ASP.NET MVC 3 Razor - Adding class to EditorFor
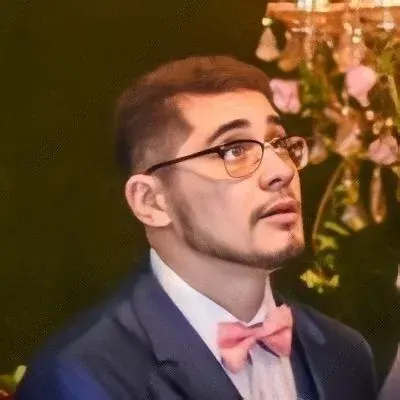
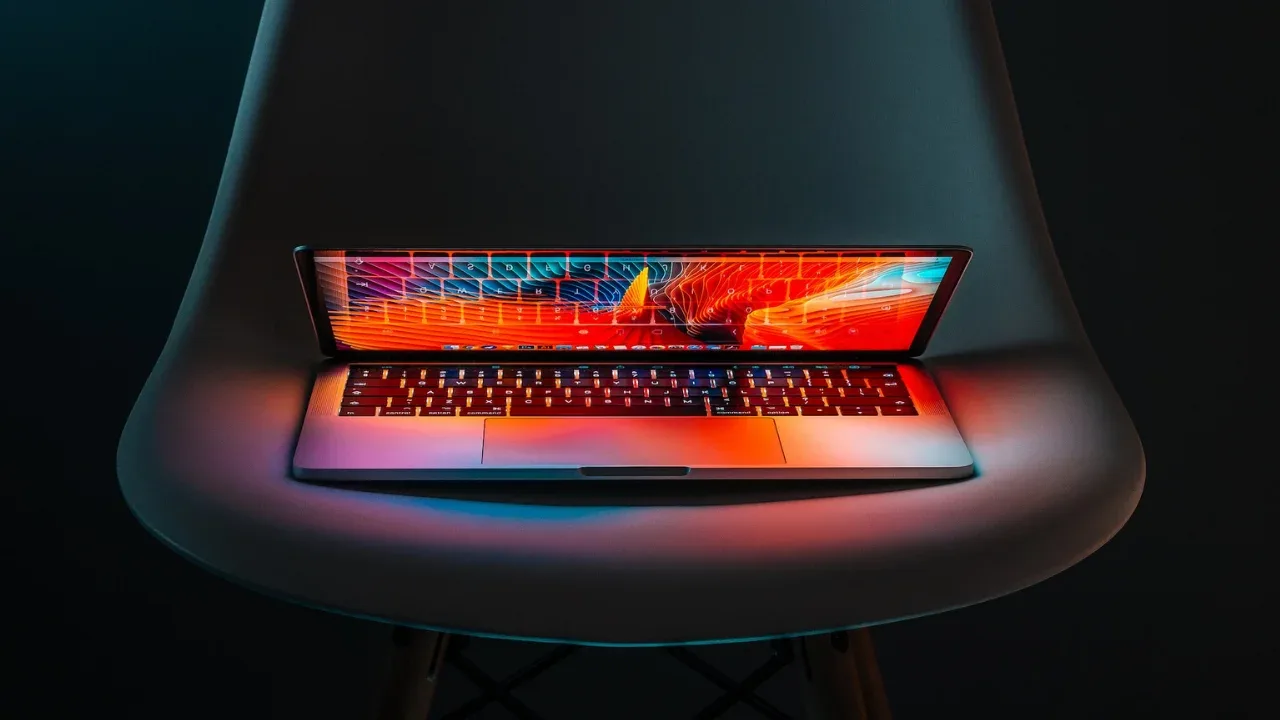
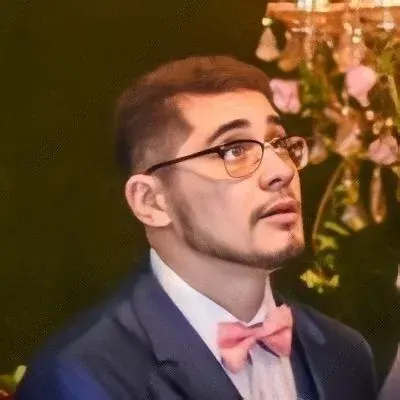
ASP.NET MVC 3 Razor - Adding class to EditorFor
So you're trying to add a class to an input field in ASP.NET MVC 3 Razor, but it's just not working? Not to worry, we've got a simple solution for you!
The Problem
Let's take a look at the code snippet that is causing you trouble:
@Html.EditorFor(x => x.Created, new { @class = "date" })
This code may seem fine at first glance, but there is a small issue. When using EditorFor
, the additional attributes are not applied directly to the generated input field. So, adding the @class
attribute like this won't work.
The Solution
To add a class to the EditorFor
input field, we need to make a small modification to the code. We can achieve this by using Editor
instead of EditorFor
and applying the class directly to the HTML attributes.
Here's what the updated code looks like:
@Html.Editor("Created", new { htmlAttributes = new { @class = "date" } })
With this modification, the class "date" will now be applied to the input field.
An Example
Let's consider a scenario where you have a Person
model with a Name
property that needs to have a class applied to it.
Step 1: Create your model
public class Person
{
public string Name { get; set; }
}
Step 2: Update your view
In your view, use the Editor
method to add the class to the input field:
@model Person
@Html.Editor("Name", new { htmlAttributes = new { @class = "custom-class" } })
Step 3: Check the output
When the view is rendered, you'll notice that the input field for the Name
property has the class "custom-class" applied to it.
Take It a Step Further
Adding a class to an EditorFor
field might just be the tip of the iceberg when it comes to customizing your forms. If you're interested in diving deeper into ASP.NET MVC 3 Razor and want to learn more about form customization, handle form submissions, or style your forms with CSS, check out our comprehensive guide on ASP.NET MVC 3 Razor Forms.
Wrap Up
Now you know how to add a class to an EditorFor
input field in ASP.NET MVC 3 Razor. By using the Editor
method and applying the class directly to the HTML attributes, you can easily customize your forms to fit your needs. Give it a try and see the difference it makes in your application!
If you found this blog post helpful, we'd love to hear your thoughts in the comments below. Have you encountered any other common issues with ASP.NET MVC 3 Razor? Let us know and we'll be glad to help!