Removing duplicate elements from an array in Swift
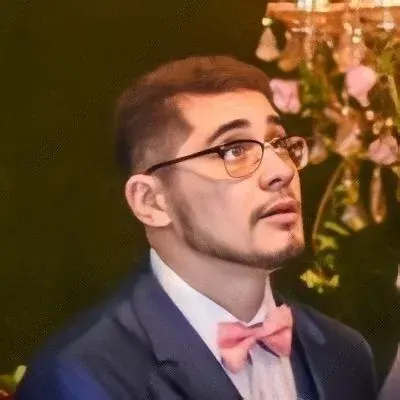
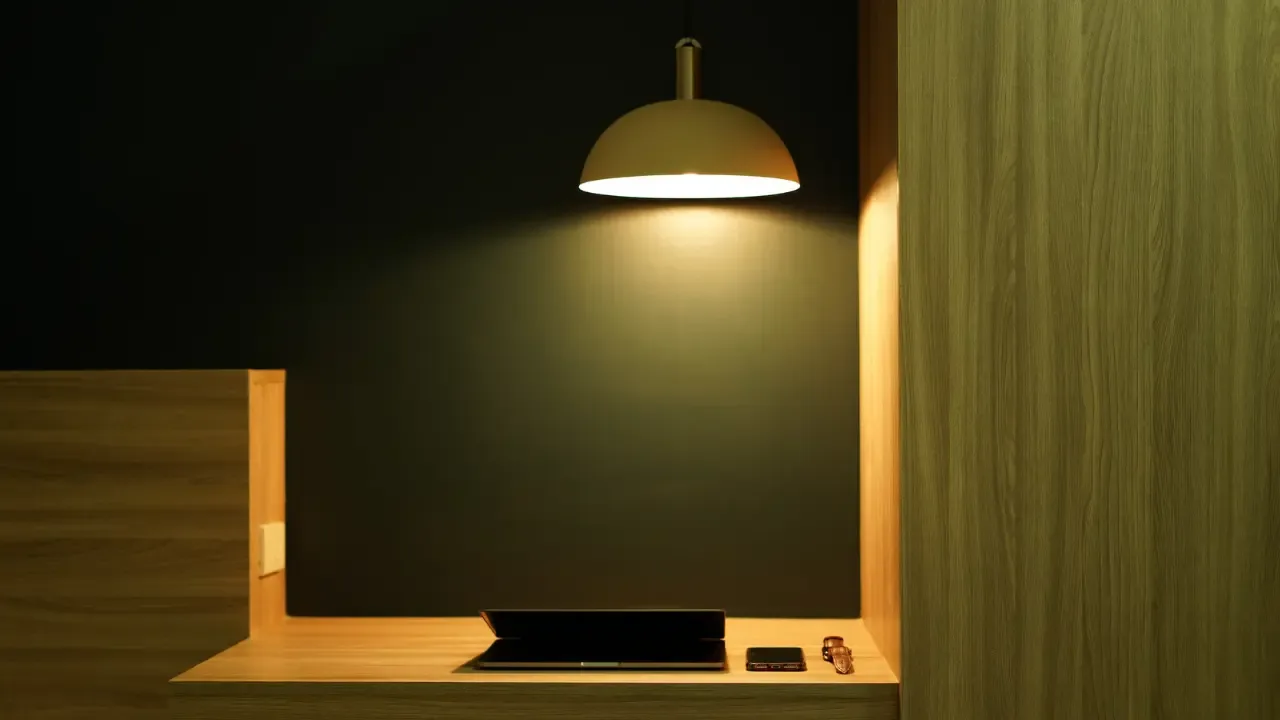
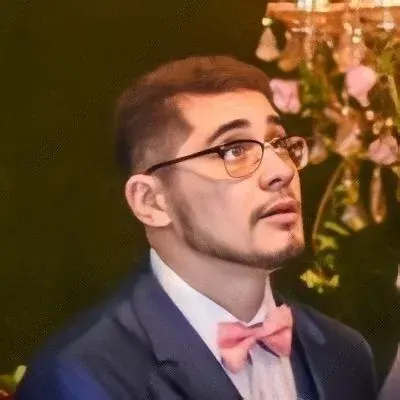
Removing Duplicate Elements from an Array in Swift: A Simple Guide ๐งน
Have you ever found yourself dealing with an array that contains duplicate elements, and you just want to keep one of each? Luckily, Swift provides us with a simple way to achieve this without having to reinvent the wheel. In this blog post, we'll explore how to remove duplicate elements from an array in Swift, addressing common issues and providing easy solutions.
So let's dive right in! ๐โโ๏ธ
The Problem: Duplicate Elements ๐๐
Consider the following array:
let array = [1, 4, 2, 2, 6, 24, 15, 2, 60, 15, 6]
As you can see, there are several duplicate elements in this array. Our goal is to remove the duplicates and create a new array that only contains unique elements. In this case, we want the resulting array to look like this:
let uniqueArray = [1, 4, 2, 6, 24, 15, 60]
The Solution: Using Sets ๐ โจ
Swift provides us with the Set
data structure, which is perfect for removing duplicate elements from an array. Here's a simple solution:
let array = [1, 4, 2, 2, 6, 24, 15, 2, 60, 15, 6]
let uniqueArray = Array(Set(array))
By converting the array to a set using Set(array)
, we automatically remove any duplicate elements. Finally, we convert the set back to an array using Array(...)
to obtain our desired unique array.
That's it! By just a few lines of code ๐, we've successfully removed the duplicate elements.
Dealing with Non-Hashable Elements ๐๐ซ
It's important to note that the elements of the array must be hashable in order to use this approach. Most of the time, Swift's built-in types like Int
, String
, and Bool
are already hashable. However, if you're dealing with custom objects or complex data types, you might need to make them conform to the Hashable
protocol for this solution to work.
Conclusion and Call-to-Action ๐๐ข
Removing duplicate elements from an array in Swift can be achieved easily using the power of sets. By converting the array to a set and then back to an array, we can obtain a unique array without any duplicates.
Now it's your turn! Try out this technique in your own projects and see how it simplifies your code. If you have any questions or other clever solutions for removing duplicate elements in Swift, feel free to share in the comments below. Let's connect and learn from each other! ๐ช๐ฌ
Happy coding! ๐๐ป