VBA array sort function?
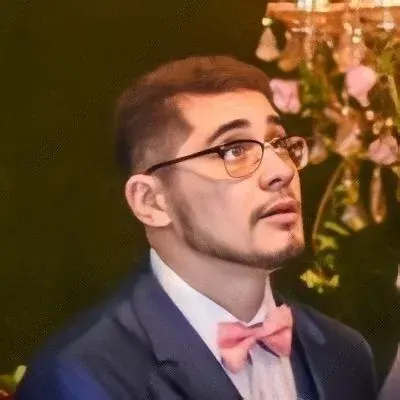
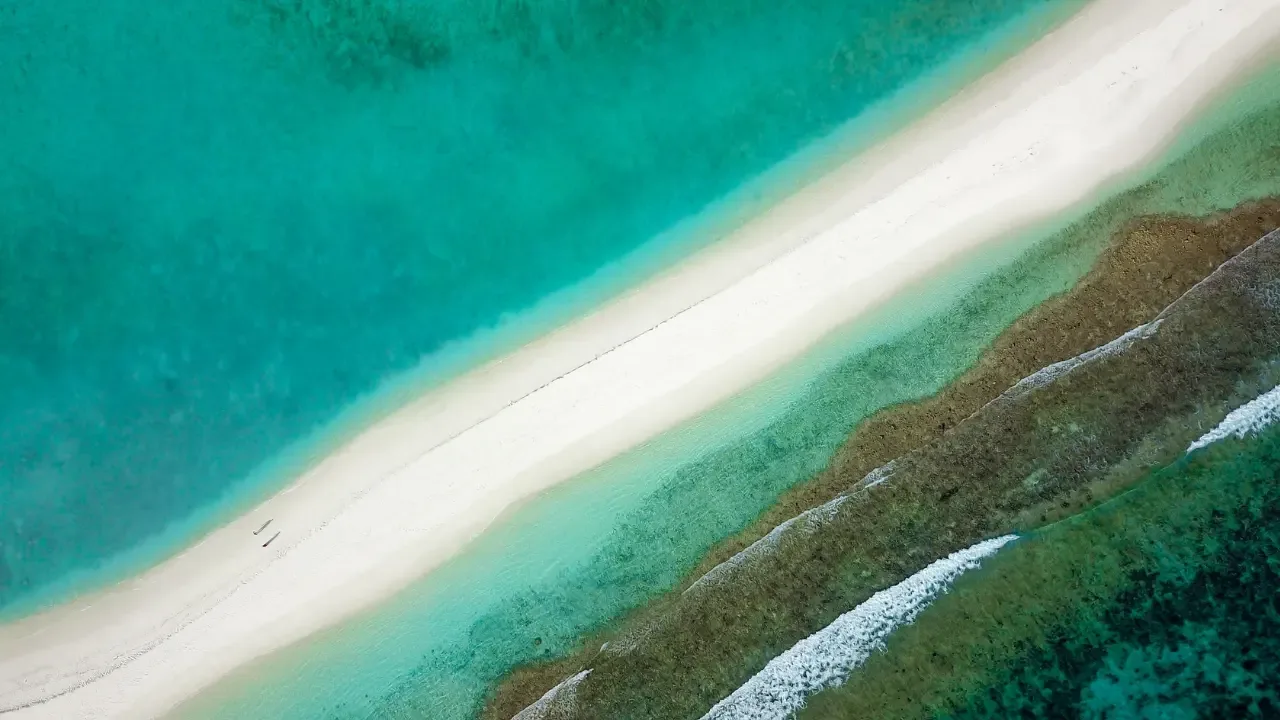
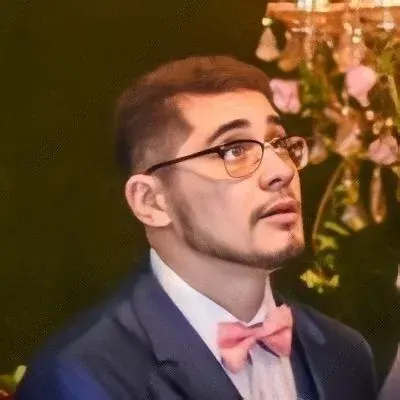
📝 VBA Array Sort Function: Sorting Made Easy in MS Project 2003 🔄
Are you struggling to find a decent sorting implementation for arrays in VBA? Look no further! In this post, we'll dive into the world of VBA array sorting and provide easy solutions to common issues. Whether you prefer a Quicksort or any other sort algorithm, we've got you covered! 💪
🤔 Why Sorting Matters in VBA?
Arrays play a crucial role in VBA programming, allowing you to store and manipulate data efficiently. When it comes to managing data, sorting becomes a must-have feature. It brings order to your array and simplifies data analysis, making it easier to find, compare, and process information.
🧩 Common Issues with VBA Array Sorting
1️⃣ Limited Sorting Options: MS Project 2003 restricts the use of Excel native functions or anything .NET related. This limitation can make finding a suitable sorting solution a bit tricky. But worry not, a few workarounds exist!
2️⃣ Bubble or Merge Sorting: The provided context emphasizes the preference for a Quicksort or alternative sort algorithm over bubble or merge sort. While bubble and merge sort have their merits, they might not be the most efficient for your specific VBA array sorting needs.
🚀 Easy Solutions: Sorting Algorithms for MS Project 2003
1️⃣ Quicksort: Quicksort is a widely used and efficient sorting algorithm that can help you tame your arrays in VBA. While Quicksort is not natively available in VBA, you can implement it on your own. Here's a simplified example of a Quicksort implementation in VBA:
Sub QuickSort(arr, low, high)
Dim i As Integer
Dim j As Integer
Dim pivot As Variant
Dim temp As Variant
i = low
j = high
pivot = arr((low + high) \ 2)
While i <= j
While arr(i) < pivot
i = i + 1
Wend
While arr(j) > pivot
j = j - 1
Wend
If i <= j Then
temp = arr(i)
arr(i) = arr(j)
arr(j) = temp
i = i + 1
j = j - 1
End If
Wend
If low < j Then QuickSort arr, low, j
If i < high Then QuickSort arr, i, high
End Sub
2️⃣ Alternative Sorting Algorithms: If you're not keen on Quicksort, you can explore other sorting algorithms suitable for VBA, such as Insertion Sort, Selection Sort, or Shell Sort. Each algorithm has its own advantages and implementation nuances, so choose the one that best fits your needs.
📣Call-to-Action: Engage with the Community!
We hope this guide helped you find the perfect sorting solution for your VBA arrays in MS Project 2003. Now it's time to take action! Share your experience, ask questions, or provide additional insights in the comments section below. Let's engage with the community and collectively find the best VBA array sorting approaches! 🌟
Keep coding, keep sorting! 👩💻👨💻