Adding ASP.NET MVC5 Identity Authentication to an existing project
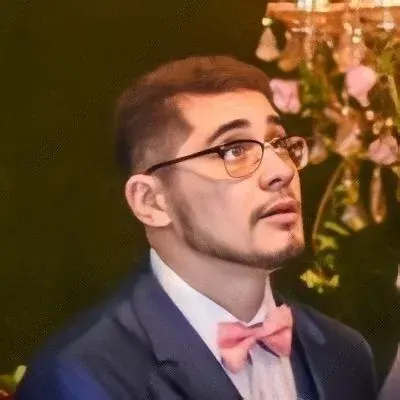
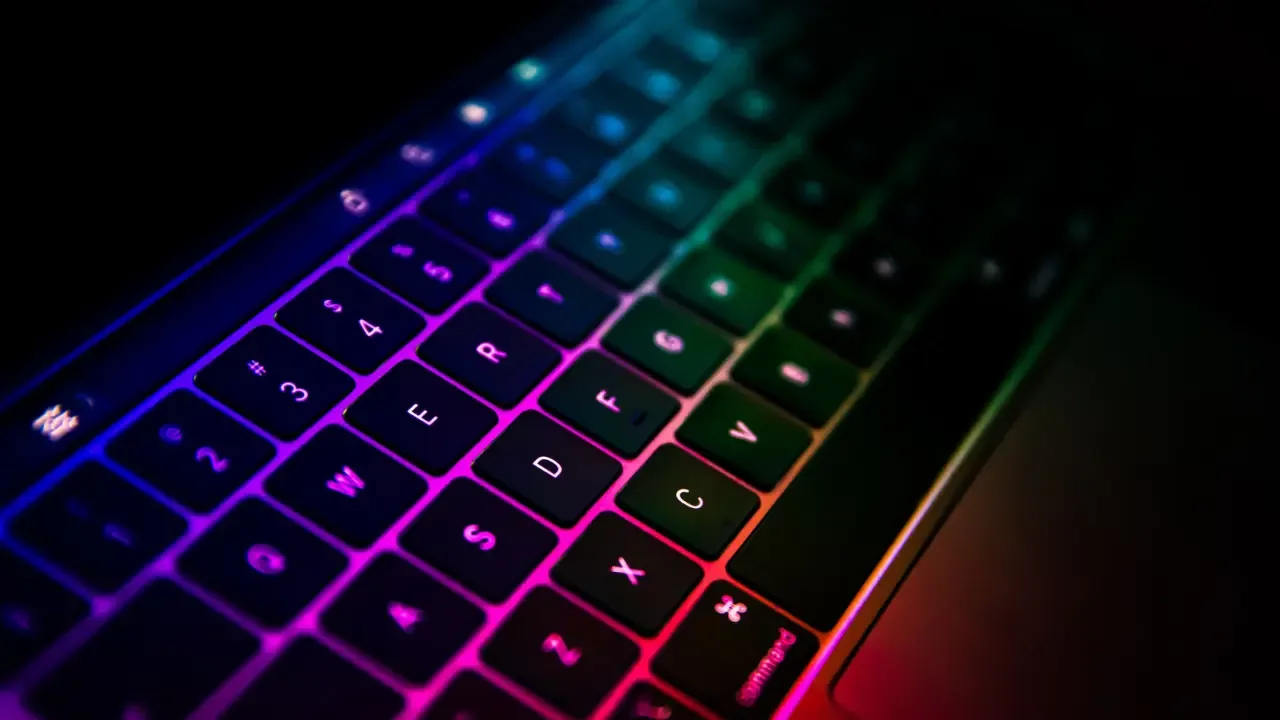
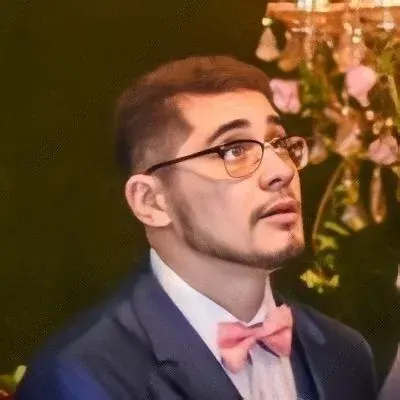
Adding ASP.NET MVC5 Identity Authentication to an Existing Project: A Simple Guide
So you've got an existing ASP.NET MVC5 project and you want to add ASP.NET MVC5 Identity authentication features like login, email confirmation, and password reset. You may have searched the internet and found similar pages, but they all seem to be using new projects or lack the necessary features.
