How to use ? : if statements with Razor and inline code blocks
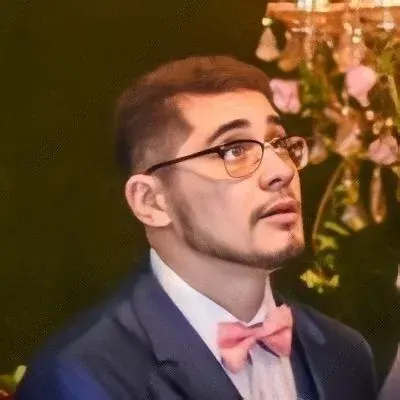
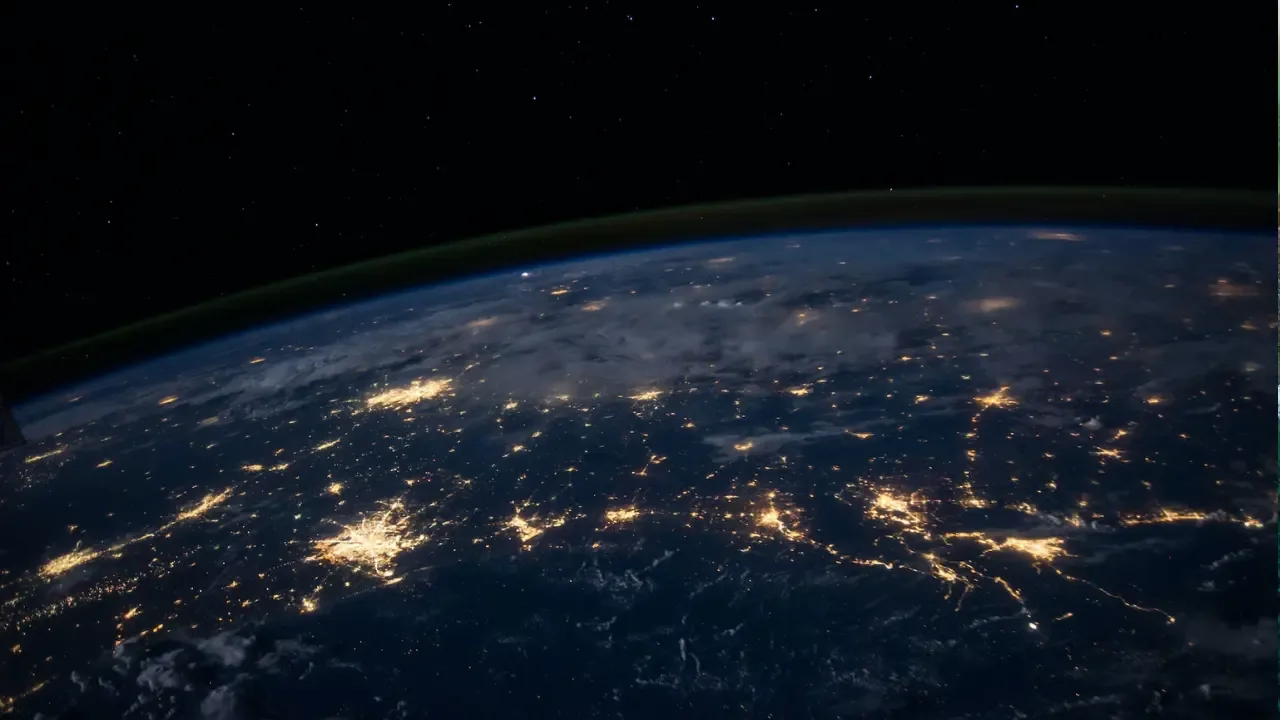
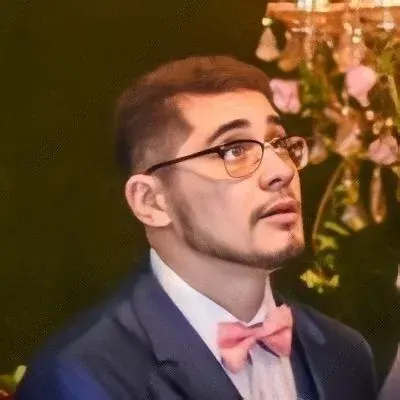
How to use ? : if statements with Razor and inline code blocks
Are you trying to update your old .aspx views with the new Razor view engine? Do you have code snippets that use if statements with inline code blocks, but you're facing some issues? Don't worry, we've got you covered! In this blog post, we'll address common problems and provide easy solutions to help you use if statements with Razor and inline code blocks seamlessly.
The Problem
Let's take a look at the code snippet that prompted this question:
<span class="vote-up<%= puzzle.UserVote == VoteType.Up ? "-selected" : "" %>">Vote Up</span>
The goal is to simplify it like this:
<span class="vote-up@{puzzle.UserVote == VoteType.Up ? "-selected" : ""}">Vote Up</span>
However, there are two issues that need to be addressed.
Issue 1: @ symbol not recognized as a code block start
The first problem is that vote-up@{puzzle.UserVote
doesn't treat the @
symbol as the start of a code block.
Issue 2: @puzzle.UserVote treated as a variable value
The second problem is that @puzzle.UserVote == VoteType.Up
considers the first part @puzzle.UserVote
as if it's supposed to render the value of the variable.
The Solution
Let's dive into the solutions for both of these issues.
Solution for Issue 1
To make sure the @
symbol is recognized as the start of the code block, you can enclose the entire inline code block within parentheses. Here's the modified code snippet:
<span class="vote-up@(puzzle.UserVote == VoteType.Up ? "-selected" : "")">Vote Up</span>
By adding parentheses around the inline code block, you ensure that the @
symbol is properly recognized.
Solution for Issue 2
To evaluate the @puzzle.UserVote
expression without rendering its value, you can simply add a prefix @:
before it. Here's the updated code snippet:
<span class="vote-up@(puzzle.UserVote == VoteType.Up ? "-selected" : "")">Vote Up</span>
By adding @:
before @puzzle.UserVote
, you let Razor know that it should evaluate the expression as code and not render its value.
Conclusion
Using if statements with Razor and inline code blocks can be tricky, but by following these simple solutions, you can overcome the common issues that arise. Remember to enclose the inline code block within parentheses to ensure the proper recognition of the @
symbol, and add @:
as a prefix to evaluate expressions without rendering their values.
We hope this guide helps you update your views smoothly and efficiently. If you have any more questions or face more challenges, feel free to leave a comment below. Happy coding! 👩💻👨💻
Did this guide help you? Share your experience in the comments below and let's keep the conversation going!