Swift Set to Array
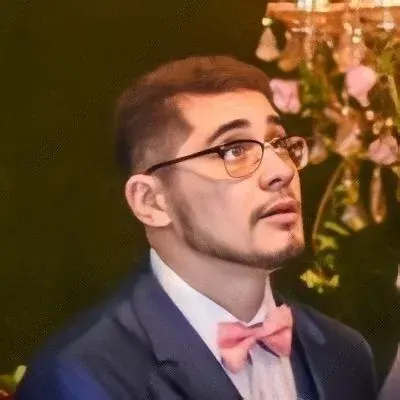
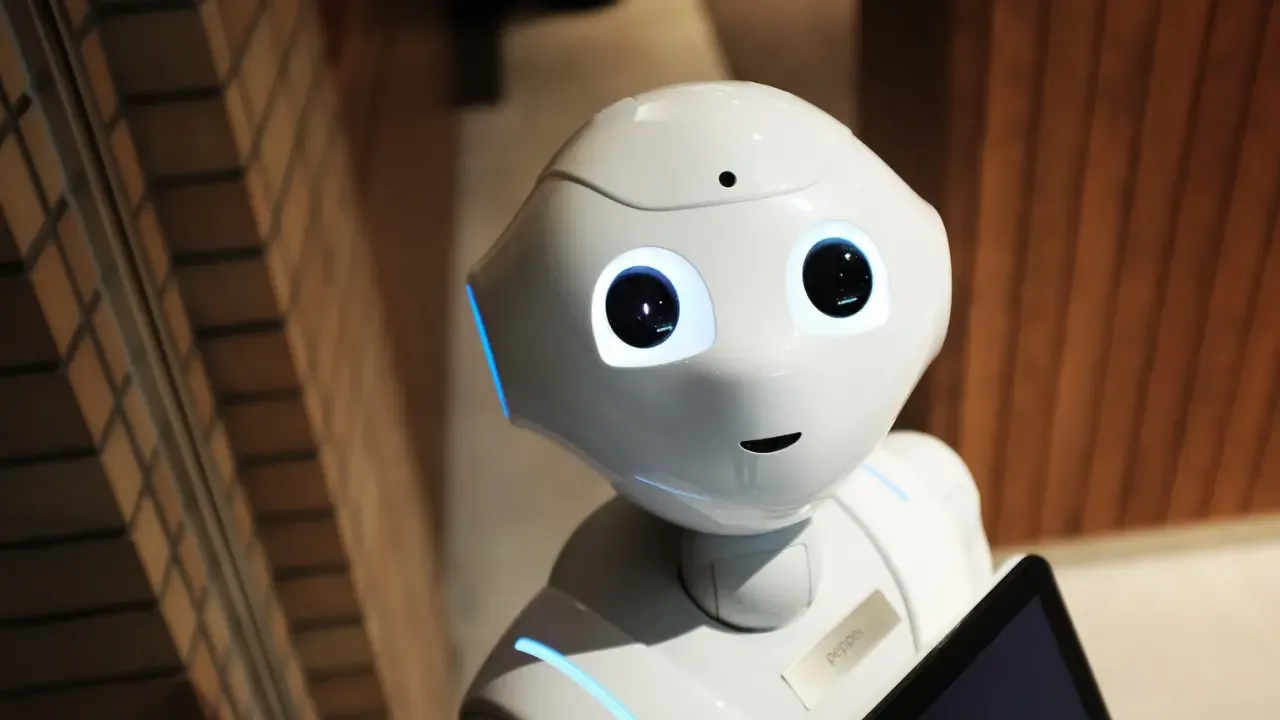
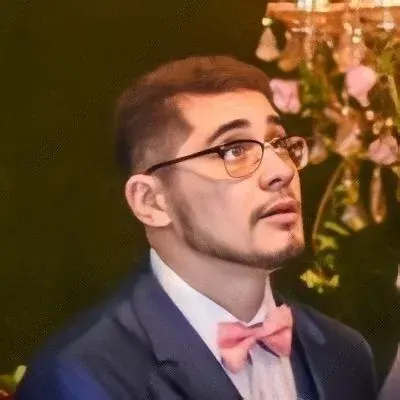
Swift Set to Array: The Ultimate Guide š
š Hello there! Welcome to our tech blog. Today, we will be diving into a common issue that many Swift developers face: converting a Swift Set to an Array. While this process is straightforward with the older NSSet
, things can get a bit trickier when dealing with the new Set
introduced in Swift 1.2. But fear not! We're here to provide you with easy solutions to this problem.
The Challenge:
ā”ļø An NSSet
can be easily converted to an Array
using the allObjects()
method. However, the same method is not available for the new Set
data structure in Swift 1.2. This leaves us scratching our heads when we need to convert a Set
to an Array
.
Solution 1: Using the Array constructor š
⨠Thankfully, Swift provides us with a convenient solution. We can convert a Set
to an Array
using the Array
constructor. Here's an example:
let mySet: Set<String> = ["apple", "banana", "orange"]
let myArray = Array(mySet)
In the above example, we have a Set
called mySet
containing strings. Using the Array
constructor, we simply pass in our Set
as an argument, and voila! We now have an array called myArray
containing the same elements as our original Set
.
Solution 2: Using the sorted() method š
š¢ Sometimes, you might want to convert a Set
to an Array
but also have the elements sorted. Luckily, Swift's sorted()
method comes to the rescue! Let's take a look at an example:
let mySet: Set<Int> = [5, 3, 8, 2, 1]
let mySortedArray = mySet.sorted()
In this example, our Set
called mySet
contains a list of integers. By applying the sorted()
method to our Set
, we can convert and obtain a new array called mySortedArray
with the elements sorted in ascending order.
Solution 3: Using the map() method šŗ
š Last but not least, if you need to apply a transformation to each element in your Set
before converting it to an Array
, the map()
method is your go-to tool. Here's an example to demonstrate its usage:
let mySet: Set<Int> = [1, 2, 3, 4, 5]
let multipliedByTenArray = mySet.map { $0 * 10 }
In this example, our Set
called mySet
contains integers. We want to convert this Set
to an Array
, but also multiply each element by 10. By using the map()
method and specifying the desired transformation as a closure, we can achieve our goal easily.
The Wrap Up and Your Next Move! š
š” Converting a Swift Set
to an Array
doesn't have to be a hassle anymore. We've explored three easy solutions to tackle this problem:
Using the
Array
constructorApplying the
sorted()
methodUtilizing the
map()
method
šŖ Armed with these techniques, you can now confidently convert Set
to Array
in Swift. So go ahead and give them a try!
š If you found this guide helpful, feel free to share it with your fellow developers. And don't hesitate to leave your comments below. We'd love to hear your thoughts and any other solutions you may have!
Happy coding! āØš