VBA using ubound on a multidimensional array
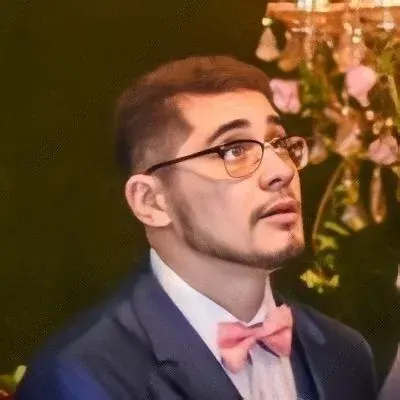
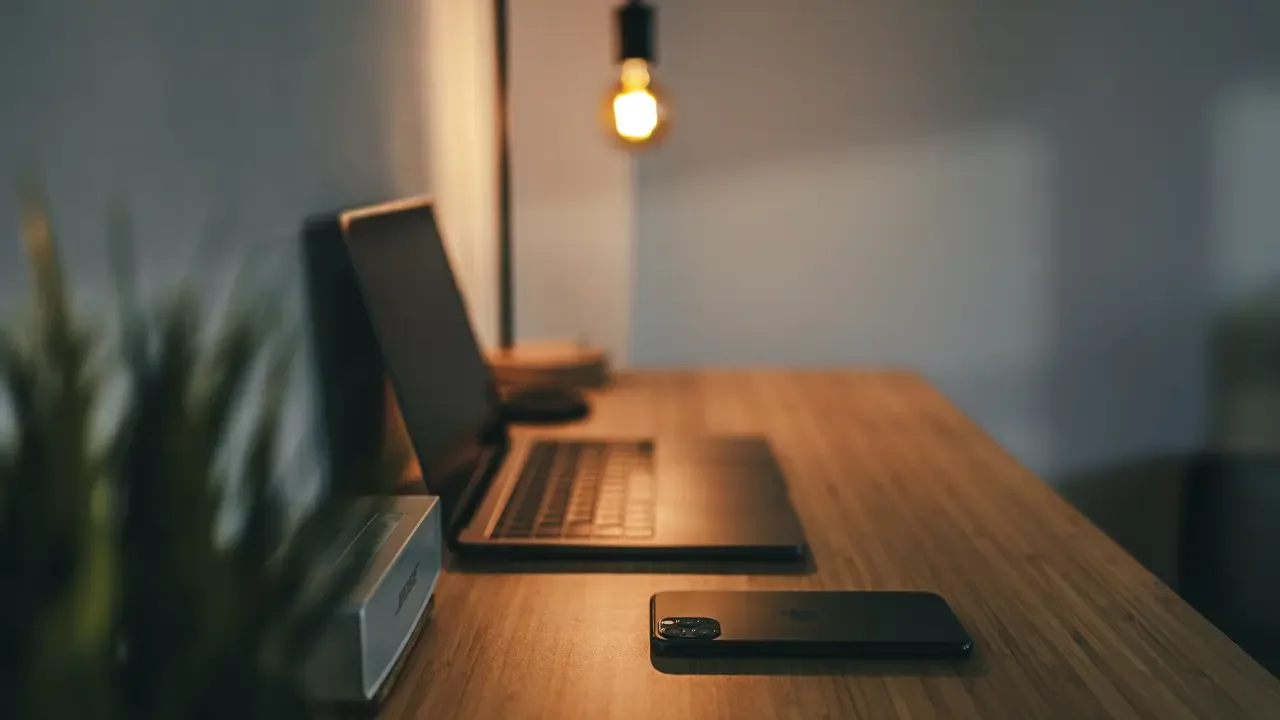
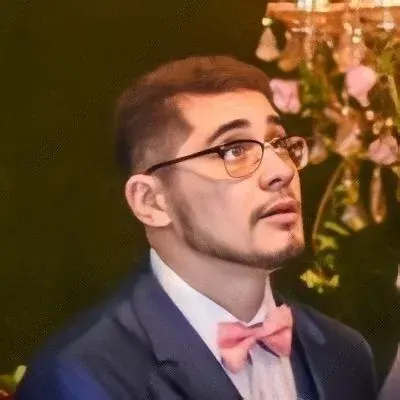
📝 VBA Using Ubound on a Multidimensional Array: Demystified!
Welcome, tech enthusiasts and aspiring VBA wizards! Today, we tackle a common conundrum that many programmers face when dealing with multidimensional arrays in VBA: how to effectively use the Ubound
function to obtain the maximum index value for a specific dimension. 🤔
Let's dive right in, shall we? 💪
The Ubound Riddle: Solving the Mystery of Multiple Dimensions
The Ubound
function in VBA is a powerful tool that returns the maximum index value of an array. However, when it comes to multidimensional arrays, it can become a bit tricky to specify which dimension you want to focus on.
Consider the following example:
Dim arr(1 to 4, 1 to 3) As Variant
In this 4x3 array, how do we instruct Ubound
to return either 4 or 3, corresponding to each dimension? Let's unravel the mystery together! 🕵️♂️
Solution 1: Single-Dimensional Approach
If you are interested in finding the maximum index value of a specific dimension independently, you can utilize a simple trick: iterate over the desired dimension and increment a counter until reaching the maximum value.
Let's say we want to obtain the maximum value for the first dimension (rows) in our example array. We can achieve this with the following code snippet:
Dim maxRow As Long
Dim i As Long
For i = LBound(arr, 1) To UBound(arr, 1)
maxRow = i
Next i
Debug.Print maxRow ' Output: 4
By looping through the array from the lower bound (LBound
) to the upper bound (UBound
) of the desired dimension, the maxRow
variable will eventually hold the maximum index value for that dimension. Easy peasy! 😎
Solution 2: Gathering Intel on All Dimensions
On the other hand, if you want to determine the maximum index value for all dimensions of a multidimensional array, VBA has got you covered too! 🎉
You can use the UBound
function without specifying the dimension parameter, and it will return an array containing the maximum index values for each dimension.
Check out this snippet to witness the magic in action:
Dim maxIndices() As Long
Dim i As Long
For i = 1 To Len(arr, i)
ReDim Preserve maxIndices(i)
maxIndices(i) = UBound(arr, i)
Next i
For i = 1 To Len(maxIndices)
Debug.Print "Dimension " & i & ": " & maxIndices(i)
Next i
' Output:
' Dimension 1: 4
' Dimension 2: 3
By using a dynamic array maxIndices
and looping through all dimensions of the array, we can store and display the maximum index value for each dimension using Debug.Print
. Mind-blowing, right? 🤯
Your Turn to Conquer VBA Multidimensional Arrays!
Now that we have unravelled the mysteries of using Ubound
on multidimensional arrays, it's your turn to put these solutions into practice! 🚀
Try incorporating these techniques into your VBA projects and let us know how they work out for you. Have you encountered any other interesting challenges with multidimensional arrays? Share your experiences and insights in the comments section below to engage with our vibrant community. 👇
Remember, the path to VBA greatness is paved with curiosity, exploration, and collaborative learning! Join us in our quest to conquer the tech realm, one multidimensional array at a time. Happy coding! 💻🌟