TypeScript Objects as Dictionary types as in C#
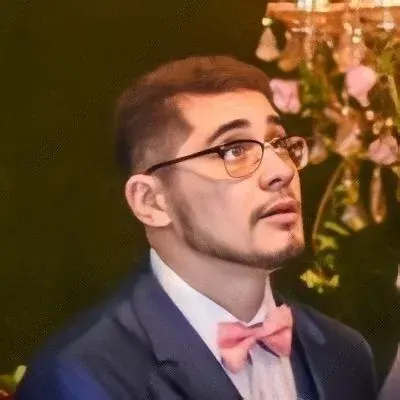
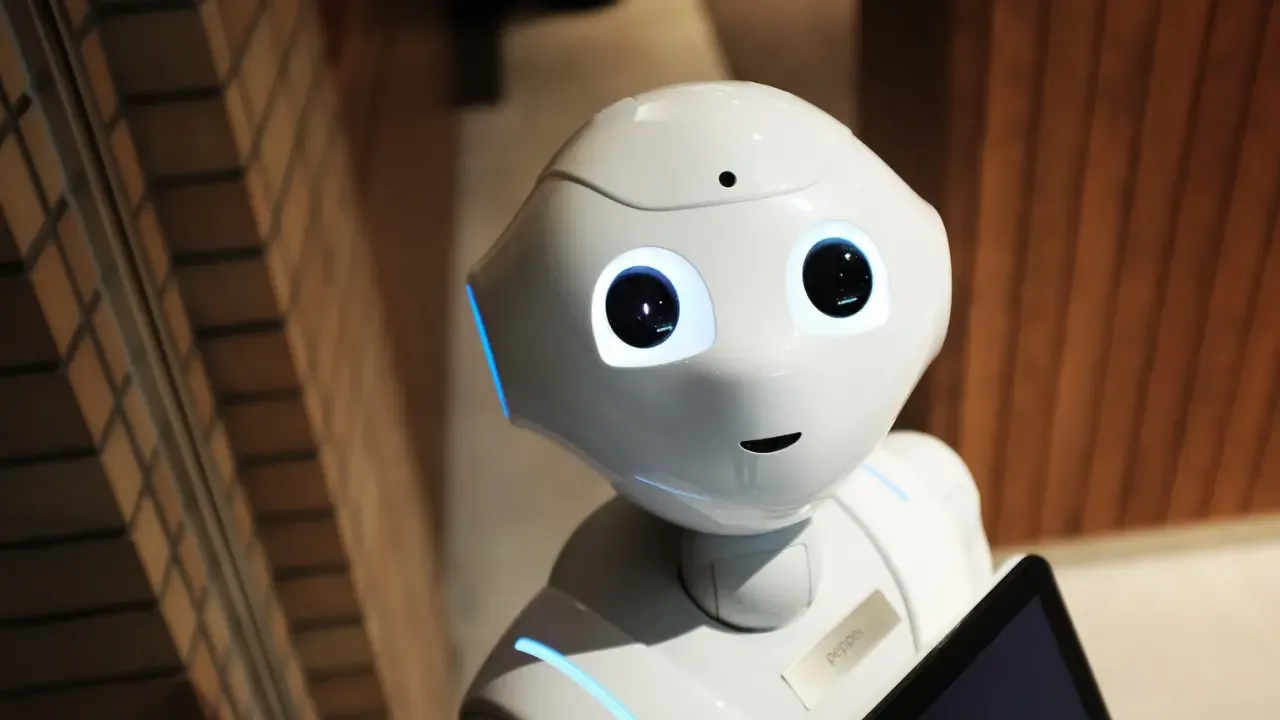
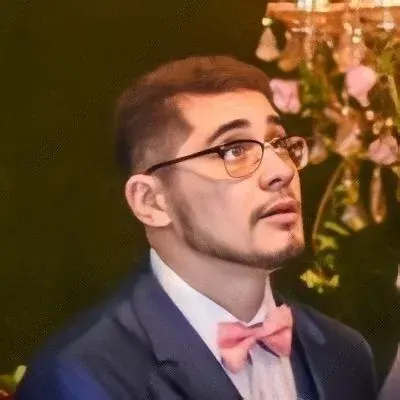
TypeScript Objects as Dictionary Types in C#
š Hey there, tech enthusiasts! Are you struggling with using objects as dictionaries in TypeScript like you would in C#? š¤ Don't worry, we've got you covered! In this blog post, we'll address common issues and provide you with easy solutions to conquer this challenge. So, let's dive in! šŖ
The Scenario
You have some JavaScript code that utilizes objects as dictionaries. For instance, let's take an example where a 'person' object holds various personal details, which are accessed using the email address as the key. So far, so good! Here's how it looks in JavaScript:
var people = {<email>: <'some personal data'>};
// Adding data
people[<email>] = <data>;
// Getting data
var data = people[<email>];
// Deleting data
delete people[<email>];
The TypeScript Dictionary Solution
Now, you might be wondering, "Can I achieve the same behavior in TypeScript, or do I need to use an array?" š¤ Well, the good news is that TypeScript allows us to preserve this functionality without resorting to arrays. š Let's see how we can do it!
Option 1: Index Signature
The first and simplest option is to utilize TypeScript's index signature. This feature allows us to define the types for dynamic keys within an object. Here's an example:
interface People {
[email: string]: string;
}
const people: People = {};
// Adding data
people[<email>] = <data>;
// Getting data
const data: string = people[<email>];
// Deleting data
delete people[<email>];
In this approach, we define an interface called People
, where the keys are of type string
, representing the email addresses, and the values are of type string
, representing the personal data. By utilizing this index signature, we can easily add, retrieve, and delete data from our people
object, just like in the JavaScript code. Sweet! š
Option 2: Using Maps
Another way to achieve dictionary-like behavior in TypeScript is by utilizing the built-in Map
object. The Map
object holds key-value pairs and provides convenient methods for adding, getting, and deleting data. Here's how you can use it:
const people: Map<string, string> = new Map();
// Adding data
people.set(<email>, <data>);
// Getting data
const data: string | undefined = people.get(<email>);
// Deleting data
people.delete(<email>);
In this approach, we create a new Map
object, where the keys are of type string
(email addresses) and the values are of type string
(personal data). The Map
object provides methods like set
, get
, and delete
to manipulate the data within it. Easy peasy! š
The Compelling Call-to-Action
Congratulations! You now know how to use TypeScript objects as dictionary types similar to C#. It's time to put your newfound knowledge into action! š Experiment with both options mentioned above and see which one suits your needs best.
If you found this blog post helpful, why not share it with your fellow developers? Together, we can make coding in TypeScript a breeze! šš» Don't forget to leave us a comment with your thoughts, questions, or any other cool tips you have for using objects as dictionaries in TypeScript.
Happy coding! š
š©āš» Tech Hacks | All rights reserved | www.techhacks.com