ASP.NET Identity DbContext confusion
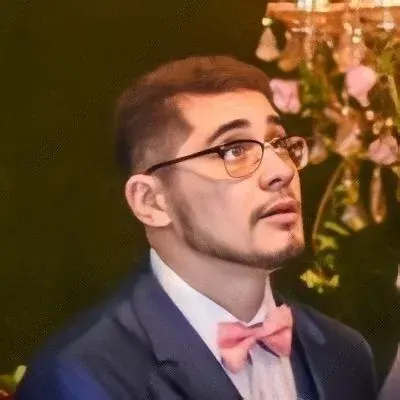
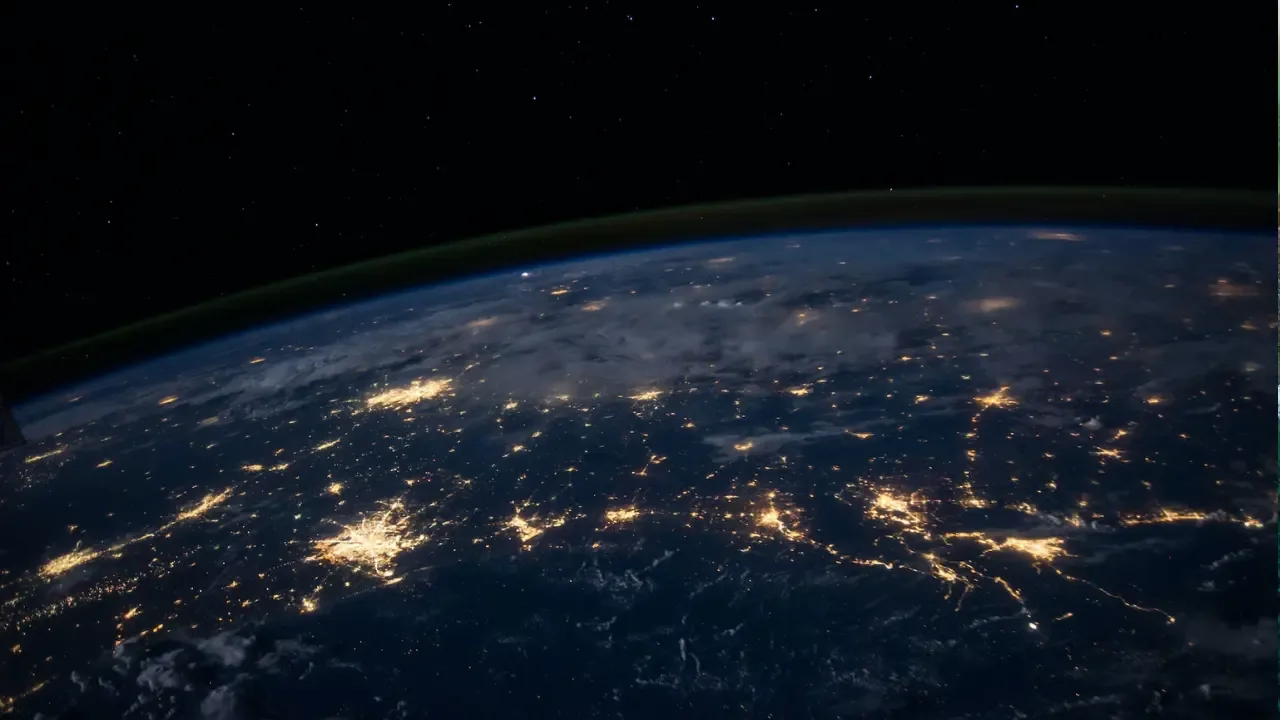
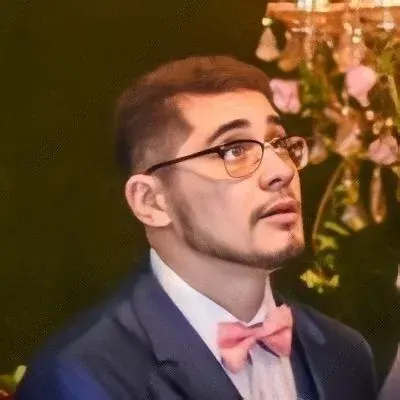
🔍 Understanding the problem
ASP.NET Identity is a framework that provides authentication and authorization for web applications. When working with ASP.NET Identity, you may come across confusion regarding the DbContext
that should be used for your own models.
In the provided code snippet, there are two DbContext
classes: ApplicationDbContext
and AllTheOtherStuffDbContext
. The ApplicationDbContext
is used for ASP.NET Identity operations, while the AllTheOtherStuffDbContext
is for your own models.
The question arises whether it is advisable to use both DbContext
classes or just stick to the default ApplicationDbContext
for all models.
💡 Easy solutions
The best practice in MVC 5 with ASP.NET Identity suggests using only one DbContext
for all models in your application. In this case, you should consider using the default ApplicationDbContext
that is generated for the ASP.NET Identity operations.
To utilize the ApplicationDbContext
for your own models, you can simply extend it to include the necessary DbSet<T>
properties for your additional models. For example, if you have an Animal
model, you can modify the ApplicationDbContext
as follows:
public class ApplicationDbContext : IdentityDbContext<Applicationuser>
{
public ApplicationDbContext() : base("DefaultConnection")
{
}
public DbSet<Animal> Animals { get; set; }
}
By adding the public DbSet<Animal> Animals { get; set; }
property, you can access the Animal
model through the ApplicationDbContext
.
📣 Call-to-action
To summarize, it is recommended to use the default ApplicationDbContext
for all your models in MVC 5 with ASP.NET Identity. Extend the ApplicationDbContext
to include the necessary DbSet<T>
properties for your own models.
By following this best practice, you will have a single DbContext
object in your application and avoid any potential overhead.
🤝 Now it's your turn!
Have you encountered any confusion related to ASP.NET Identity or DbContext
inheritance? Share your experience and thoughts in the comments below. Let's discuss the best practices together! 👇