Return Index of an Element in an Array Excel VBA
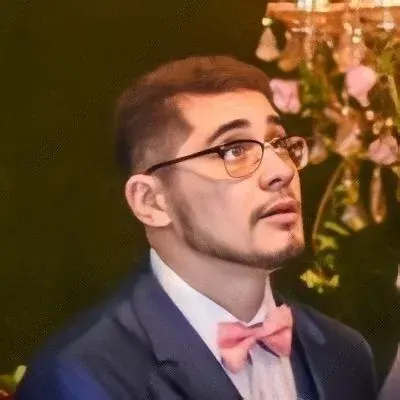
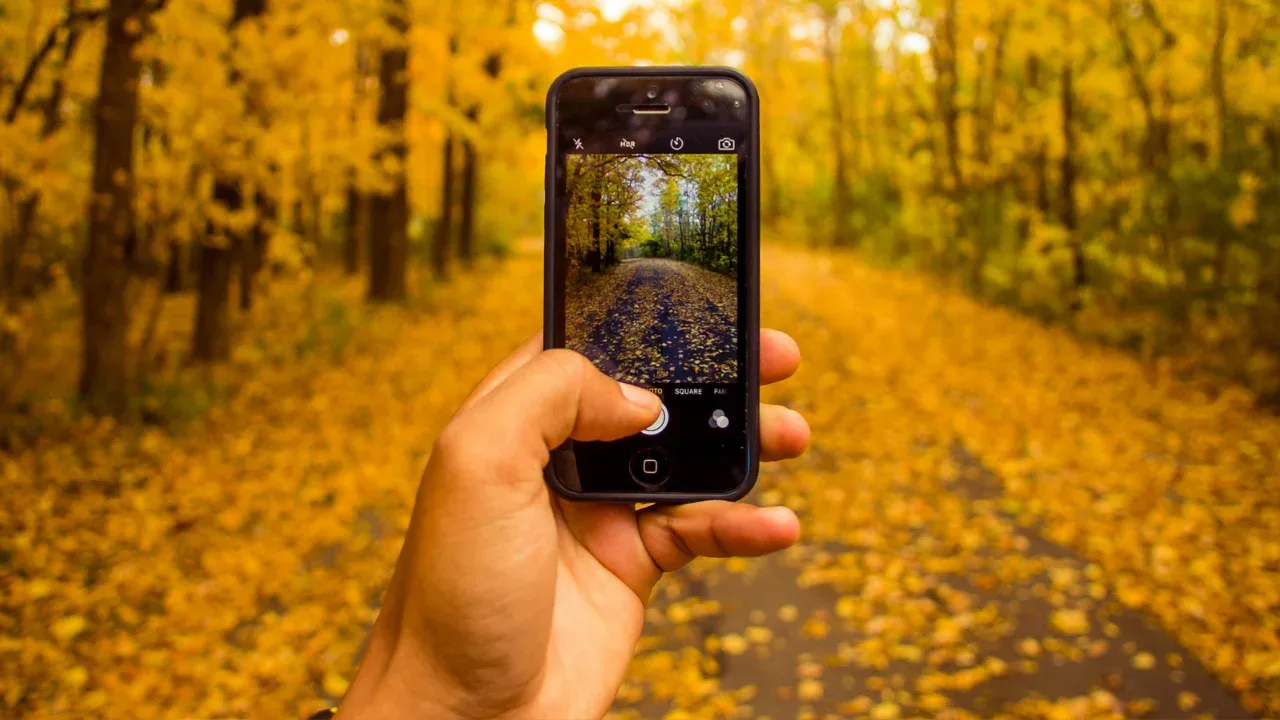
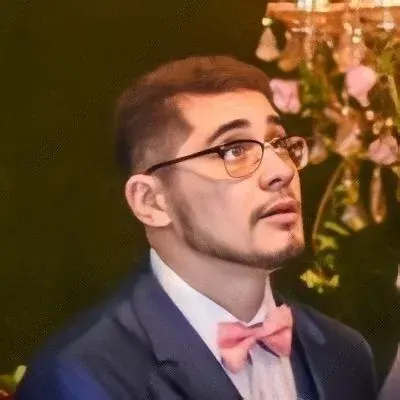
Guide: Return Index of an Element in an Array - Excel VBA
<insert engaging introduction here>
Oh boy! 🤔 We've got a reader who needs help with finding the index of an element in an array using Excel VBA! Look no further, my friend, because we've got you covered! 😎
Understanding the Problem
Our reader has an array called prLst
filled with integers. These integers are not sorted because they represent specific columns in a spreadsheet. The challenge is to find a particular integer in the array and return its index. 📈
The Challenge of Not Using a Range
Our reader mentioned that they couldn't find any resources on how to solve this problem without turning the array into a range on the worksheet. But fear not! It is absolutely possible to achieve this using VBA without the need for complex range conversions. 🙌
The Solution: VBA's Index Function
To return the index of an element in an array using Excel VBA, we can leverage the power of Excel's Index
function. The Index
function is an extremely handy tool that can retrieve the value of a cell in an array, based on its row and column indexes.
However, in our case, we need to find the index of an element based on its value. To do this, we'll need to iterate through the array and compare each element to our desired value.
Here's an example code snippet that demonstrates how to achieve this:
Function FindIndex(arr As Variant, value As Integer) As Variant
Dim i As Long
FindIndex = "Not Found" ' Default value if element is not found
For i = LBound(arr) To UBound(arr)
If arr(i) = value Then
FindIndex = i
Exit Function
End If
Next i
End Function
Let's break down this code:
We define a function called
FindIndex
that takes in two parameters:arr
(the array we want to search in) andvalue
(the element we want to find).We initialize a variable
i
to iterate through the array.We set the default return value of our function to "Not Found" in case the element is not found.
We loop through each element of the array using the
For
loop, starting from the lower bound (LBound
) to the upper bound (UBound
).Inside the loop, we compare each element (
arr(i)
) to the desired value. If we find a match, we setFindIndex
to the current index (i
) and exit the loop usingExit Function
.If the loop finishes without finding a match, the function will return the default value "Not Found".
Putting It Into Action
To use our FindIndex
function, simply call it wherever you need to find the index of an element. Here's an example of how to use it:
Sub ExampleUsage()
Dim prLst() As Integer
Dim desiredValue As Integer
Dim index As Variant
prLst = Array(10, 20, 30, 40, 50)
desiredValue = 30
index = FindIndex(prLst, desiredValue)
If index <> "Not Found" Then
MsgBox "The index of " & desiredValue & " is " & index
Else
MsgBox "Element not found in the array."
End If
End Sub
In this example, we have an array prLst
containing some values. We want to find the index of the element 30
in the array. We call the FindIndex
function, passing in our array and the desired value as arguments. The return value is stored in the index
variable.
Finally, we check if the index
is not equal to "Not Found" and display a message box with the index value. If the index is "Not Found", we display a message indicating that the element was not found in the array.
Conclusion
Don't stress about finding the index of an element in an array using Excel VBA! 📊 With the power of VBA's Index
function and our nifty FindIndex
function, you'll be able to conquer any array search challenge with ease. 🚀
Remember, friends, never be afraid to explore new possibilities with your VBA knowledge. Share this post with your fellow spreadsheet enthusiasts who might be struggling with similar challenges. Let's help each other out! 👯♀️
So go ahead, give it a try, and let us know in the comments below if you found this guide helpful or if you have any other Excel VBA questions. We love hearing from our readers! 💬
Happy coding! ✨