Get URL of ASP.Net Page in code-behind
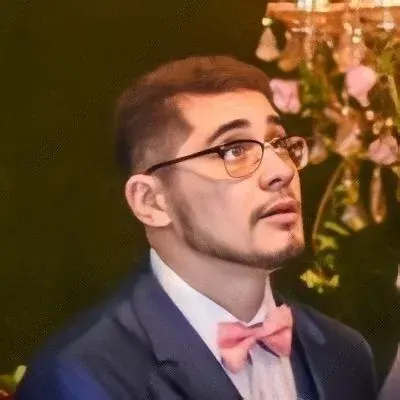
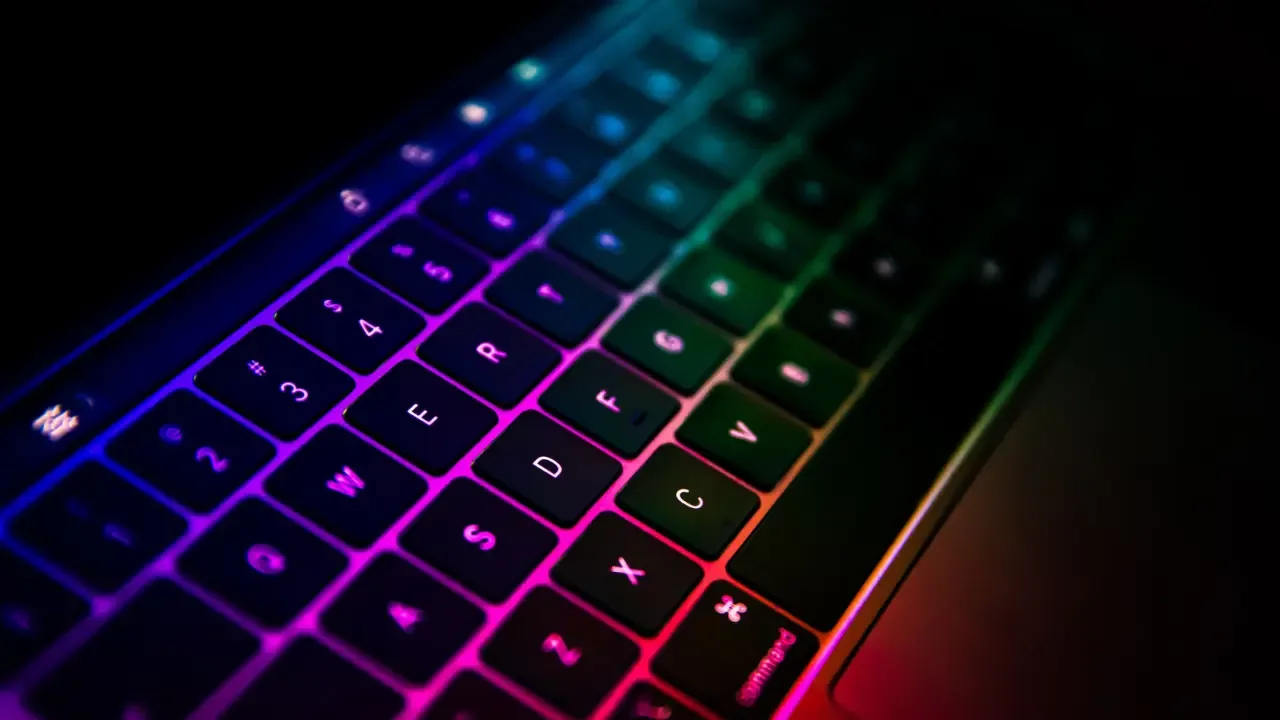
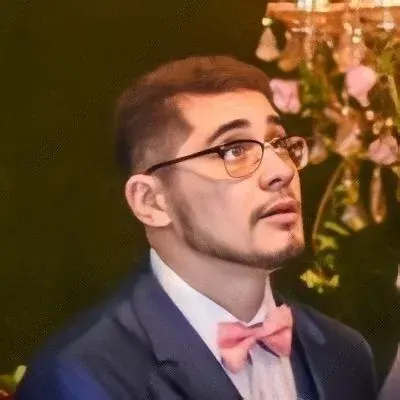
š Title: Get the URL of your ASP.Net page in the code-behind šāØ
š Hey there, web developers! If you've ever wondered how to easily retrieve the URL of your ASP.Net page in the code-behind, you're in the right place! šÆ
Sometimes, while working on an ASP.Net project, you might find yourself in a situation where you need to obtain the URL of your page or even the site where the page is hosted as a string for use in your code-behind. But fear not, as I'm here to provide you with some simple and effective solutions! Let's dive in! šŖ
ā”ļø Solution 1: Using the Request object
One way to accomplish this is by using the Request
object. You can access the Url
property from the HttpRequest
object to retrieve the URL of your current page. Here's how you can do it:
string url = Request.Url.ToString();
By calling the ToString()
method on the Url
property, you'll get the complete URL of the current page. Awesome, right? š
ā”ļø Solution 2: Using the HttpContext
object
Another approach you can take is by utilizing the HttpContext
object. This option provides more flexibility, allowing you to retrieve the URL of your page or the site hosting your page. Check out the code snippet below:
string url = HttpContext.Current.Request.Url.ToString();
Here, the Current
property of the HttpContext
class provides access to the current HTTP context, including the request information. By calling ToString()
on the Url
, you obtain the desired URL as a string.
ā”ļø Solution 3: Using the Page
object
Lastly, you can also leverage the Page
object to retrieve the URL of your ASP.Net page. Take a look at the following example:
string url = Page.Request.Url.ToString();
By referencing Page.Request.Url.ToString()
, you can acquire the URL as a string, just like in the previous solutions. It's as simple as that!
š” Pro Tip: If you also want to capture the site where the page is hosted, you can use the Uri.Scheme
and Uri.Authority
properties to obtain the scheme (HTTP/HTTPS) and the authority (domain and port) of the URL, respectively. Combine these properties with the Request.Url.AbsolutePath
to get a more comprehensive result.
š Congratulations!š You're now equipped with multiple options to easily retrieve the URL of your ASP.Net page in the code-behind. Choose the one that best suits your needs and make your development process smoother than ever! š
š¬ Share your thoughts! What other tricks do you use to obtain the URL in your ASP.Net projects? Let us know in the comments below! š
Now that you know how to get the URL in your code-behind, go out there and unleash your ASP.Net skills! āØš»
If you found this guide useful, don't forget to share it with your developer buddies! š¤āļø
Happy coding! šŖš