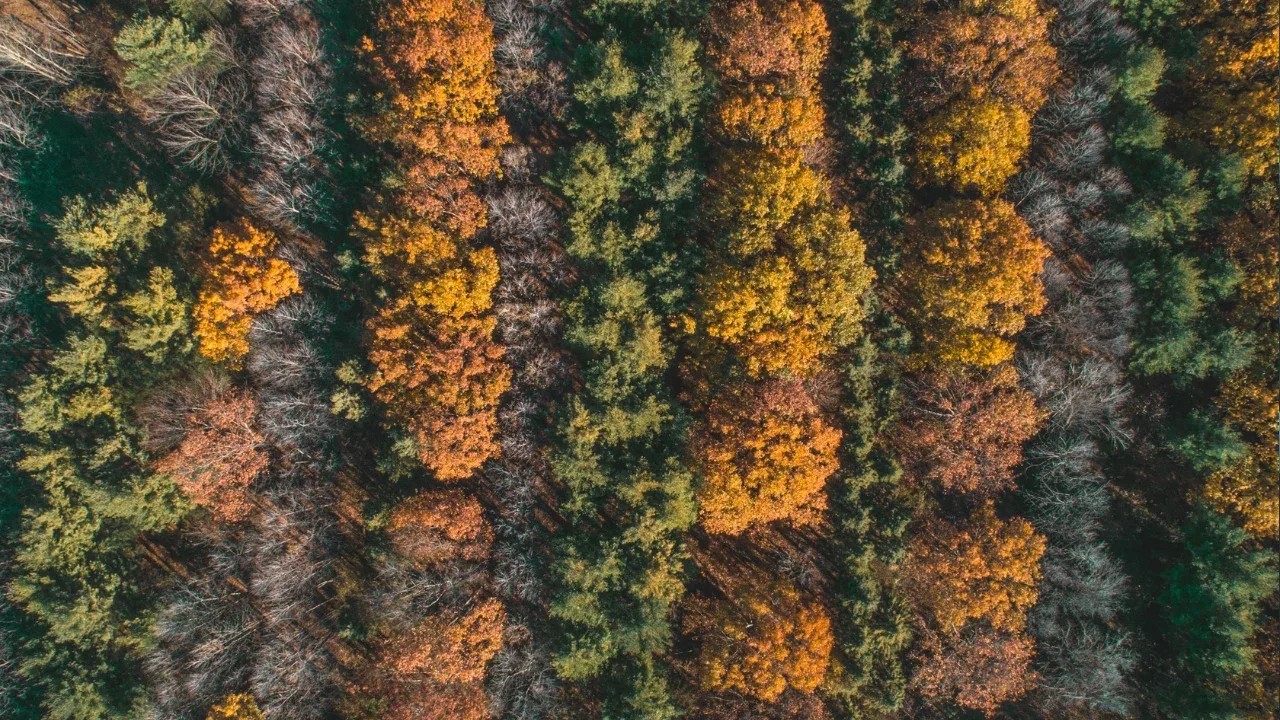
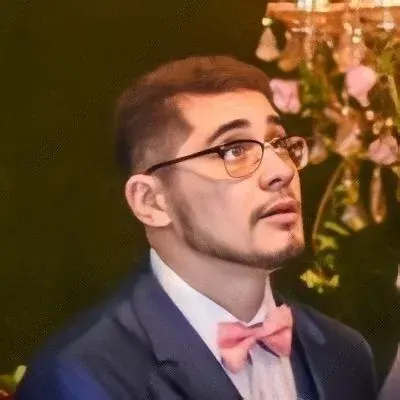
📝 Blog Post: ASP.NET MVC Html.ValidationSummary(true) Does Not Display Model Errors
Are you facing a problem with the Html.ValidationSummary
function in your ASP.NET MVC project? Is it not displaying the error messages from the ModelState
when using the Html.ValidationSummary(true)
parameter? Don't worry, you're not alone! In this blog post, we'll dive into this common issue and provide you with an easy solution to fix it.
The Problem
One user encountered an issue where they didn't want to display property errors in the Validation Summary. However, when they set Html.ValidationSummary(true)
, it wasn't showing the error messages from the ModelState
. Here's the code snippet that adds an error to the ModelState
:
try
{
// Some code here
}
catch (Exception ex)
{
ModelState.AddModelError("error", ex.Message);
return View(member);
}
And here's the code the user was using in their view:
<% using (Html.BeginForm("Register", "Members", FormMethod.Post, new { enctype = "multipart/form-data" })) {%>
<p>
<%= Html.LabelFor(model => model.Login)%>
<%= Html.TextBoxFor(model => model.Login)%>
<%= Html.ValidationMessageFor(model => model.Login)%>
</p>
<p>
<%= Html.LabelFor(model => model.Password)%>
<%= Html.PasswordFor(model => model.Password)%>
<%= Html.ValidationMessageFor(model => model.Password)%>
</p>
<p>
<%= Html.LabelFor(model => model.ConfirmPassword)%>
<%= Html.PasswordFor(model => model.ConfirmPassword)%>
<%= Html.ValidationMessageFor(model => model.ConfirmPassword)%>
</p>
<div>
<input type="submit" value="Create" />
</div>
<%= Html.ValidationSummary(true)%>
<% } %>
The Solution
To fix this issue and display the error messages from the ModelState
using Html.ValidationSummary(true)
, follow these steps:
Make sure you have the following nuget package installed:
Microsoft.AspNet.Mvc
.Add the following code to your view:
@using System.Text.RegularExpressions
@{
string errorMessage = string.Empty;
if (ViewData.ModelState.ContainsKey("error"))
errorMessage = Regex.Replace(ViewData.ModelState["error"].Errors.FirstOrDefault().ErrorMessage, "<[^>]*(>|$)", "");
}
@if (!string.IsNullOrEmpty(errorMessage))
{
<div class="alert alert-danger">
@errorMessage
</div>
}
Replace the line
<%= Html.ValidationSummary(true)%>
with the following code:
<%= Html.ValidationSummary(false)%>
Save the changes, and you're good to go! Now, when an error is added to the
ModelState
, it will be displayed in theHtml.ValidationSummary(false)
section.
Call-to-Action
Have you ever faced this issue with Html.ValidationSummary
in your ASP.NET MVC project? Did this easy solution help you fix it? Share your experience and let us know in the comments section below!
Don't forget to subscribe to our newsletter to receive more helpful tips and tricks for ASP.NET MVC development.
Happy coding! 😄🚀