Allow multiple roles to access controller action
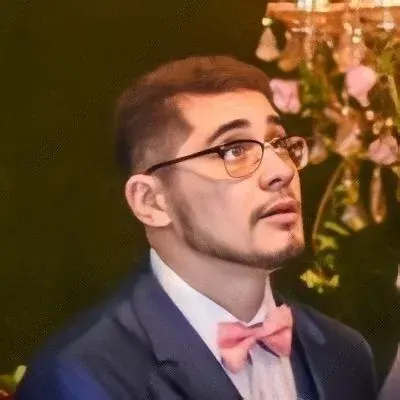
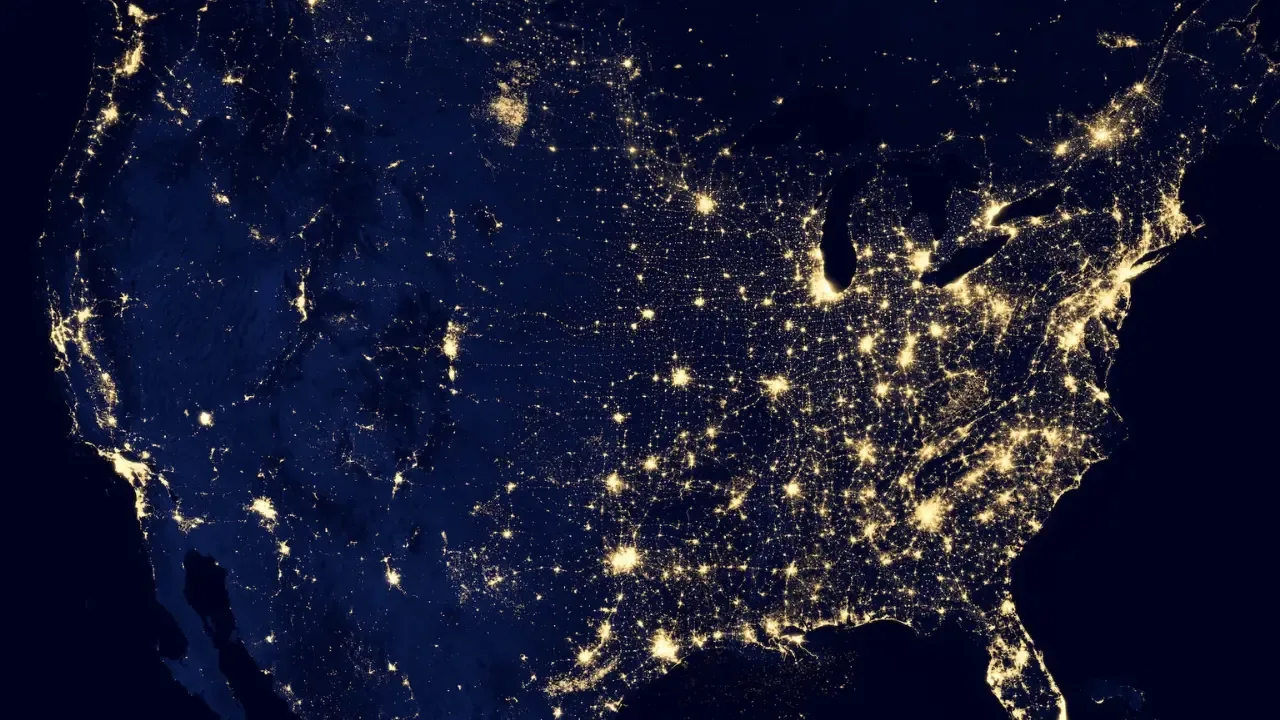
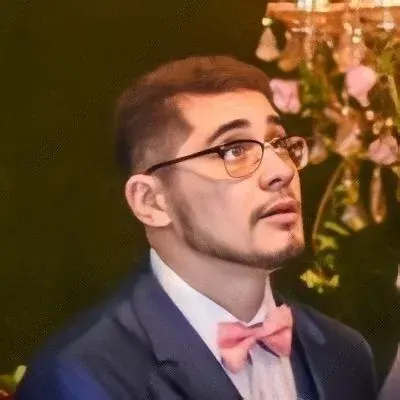
🚀 Allowing Multiple Roles to Access Controller Action
Are you tired of only allowing one role to access your controller action? Do you need to let multiple roles access it with ease? Look no further! In this blog post, we'll dive into a common issue where developers struggle to allow more than one role to access a controller action. And don't worry, we'll provide you with simple and straightforward solutions. Let's get started! 🤩
The Problem 💥
Imagine you have a controller action that you want both "members" and "admin" roles to access. You might be tempted to write code like this:
[Authorize(Roles = "members", "admin")]
public IActionResult YourAction()
{
// Action code here
}
However, the above code won't work. You'll likely encounter an error because the Roles
attribute doesn't accept multiple roles in this way.
The Solution(s) 💡
Fear not! There are several ways to solve this problem and allow multiple roles to access your controller action. Let's explore a few simple solutions:
Solution 1: Using Multiple Authorize
Attributes
One way to solve this problem is by using multiple Authorize
attributes. Each attribute will specify a different role. Here's how you can do it:
[Authorize(Roles = "members")]
[Authorize(Roles = "admin")]
public IActionResult YourAction()
{
// Action code here
}
That's it! Both "members" and "admin" roles can now access your YourAction
method. 🎉
Solution 2: Using a Custom Role Attribute
Another solution is to create a custom role attribute that accepts multiple roles. This can be done by creating a custom attribute class inherited from the AuthorizeAttribute
. Let's see how it's done:
public class MultiRoleAuthorizeAttribute : AuthorizeAttribute
{
public MultiRoleAuthorizeAttribute(params string[] roles)
{
this.Roles = string.Join(",", roles);
}
}
[MultiRoleAuthorize("members", "admin")]
public IActionResult YourAction()
{
// Action code here
}
That's all there is to it! With the custom MultiRoleAuthorizeAttribute
, you can now specify multiple roles conveniently. 🎉
The Call-to-Action 📢
We hope these solutions have helped you overcome the hurdle of allowing multiple roles to access your controller action. Now, it's your turn! Try implementing these solutions in your own projects and let us know how they work for you. Have any other questions or ideas? Share them in the comments below! Let's keep the conversation going. 🔥💬
Remember, sharing is caring! If you found this blog post helpful, share it with your fellow developers. Together, we can make coding more accessible and enjoyable for everyone. Happy coding! 💻❤️