Best way to trim strings after data entry. Should I create a custom model binder?
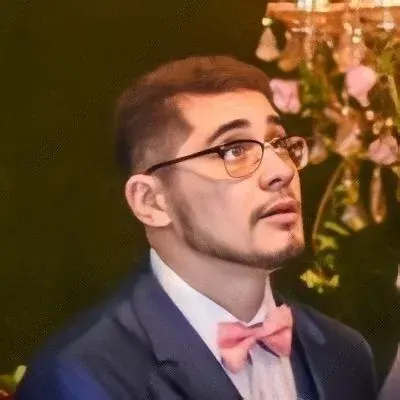
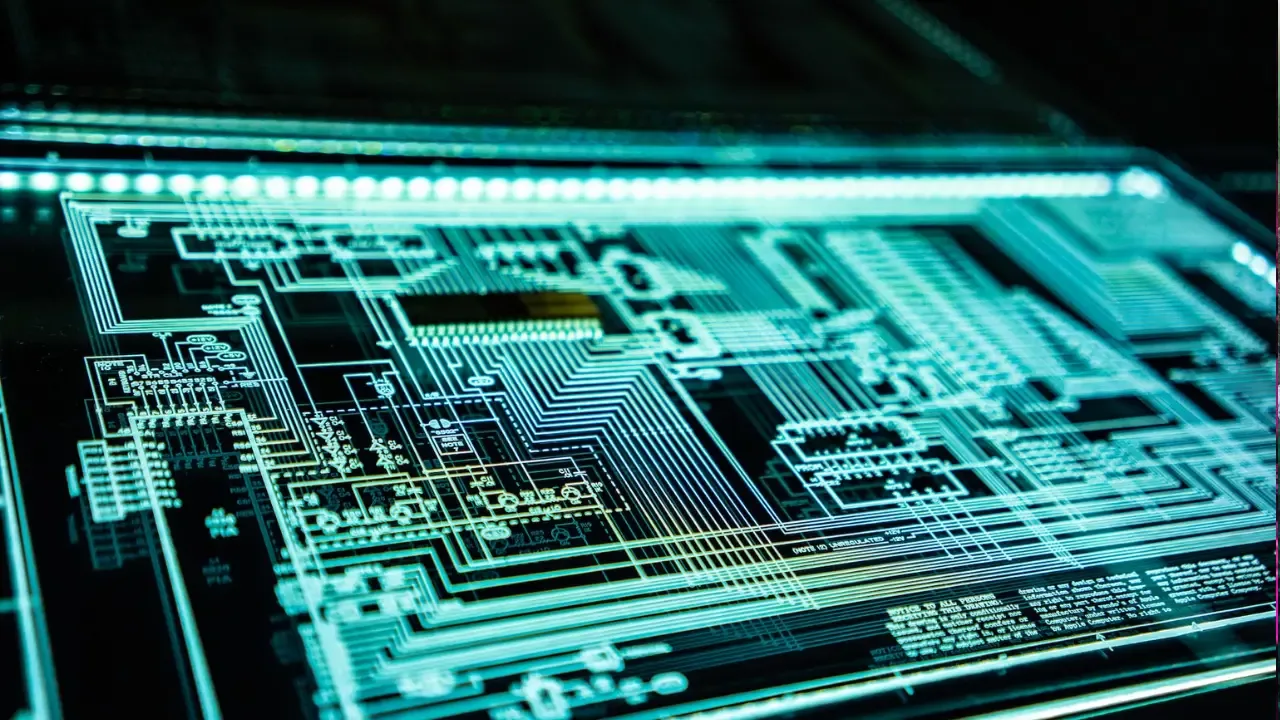
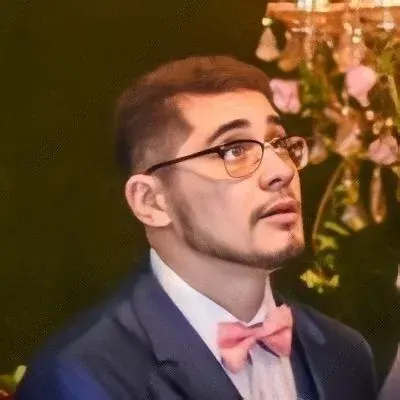
š»š§© Blog Post: Best Way to Trim Strings After Data Entry. Should I Create a Custom Model Binder? šŖ
š Hey there, tech-savvy folks! š Are you tired of manually trimming every single user-entered string value before storing it in your database? š¤·āāļø Well, you're in the right place! In this blog post, we're going to explore the best way to trim strings after data entry in ASP.NET MVC and discuss the potential benefits of creating a custom model binder. Let's dive in! šāāļø
š Understanding the Problem
The issue at hand is that you want to trim all user-entered string fields automatically before inserting them into the database. Instead of explicitly trimming each individual value, you're looking for an elegant solution that centralizes the trimming logic. š¤
š ļø The Custom Model Binder Approach
Creating a custom model binder is indeed a fantastic approach to tackle this problem. By implementing a custom model binder, you can define your own rules for binding and transform the user input before it reaches your controllers. This allows you to abstract away the trimming logic from your controllers and keeps your codebase cleaner and more maintainable. š
š” Easy Solutions and Code Samples
To get you started, here's a simple example of how you can create a custom model binder to trim string values:
public class TrimModelBinder : DefaultModelBinder
{
protected override void SetProperty(
ControllerContext controllerContext,
ModelBindingContext bindingContext,
PropertyDescriptor propertyDescriptor,
object value)
{
if (propertyDescriptor.PropertyType == typeof(string))
{
value = value?.ToString().Trim();
}
base.SetProperty(controllerContext, bindingContext, propertyDescriptor, value);
}
}
To make use of this custom model binder, you'll need to register it in your application's Global.asax.cs
file or using the new configuration system introduced in ASP.NET Core:
ModelBinders.Binders.DefaultBinder = new TrimModelBinder();
šļø Compelling Call-to-Action
Now that you've learned about the benefits of using a custom model binder for trimming string values after data entry, it's time to give it a try! š Implementing this technique in your ASP.NET MVC application can save you time, improve code maintainability, and ensure consistent data cleanliness throughout your project. So go ahead and put this knowledge into action! šŖ
š¬ Share Your Experience!
Have you ever faced a similar issue in your projects? How did you handle it? Let's start a discussion in the comments section below! š Your insights and experiences might help other developers facing the same dilemma. And don't forget to share this post with your fellow devs who may find it useful. Sharing is caring, after all! ā¤ļø
š Happy coding, and may the strings always be neatly trimmed in your applications! šāØ