No worries, we've got you covered! In this blog post, we'll guide you through the process of integrating ASP.NET MVC5 Identity authentication features into your existing project, along with creating the necessary tables in your database using EF Code-First.
Understanding the Scope
Before diving into the implementation, it's important to understand what ASP.NET MVC5 Identity is and its role in your project. ASP.NET MVC5 Identity is a membership framework that provides ready-to-use authentication and authorization functionalities for your application.
Step 1: Add Package Dependencies
The first step is to add the necessary ASP.NET MVC5 Identity package dependencies to your project. Open your project in Visual Studio and navigate to the Package Manager Console (Tools -> NuGet Package Manager -> Package Manager Console
).
In the Package Manager Console, run the following commands:
Install-Package Microsoft.AspNet.Identity.EntityFramework
Install-Package Microsoft.AspNet.Identity.Owin
Install-Package Microsoft.Owin.Security.Cookies
These packages will provide the required components for implementing ASP.NET MVC5 Identity in your project.
Step 2: Configure Identity
Now that you have the necessary packages, it's time to configure ASP.NET MVC5 Identity in your project. Open the Startup.Auth.cs
file in your project and locate the ConfigureAuth
method.
Within the ConfigureAuth
method, add the following code to enable ASP.NET MVC5 Identity's authentication and cookie middleware:
app.UseCookieAuthentication(new CookieAuthenticationOptions
{
AuthenticationType = DefaultAuthenticationTypes.ApplicationCookie,
LoginPath = new PathString("/Account/Login"),
});
This code sets the authentication type to ApplicationCookie
and specifies the login path for unauthorized access.
Step 3: Add Identity-related Tables to the Database
Since you're using EF Code-First in your project, you'll need to create the necessary Identity-related tables in your database. Open the DbContext
class file (often named ApplicationDbContext.cs
) and add the following code:
using Microsoft.AspNet.Identity.EntityFramework;
public class ApplicationDbContext : IdentityDbContext<ApplicationUser>
{
// Your existing DbSet properties go here
protected override void OnModelCreating(DbModelBuilder modelBuilder)
{
base.OnModelCreating(modelBuilder);
modelBuilder.Entity<ApplicationUser>().ToTable("Users");
modelBuilder.Entity<IdentityRole>().ToTable("Roles");
modelBuilder.Entity<IdentityUserRole>().ToTable("UserRoles");
modelBuilder.Entity<IdentityUserClaim>().ToTable("UserClaims");
modelBuilder.Entity<IdentityUserLogin>().ToTable("UserLogins");
}
}
This code extends the IdentityDbContext
class from Microsoft.AspNet.Identity.EntityFramework
and configures the names of the Identity-related tables in your database.
Step 4: Add Controllers and Views
To make use of ASP.NET MVC5 Identity's authentication features, you'll need to add controllers and views for user registration, login, email confirmation, and password reset.
You can either manually create these controllers and views or use Visual Studio scaffolding to generate them for you. To use scaffolding, right-click on the desired folder in your project (e.g., Controllers
or Views
) and select Add -> Controller or Add -> View.
Step 5: Customize as Needed
At this point, you should have the basic ASP.NET MVC5 Identity authentication features integrated into your existing project. However, you may need to customize and extend these features to fit your application's specific requirements.
Refer to the official ASP.NET documentation and Stack Overflow for further guidance on customizing ASP.NET MVC5 Identity in your project.
Step 6: Share Your Success Story!
Congratulations, you've successfully added ASP.NET MVC5 Identity authentication to your existing project! Now it's time to share your success with the world.
Leave a comment below and let us know how this guide helped you. Did you encounter any challenges? How did you overcome them? We'd love to hear your experiences and answer any questions you may have!
Remember, the key to success is sharing knowledge and helping others in their tech journey. So don't forget to share this blog post with your fellow developers who might be struggling with ASP.NET MVC5 Identity authentication in their existing projects.
Stay tuned for more exciting tech tips and tricks on our blog!